filmov
tv
How to Fix TypeError: String Indices Must Be Integers While Parsing JSON in Python
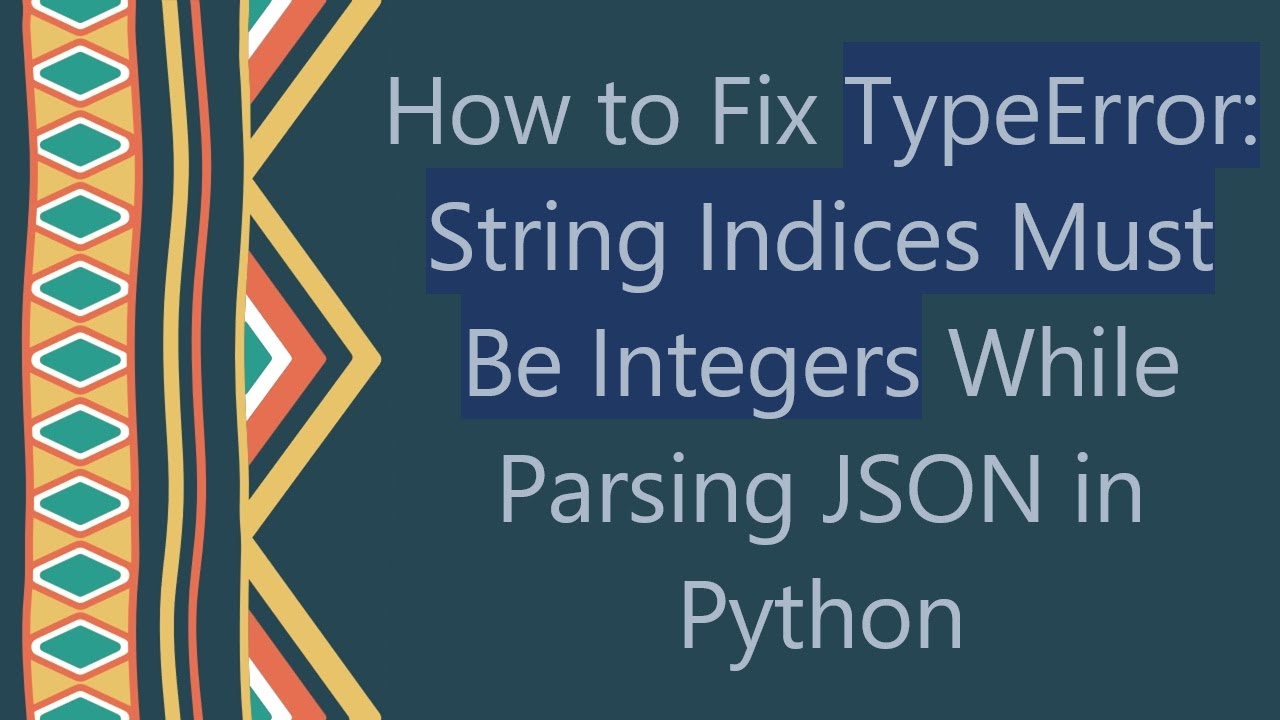
Показать описание
Summary: Learn how to tackle `TypeError: string indices must be integers` during JSON parsing in Python, along with practical tips and code examples to resolve this common issue.
---
How to Fix TypeError: String Indices Must Be Integers While Parsing JSON in Python
As a Python programmer, you might have encountered the perplexing TypeError: string indices must be integers when working with JSON data. This error often occurs when trying to access JSON content improperly. This guide aims to demystify this error and guide you on how to fix it efficiently.
Understanding the Error
The error message TypeError: string indices must be integers is fairly explicit. It means that you are trying to apply string indexing or slicing incorrectly. In Python, strings are indexed using integers, while dictionaries are accessed using keys. Confusing these two can result in the dreaded TypeError.
