filmov
tv
How to Properly Declare an Array of Objects in TypeScript
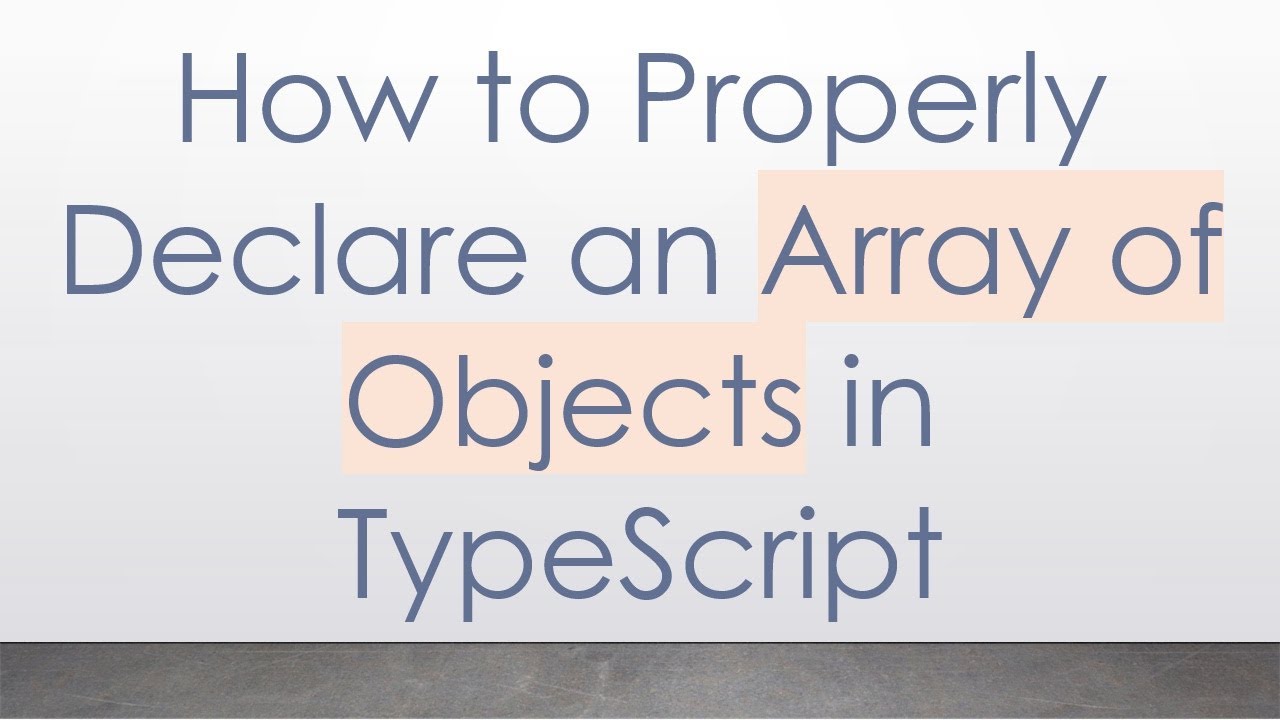
Показать описание
A complete guide to declaring an array of objects in TypeScript, featuring examples and best practices for instantiating interfaces.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to declare an array of objects typescript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Properly Declare an Array of Objects in TypeScript
TypeScript is a powerful tool for developers, allowing them to create robust applications through static typing. However, for beginners, understanding how to declare objects and arrays can be a bit challenging. If you're finding yourself confused about how to declare an array of objects in TypeScript, you're not alone! In this guide, we'll walk through the steps to achieve this correctly, using a practical example.
The Problem
Imagine you have an interface defining a Person:
[[See Video to Reveal this Text or Code Snippet]]
Now, you want to store a predefined array of Person objects. However, you might run into some syntax issues or misunderstandings about how to properly set this up. The initial approach could look something like this:
[[See Video to Reveal this Text or Code Snippet]]
Although it may seem straightforward, this method won't work because interfaces cannot be instantiated directly. Let's dive into the solution!
The Solution
Step 1: Define the Interface Correctly
Instead of trying to initialize an array directly within the interface, you should define the DefinedPeople interface using arrays of Person like this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create a Class or an Object
After defining the interface, you can utilize it in one of two ways: by creating a class that implements the interface or by simply creating an object adhering to that interface.
Option 1: Using a Class
[[See Video to Reveal this Text or Code Snippet]]
Key Points:
Each new instance of SomeClassWithDefinedPeople will come initialized with the values specified in the class definition.
You can access properties like CategoryA[0].name after creating an instance.
Option 2: Creating a Single Object
Alternatively, you can directly create a single object of type DefinedPeople without needing a class:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Declaring an array of objects in TypeScript doesn't have to be complex! By following the proper structure for interfaces and utilizing classes or objects appropriately, you can manage your data in a clean and efficient manner. Remember, the key is to define your infrastructure with clarity and purpose.
Happy coding! If you have any questions or run into issues, don't hesitate to reach out to the programming community for support.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to declare an array of objects typescript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Properly Declare an Array of Objects in TypeScript
TypeScript is a powerful tool for developers, allowing them to create robust applications through static typing. However, for beginners, understanding how to declare objects and arrays can be a bit challenging. If you're finding yourself confused about how to declare an array of objects in TypeScript, you're not alone! In this guide, we'll walk through the steps to achieve this correctly, using a practical example.
The Problem
Imagine you have an interface defining a Person:
[[See Video to Reveal this Text or Code Snippet]]
Now, you want to store a predefined array of Person objects. However, you might run into some syntax issues or misunderstandings about how to properly set this up. The initial approach could look something like this:
[[See Video to Reveal this Text or Code Snippet]]
Although it may seem straightforward, this method won't work because interfaces cannot be instantiated directly. Let's dive into the solution!
The Solution
Step 1: Define the Interface Correctly
Instead of trying to initialize an array directly within the interface, you should define the DefinedPeople interface using arrays of Person like this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Create a Class or an Object
After defining the interface, you can utilize it in one of two ways: by creating a class that implements the interface or by simply creating an object adhering to that interface.
Option 1: Using a Class
[[See Video to Reveal this Text or Code Snippet]]
Key Points:
Each new instance of SomeClassWithDefinedPeople will come initialized with the values specified in the class definition.
You can access properties like CategoryA[0].name after creating an instance.
Option 2: Creating a Single Object
Alternatively, you can directly create a single object of type DefinedPeople without needing a class:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Declaring an array of objects in TypeScript doesn't have to be complex! By following the proper structure for interfaces and utilizing classes or objects appropriately, you can manage your data in a clean and efficient manner. Remember, the key is to define your infrastructure with clarity and purpose.
Happy coding! If you have any questions or run into issues, don't hesitate to reach out to the programming community for support.