filmov
tv
Convert array pointer to list in python ctypes
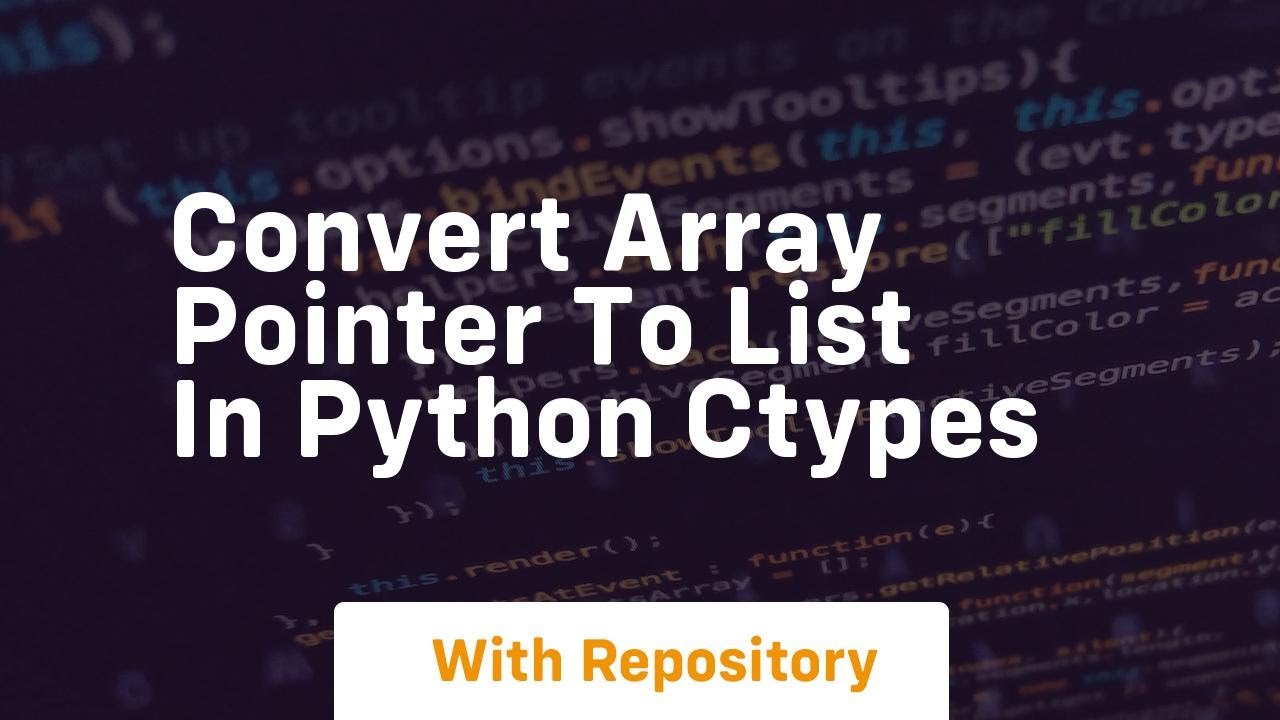
Показать описание
in python, the `ctypes` library allows you to work with c-style data types and structures, including arrays and pointers. if you have an array represented as a pointer and you want to convert it into a python list, you can do so with `ctypes` by following these steps:
### step-by-step tutorial
1. **import `ctypes`:** begin by importing the `ctypes` module which provides the necessary tools to work with c data types and structures.
2. **define the c array type:** use `ctypes` to define the type of the array you are dealing with. for example, if it’s an array of integers, you can use `ctypes.c_int`.
3. **create the pointer:** create a pointer to the array using `ctypes` functions.
4. **convert the pointer to a list:** use the `from_buffer` or `from_address` method to get the array elements and convert them to a python list.
### code example
here's an example that demonstrates how to convert a c-style array pointer to a python list using `ctypes`.
### explanation of the code
1. **define the c array type:**
- `arraytype = ctypes.c_int * 5` defines a c-style array that can hold 5 integers.
2. **create an instance of the array:**
- `c_array = arraytype(1, 2, 3, 4, 5)` initializes the array with given values.
3. **create a pointer to the array:**
4. **convert the pointer to a python list:**
- `length = len(c_array)` gets the length of the array.
- the list comprehension `[array_pointer[i] for i in range(length)]` iterates through the pointer and collects the values into a python list.
### output
when you run the above code, the output will be:
### conclusion
using `ctypes` in python, you can easily convert c-style array pointers to python lists. this is particularly useful when interfacing with c libraries or when working with low-level data structures. remember to manage memory carefully when working with pointers, ...
#python array append
#python array slice
#python array indexing
#python array pop
#python array methods
python array append
python array slice
python array indexing
python array pop
python array methods
python array vs list
python array size
python array
python array to string
python array length
python convert float to int
python convert set to list
python convert bytes to string
python convert list to string
python convert string to json
python convert dict to json
python convert string to float
python convert to string
### step-by-step tutorial
1. **import `ctypes`:** begin by importing the `ctypes` module which provides the necessary tools to work with c data types and structures.
2. **define the c array type:** use `ctypes` to define the type of the array you are dealing with. for example, if it’s an array of integers, you can use `ctypes.c_int`.
3. **create the pointer:** create a pointer to the array using `ctypes` functions.
4. **convert the pointer to a list:** use the `from_buffer` or `from_address` method to get the array elements and convert them to a python list.
### code example
here's an example that demonstrates how to convert a c-style array pointer to a python list using `ctypes`.
### explanation of the code
1. **define the c array type:**
- `arraytype = ctypes.c_int * 5` defines a c-style array that can hold 5 integers.
2. **create an instance of the array:**
- `c_array = arraytype(1, 2, 3, 4, 5)` initializes the array with given values.
3. **create a pointer to the array:**
4. **convert the pointer to a python list:**
- `length = len(c_array)` gets the length of the array.
- the list comprehension `[array_pointer[i] for i in range(length)]` iterates through the pointer and collects the values into a python list.
### output
when you run the above code, the output will be:
### conclusion
using `ctypes` in python, you can easily convert c-style array pointers to python lists. this is particularly useful when interfacing with c libraries or when working with low-level data structures. remember to manage memory carefully when working with pointers, ...
#python array append
#python array slice
#python array indexing
#python array pop
#python array methods
python array append
python array slice
python array indexing
python array pop
python array methods
python array vs list
python array size
python array
python array to string
python array length
python convert float to int
python convert set to list
python convert bytes to string
python convert list to string
python convert string to json
python convert dict to json
python convert string to float
python convert to string