Scenario: Parsing JSON
JSON (JavaScript Object Notation) is a popular data format used for APIs and configuration files. When parsing JSON data in Python, it is typically loaded into a dictionary. However, improper handling may trigger this type of error.
Example:
Consider a JSON string:
[[See Video to Reveal this Text or Code Snippet]]
Running the above code yields the following error:
[[See Video to Reveal this Text or Code Snippet]]
Why Does This Error Happen?
In the above example, data contains a dictionary: {"name": "Alice", "age": 30}. When we try to access data["name"]["first"], Python expects data["name"] to be another dictionary containing a key "first". However, data["name"] is a string ("Alice"), and strings should be accessed using integer indices.
How to Fix It
Solution: Correct Key Access
To fix this error, ensure that you're accessing the dictionary keys correctly. In the context of the above example, if you want to access the values name and age, do it directly without assuming sub-keys:
[[See Video to Reveal this Text or Code Snippet]]
Additional Tips:
Always Validate JSON Structure: Use debugging and validation to confirm the JSON structure before accessing its contents.
Use .get() Method: The dictionary get method can be used to provide a default value if the key does not exist.
[[See Video to Reveal this Text or Code Snippet]]
nD Array Traversal: For nested JSON structures, ensure you correctly navigate through nested dictionaries and arrays.
Handling Nested JSON Objects:
[[See Video to Reveal this Text or Code Snippet]]
Best Practices:
Use type() or isinstance(): Before accessing elements, confirm their type.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Encountering the TypeError: string indices must be integers error while parsing JSON is common among Python developers. By carefully validating JSON structure and properly accessing its elements, you can avoid this error and ensure smoother data handling in your applications. Follow these best practices to navigate and manipulate JSON data effectively in Python.
---
How to Fix TypeError: String Indices Must Be Integers While Parsing JSON in Python
As a Python programmer, you might have encountered the perplexing TypeError: string indices must be integers when working with JSON data. This error often occurs when trying to access JSON content improperly. This guide aims to demystify this error and guide you on how to fix it efficiently.
Understanding the Error
The error message TypeError: string indices must be integers is fairly explicit. It means that you are trying to apply string indexing or slicing incorrectly. In Python, strings are indexed using integers, while dictionaries are accessed using keys. Confusing these two can result in the dreaded TypeError.
Scenario: Parsing JSON
JSON (JavaScript Object Notation) is a popular data format used for APIs and configuration files. When parsing JSON data in Python, it is typically loaded into a dictionary. However, improper handling may trigger this type of error.
Example:
Consider a JSON string:
[[See Video to Reveal this Text or Code Snippet]]
Running the above code yields the following error:
[[See Video to Reveal this Text or Code Snippet]]
Why Does This Error Happen?
In the above example, data contains a dictionary: {"name": "Alice", "age": 30}. When we try to access data["name"]["first"], Python expects data["name"] to be another dictionary containing a key "first". However, data["name"] is a string ("Alice"), and strings should be accessed using integer indices.
How to Fix It
Solution: Correct Key Access
To fix this error, ensure that you're accessing the dictionary keys correctly. In the context of the above example, if you want to access the values name and age, do it directly without assuming sub-keys:
[[See Video to Reveal this Text or Code Snippet]]
Additional Tips:
Always Validate JSON Structure: Use debugging and validation to confirm the JSON structure before accessing its contents.
Use .get() Method: The dictionary get method can be used to provide a default value if the key does not exist.
[[See Video to Reveal this Text or Code Snippet]]
nD Array Traversal: For nested JSON structures, ensure you correctly navigate through nested dictionaries and arrays.
Handling Nested JSON Objects:
[[See Video to Reveal this Text or Code Snippet]]
Best Practices:
Use type() or isinstance(): Before accessing elements, confirm their type.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Encountering the TypeError: string indices must be integers error while parsing JSON is common among Python developers. By carefully validating JSON structure and properly accessing its elements, you can avoid this error and ensure smoother data handling in your applications. Follow these best practices to navigate and manipulate JSON data effectively in Python.