filmov
tv
Find equilibrium point in an array
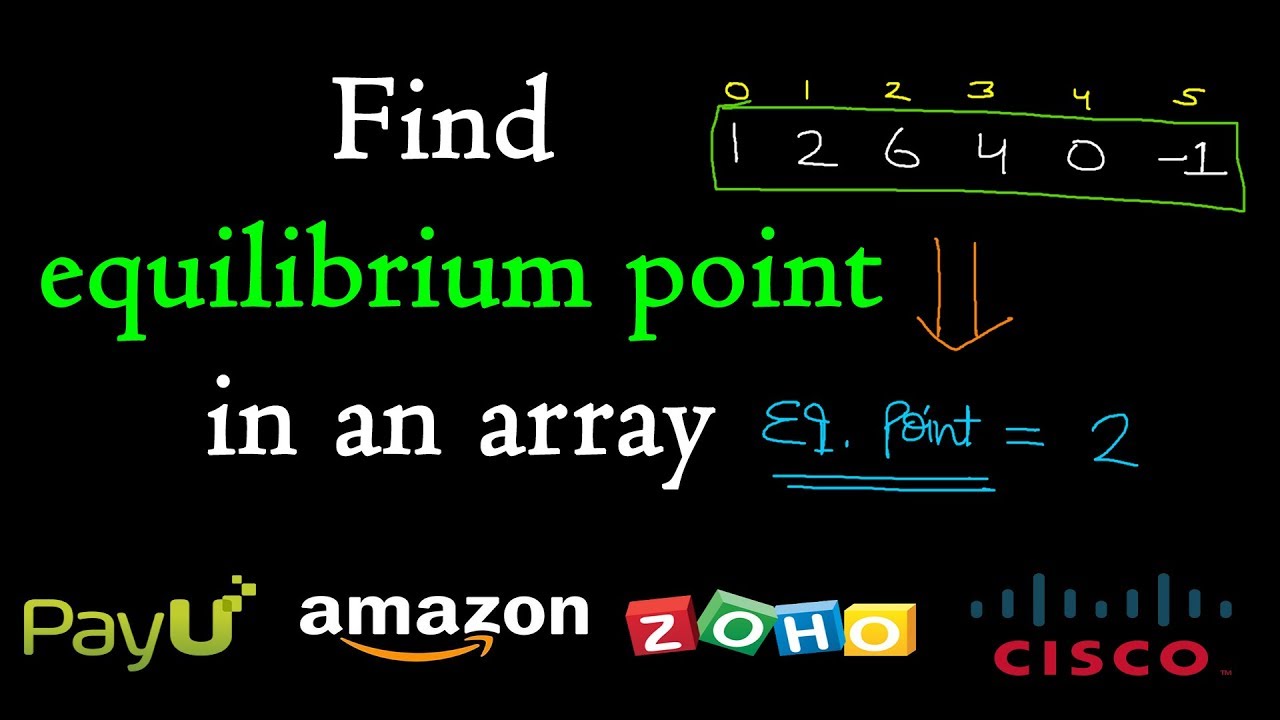
Показать описание
This video explains how to find equilibrium point in an array. 2 methods are explained which involves a preprocessing efficient method which works in just O(N) time. This program is frequently asked in interviews in software companies. CODE LINK is present in description below. If you find any difficulty or have any query then do COMMENT below. PLEASE help our channel by SUBSCRIBING and LIKE our video if you found it helpful...CYA :)
Find equilibrium point in an array
Equilibrium Points for Nonlinear Differential Equations
How to Calculate Market Equilibrium | (NO GRAPHING) | Think Econ
Autonomous Equations, Equilibrium Solutions, and Stability
Differential Equations - Non-Linear Systems - Finding Equilibrium Solutions
25.2 Stable and Unstable Equilibrium Points
How to Calculate Equilibrium Price and Quantity (Demand and Supply)
1. Equilibrium of a Point
Nondimensionalization, biochemical reaction networks
Market equilibrium | Supply, demand, and market equilibrium | Microeconomics | Khan Academy
Finding equilibrium price and quantity using linear demand and supply equations
Equilibrium Solutions and Stability of Differential Equations (Differential Equations 36)
Equilibrium Points
Equilibrium Point Analysis via Linearization
Equilibrium Point
1.7 Equilibrium Market Schedule & Graph
Determine Supply and Demand Functions and Equilibrium Point (Linear)
D-330 Equilibrium Point | 2 ways | Prefix Sum|JAVA|C++| GFG Problem of the Day |English|23 Sep
#114 GFG POTD Equilibrium Point GeeksForGeeks Problem of the Day | PlacementsReady
Nullclines and Dynamics of Systems
Types of Equilibrium points
Linear Systems ODEs Nullclines
Array - 13: Find Equilibrium point where elements before & after it has equal sum
supply demand in equilibrium
Комментарии