filmov
tv
Converting a Map to a List of Custom DTO Objects Using Java 8 Streams
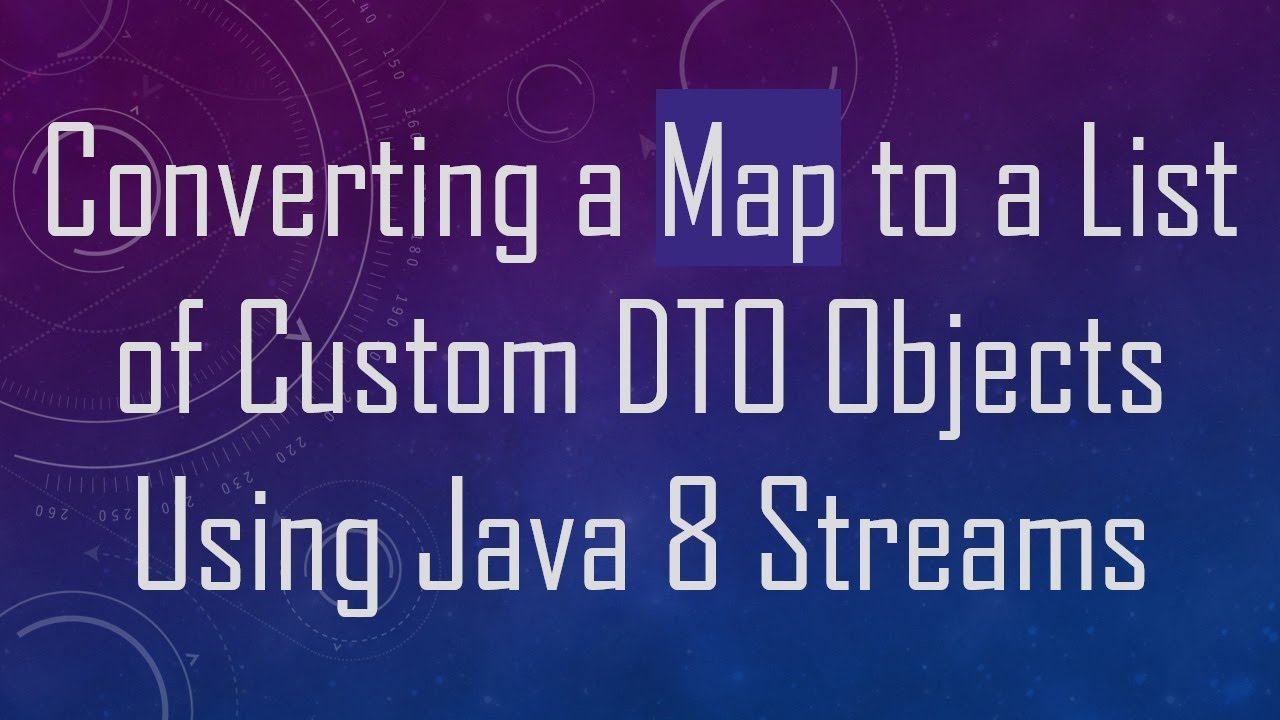
Показать описание
Learn how to easily convert a `Map` of student lists into a list of `Room` DTO objects with Java 8 streams. This guide provides a clear and concise explanation for programmers looking to streamline their data transformations.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Convert map to specific dto object using stream JAVA 8
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a Map to a List of Custom DTO Objects Using Java 8 Streams
Java 8 introduced powerful stream processing capabilities that allow developers to perform complex data manipulation in a clean and efficient manner. One common task is converting a data structure like a Map into a list of custom objects, or DTOs (Data Transfer Objects). In this guide, we'll walk through a practical example where we convert a Map<String, List<Student>> into a list of Room objects.
The Problem: Mapping Data Structures
Suppose we have a Map<String, List<Student>> that looks as follows:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to transform this Map into a list of Room objects. Each Room should be instantiated with the room number (the key of the map) and the corresponding list of students (the value). The desired output is a list containing instances of Room, such as:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Using Java 8 Streams
To achieve this transformation using Java streams, we need to follow these steps:
Stream the Entries of the Map: Access key-value pairs within the map.
Map Each Entry to a Room Object: For each entry, we will create a new Room instance using the key and value.
Here's a code example that illustrates how to implement this solution:
Step-by-Step Implementation
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code:
This initiates a stream from the entry set of the map, allowing us to work with both keys and values.
The map function takes each entry e and creates a new Room object using the entry's key (room number) and value (list of students).
This collects all the Room objects produced in the mapping process and gathers them into a list.
Conclusion
Using Java 8 streams to convert a Map<String, List<Student>> into a list of Room DTOs is both straightforward and efficient. By following the steps outlined in this post, you can enhance your data processing capabilities and write cleaner, more maintainable code.
This practice not only adheres to the principles of functional programming, as encouraged by Java 8's stream API, but also simplifies the handling of complex data structures. So the next time you're faced with a similar data conversion task, remember this concise yet powerful approach!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Convert map to specific dto object using stream JAVA 8
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a Map to a List of Custom DTO Objects Using Java 8 Streams
Java 8 introduced powerful stream processing capabilities that allow developers to perform complex data manipulation in a clean and efficient manner. One common task is converting a data structure like a Map into a list of custom objects, or DTOs (Data Transfer Objects). In this guide, we'll walk through a practical example where we convert a Map<String, List<Student>> into a list of Room objects.
The Problem: Mapping Data Structures
Suppose we have a Map<String, List<Student>> that looks as follows:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to transform this Map into a list of Room objects. Each Room should be instantiated with the room number (the key of the map) and the corresponding list of students (the value). The desired output is a list containing instances of Room, such as:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Using Java 8 Streams
To achieve this transformation using Java streams, we need to follow these steps:
Stream the Entries of the Map: Access key-value pairs within the map.
Map Each Entry to a Room Object: For each entry, we will create a new Room instance using the key and value.
Here's a code example that illustrates how to implement this solution:
Step-by-Step Implementation
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code:
This initiates a stream from the entry set of the map, allowing us to work with both keys and values.
The map function takes each entry e and creates a new Room object using the entry's key (room number) and value (list of students).
This collects all the Room objects produced in the mapping process and gathers them into a list.
Conclusion
Using Java 8 streams to convert a Map<String, List<Student>> into a list of Room DTOs is both straightforward and efficient. By following the steps outlined in this post, you can enhance your data processing capabilities and write cleaner, more maintainable code.
This practice not only adheres to the principles of functional programming, as encouraged by Java 8's stream API, but also simplifies the handling of complex data structures. So the next time you're faced with a similar data conversion task, remember this concise yet powerful approach!