filmov
tv
How IEnumerable can kill your performance in C#
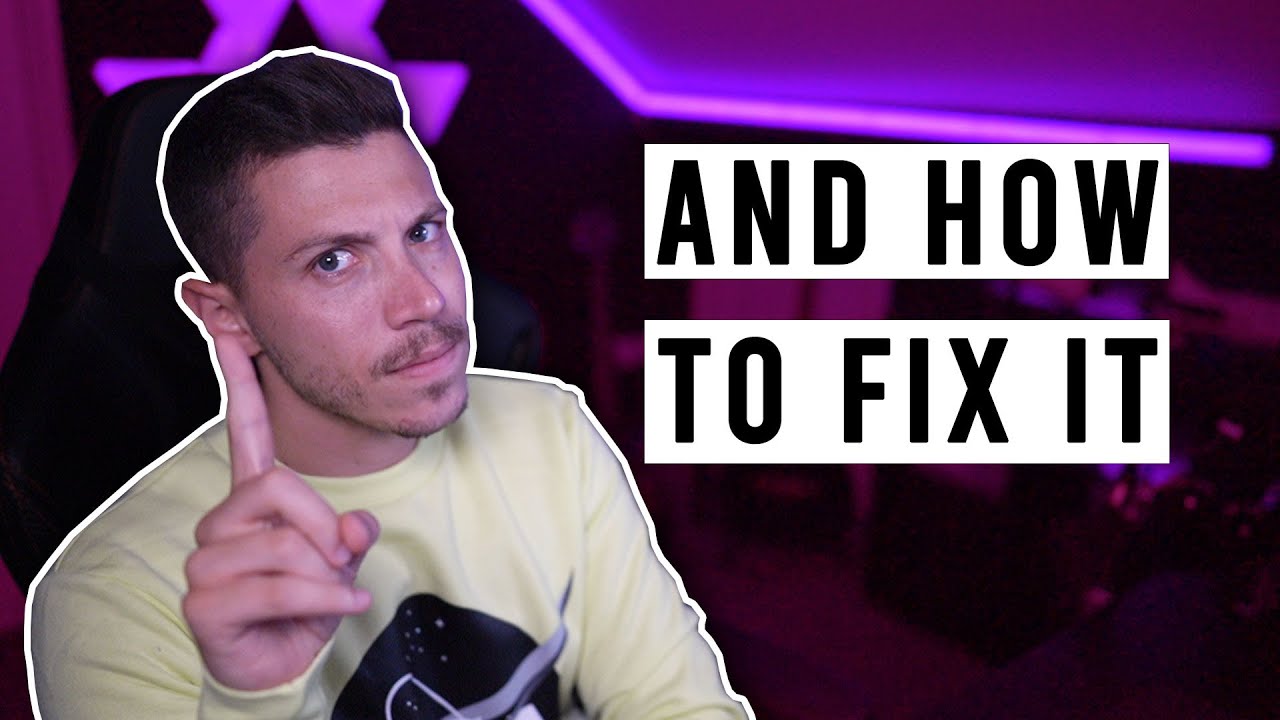
Показать описание
Hello everybody I'm Nick and in this video I will show you how IEnumerable can harm your application's performance. I will explain why it happens, what you can do about it and how to deal with it in future scenarios.
Don't forget to comment, like and subscribe :)
Social Media:
#csharp #dotnet
How IEnumerable can kill your performance in C#
How IEnumerable can make your code more efficient | IEnumerable in C# | C# Collection part 13
Understand your C# queries! IEnumerable & IQueryable in explained
Beginner CRASH COURSE for IEnumerable in .NET C#
C# IEnumerable & IEnumerator
When to use IEnumerable vs IQueryable?
C# IEnumerable deep dive in simple words and practical examples
What is the use of ienumerable in C# ?
How Regex in C# can kill your app
Prefer IEnumerable over collections in method arguments
What is IEnumerable in C#?
IEnumerable 🆚 IEnumerator Interfaces in C#
Implementing IEnumerable
Three Reasons Why you should use IEnumerable in C# .NET #dotnet #csharp #programming
When to use - IEnumerable vs IList vs ICollection?
Stop returning null collections in your code
C# : Is it possible to turn an IEnumerable into an IOrderedEnumerable without using OrderBy?
C# : Count the items from a IEnumerable T without iterating?
C# Yield - Creating Iterators for beginners
C# : Can IEnumerable.Select() skip an item?
Enumerables (IEnumerable, IEnumerator) | C# Programming Tutorials Beginners:
Don’t waste memory with collections | .NET Tips 1
C# : Handling warning for possible multiple enumeration of IEnumerable
C# : When to use IEnumerable vs IObservable?
Комментарии