filmov
tv
Queue and FIFO/FCFS explained in 10 minutes + a bonus task 📣📣 (Data Structures course)
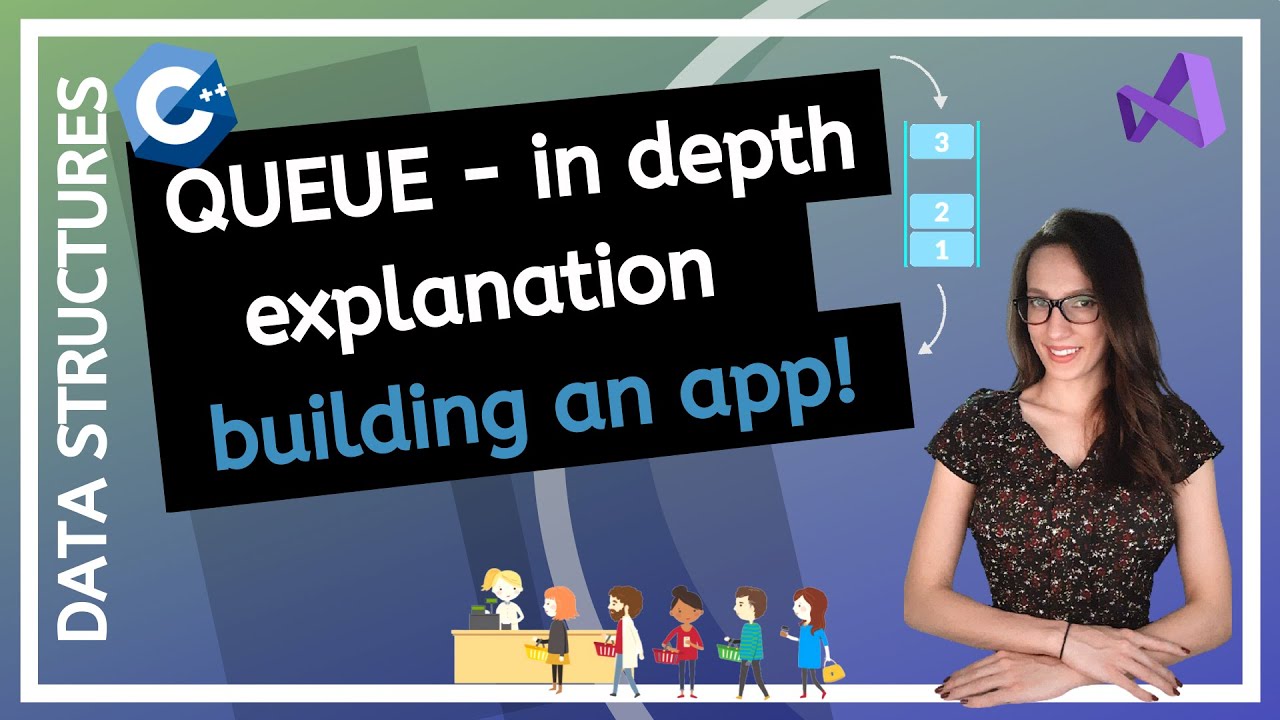
Показать описание
📚 Learn how to solve problems and build projects with these Free E-Books ⬇️
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
I use it to enhance the performance, features, and support for C, C#, and C++ development in Visual Studio.
It is a powerful, secure text editor designed specifically for programmers.
A queue is a FIFO Data structure (First in-First out). It is also known as FCFS (First come-First served)
A real-life example of a queue is a queue in a bank - the person who is at the front of the queue will be served first, and the new people who come into the bank will stand at the end of the queue and they will be served last. this means that we add new elements at the end of the queue and we remove them from the front.
We use queues in programming whenever we need things to happen in the exact order they were called, but the computer cannot keep up with the speed and execute those things fast enough.
In this situation, we put those things in a queue and we process/remove them from the front and add new things at the end of the queue.
The most common example of how queues are used in programming is the way a printer works - you can send multiple pages to print, but because printer takes a few seconds to print each page, all pages will be in a queue waiting for their turn to be printed, and they will be printed in the exact order they were sent to print.
I'm also going to share some examples of how I use queues in my programming work, and I'll explain the code of STL Queue and teach you about the most important functions used with queues:
push - add an element at the end
pop - remove an element from the front
size - tells you the size of the queue
front - first element of the queue
back - the last element of the queue
empty - tells you if the queue is empty
However, please don't feel obligated to do so. I appreciate every one of you, and I will continue to share valuable content with you regardless of whether you choose to support me in this way. Thank you for being part of the Code Beauty community! ❤️😇
Contents:
00:00 - What will you learn in this video
02:02 - What is a Queue, What is FIFO/FCFS
02:50 - When to use a queue in programming
05:40 - C++ code for STL Queue explained
08:39 - Solve this programming task and get a shoutout in my next video 📣
Tag me on you Instagram stories:
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
I use it to enhance the performance, features, and support for C, C#, and C++ development in Visual Studio.
It is a powerful, secure text editor designed specifically for programmers.
A queue is a FIFO Data structure (First in-First out). It is also known as FCFS (First come-First served)
A real-life example of a queue is a queue in a bank - the person who is at the front of the queue will be served first, and the new people who come into the bank will stand at the end of the queue and they will be served last. this means that we add new elements at the end of the queue and we remove them from the front.
We use queues in programming whenever we need things to happen in the exact order they were called, but the computer cannot keep up with the speed and execute those things fast enough.
In this situation, we put those things in a queue and we process/remove them from the front and add new things at the end of the queue.
The most common example of how queues are used in programming is the way a printer works - you can send multiple pages to print, but because printer takes a few seconds to print each page, all pages will be in a queue waiting for their turn to be printed, and they will be printed in the exact order they were sent to print.
I'm also going to share some examples of how I use queues in my programming work, and I'll explain the code of STL Queue and teach you about the most important functions used with queues:
push - add an element at the end
pop - remove an element from the front
size - tells you the size of the queue
front - first element of the queue
back - the last element of the queue
empty - tells you if the queue is empty
However, please don't feel obligated to do so. I appreciate every one of you, and I will continue to share valuable content with you regardless of whether you choose to support me in this way. Thank you for being part of the Code Beauty community! ❤️😇
Contents:
00:00 - What will you learn in this video
02:02 - What is a Queue, What is FIFO/FCFS
02:50 - When to use a queue in programming
05:40 - C++ code for STL Queue explained
08:39 - Solve this programming task and get a shoutout in my next video 📣
Tag me on you Instagram stories:
Комментарии