filmov
tv
Resolving the EOFError: EOF when reading a line Issue in Python Functions
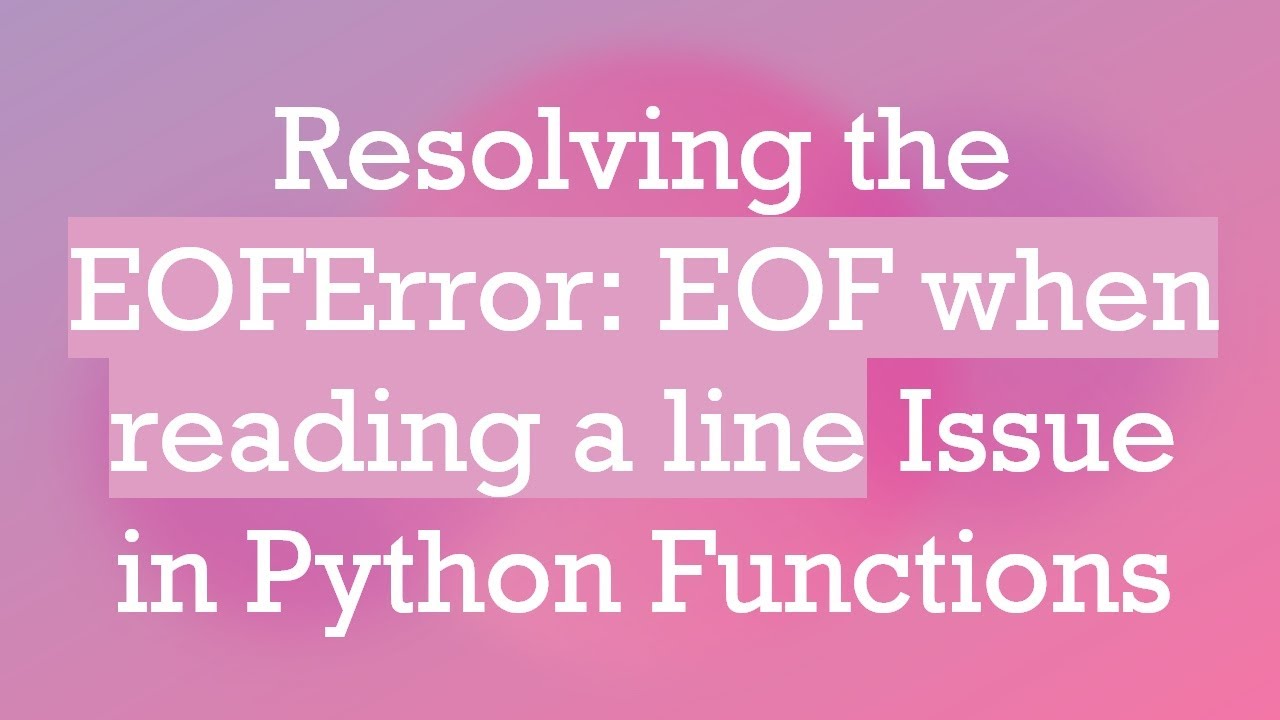
Показать описание
Learn how to fix the `EOFError` in your Python code when reading input and improve your function's design for better performance and usability.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: EOFError: EOF when reading a line error in my function
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving the EOFError: EOF when reading a line Issue in Python Functions
When working with Python, you might encounter various errors, and one common error developers face is the infamous EOFError: EOF when reading a line. This particular error is usually generated when your code tries to read input from the user, but there is no input available. Let's discuss a specific scenario where this error can occur and how to effectively solve it.
The Problem Explained
In the provided code snippet, the user has created a function to calculate the sum and product of a list of numbers inputted by the user. However, when they try to execute the function, they encounter an EOFError due to how the input() function is being used. Here's the problematic part of the code:
[[See Video to Reveal this Text or Code Snippet]]
What Causes the Error?
The first input() call collects user input successfully. However, when the second input() call is executed, there is no more input available, leading to the EOFError being raised.
This scenario often happens in automated testing or cases where input is expected only once.
The Solution
Simplifying Input Handling
To resolve this issue, the recommended approach is to call your sum_and_product function only once and store its results in variables. This way, you can then utilize those results to print the sum and product without making multiple calls to input(). Here's how you can implement this:
Read Input Once: Call the input() function once at the beginning and store the returned values.
Store Results: Assign the results from your function to separate variables.
Display Output: Print the results using those variables.
Updated Code Example
Here's the revised version of the code that follows the suggested fix:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Single Input Call: Always strive to minimize the number of input() calls, especially in a loop or when expecting multiple outputs based on user input.
Use Variables Wisely: Utilizing variables to manage and manipulate data helps keep the code clean and reduces the risk of common errors.
Format Outputs: Ensure your printed outputs are clear and formatted, as demonstrated with the use of :>8.2f to align numbers properly.
By following these guidelines, you can prevent the EOFError from occurring and enhance the functionality of your Python programs. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: EOFError: EOF when reading a line error in my function
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving the EOFError: EOF when reading a line Issue in Python Functions
When working with Python, you might encounter various errors, and one common error developers face is the infamous EOFError: EOF when reading a line. This particular error is usually generated when your code tries to read input from the user, but there is no input available. Let's discuss a specific scenario where this error can occur and how to effectively solve it.
The Problem Explained
In the provided code snippet, the user has created a function to calculate the sum and product of a list of numbers inputted by the user. However, when they try to execute the function, they encounter an EOFError due to how the input() function is being used. Here's the problematic part of the code:
[[See Video to Reveal this Text or Code Snippet]]
What Causes the Error?
The first input() call collects user input successfully. However, when the second input() call is executed, there is no more input available, leading to the EOFError being raised.
This scenario often happens in automated testing or cases where input is expected only once.
The Solution
Simplifying Input Handling
To resolve this issue, the recommended approach is to call your sum_and_product function only once and store its results in variables. This way, you can then utilize those results to print the sum and product without making multiple calls to input(). Here's how you can implement this:
Read Input Once: Call the input() function once at the beginning and store the returned values.
Store Results: Assign the results from your function to separate variables.
Display Output: Print the results using those variables.
Updated Code Example
Here's the revised version of the code that follows the suggested fix:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways
Single Input Call: Always strive to minimize the number of input() calls, especially in a loop or when expecting multiple outputs based on user input.
Use Variables Wisely: Utilizing variables to manage and manipulate data helps keep the code clean and reduces the risk of common errors.
Format Outputs: Ensure your printed outputs are clear and formatted, as demonstrated with the use of :>8.2f to align numbers properly.
By following these guidelines, you can prevent the EOFError from occurring and enhance the functionality of your Python programs. Happy coding!