filmov
tv
Python Class 8 - Python Control Flow Statements Part 2
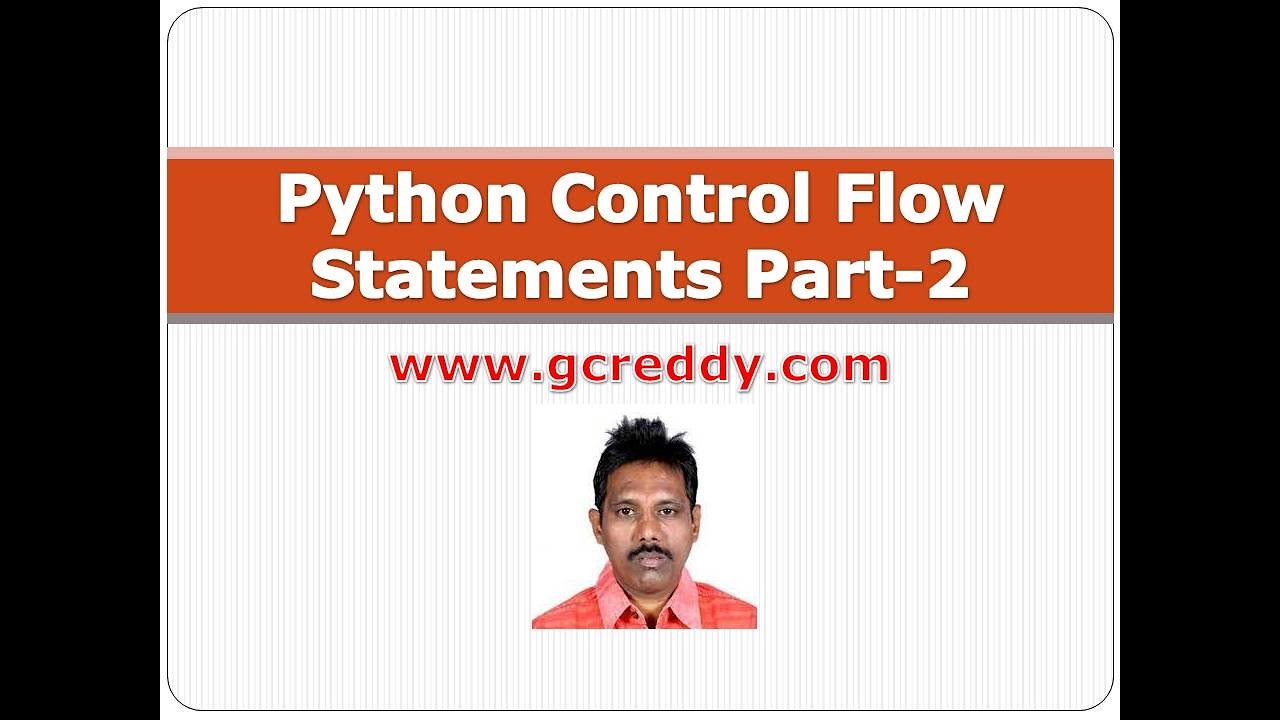
Показать описание
Python Conditional Statements, Decision making in Python programming, Python if statement, Python else statement, and Python elif statement.
We have three types of Control Flow Statements in Python:
1. Conditional Statements
2. Loop Statements
Python Language Loops
Programming languages provide various control structures that allow for more complicated execution paths.
A loop statement allows us to execute a statement or group of statements multiple times.
Python programming language provides two loop structures,
1. while loop
2. for loop to handle loop requirements…
Python Complete Tutorial
Python Videos PlayList
Python Programming Syllabus
Python Programming Quiz
Python Interview Questions for Fresher
--------------------------------------------------
1. Introduction to Python Programming Language
2. Download and Install Python
Python Environment Setup (Using PyCharm IDE)
3. Python Language Syntax
4. Python Keywords and Identifiers
5. Comments in Python
6. Python Variables
7. Python Data Types
8. Python Operators
9. Python Conditional Statements
10. Python Loops
11. Python Branching Statements
12. Python Numbers
13. String Handling in Python
14. Python Data Structures - Lists
15. Python Data Structures - Sets
16. Python Data Structures - Tuples
17. Python Data Structures - Dictionaries
18. Python User Defined Functions
19. Python Built-in Functions
20. Python Modules
21. Python User Input
22. File Handling in Python
23. Python Date and Time
24. Python Exception Handling
25. Python Regular Expressions
26. Python Object Oriented Programming
27. Inheritance in Python
28. Polymorphism in Python
29. Abstraction in Python
--------------------------------------------------
Комментарии