filmov
tv
Convert binary to decimal | C Programming Example
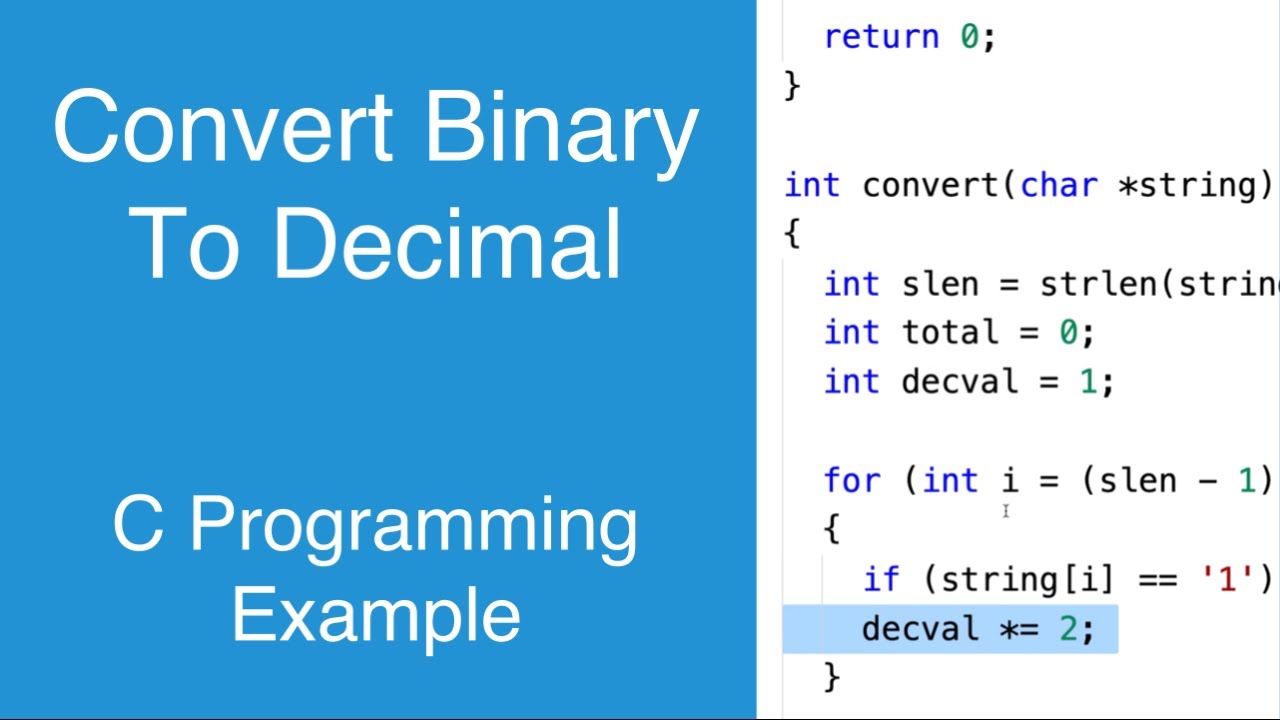
Показать описание
How To Convert Binary To Decimal - Computer Science
How to Convert Binary to Decimal
How to convert binary to decimal
How to Convert Binary to Decimal
Binary to Decimal Conversion | PingPoint
Number Systems Introduction - Decimal, Binary, Octal & Hexadecimal
Decimal to Binary and Binary to Decimal Conversion
How to convert BINARY to DECIMAL
2-hour Codesys tutorial on PLC programming and Industrial Automation Basics
How to convert a binary number to decimal number (English)
Special Programs in C − Binary to Decimal Conversion
Binary Number System: Counting in Binary Number System | Binary to Decimal Conversion
How To Convert Decimal to Binary
Binary to decimal base Conversion using calculator
How to Convert Binary to Decimal Numbers in Just a Minute!|
Binary to Decimal Using Excel!
Binary - The SIMPLEST explanation of Counting and Converting Binary numbers
Binary to Decimal and Decimal to Binary Conversion | Learn Coding
Binary to decimal short trick
Convert Binary to Decimal by calculator FX - 100 MS #conversion #FX - 100 MS
Binary to Decimal (IPv.4 conversion)
Binary to Decimal in Urdu, Binary to Decimal Conversion in Urdu
How to convert BINARY to DECIMAL, and DECIMAL to BINARY
How to convert a binary number into a decimal number in just a second| (1011)2 into Decimal
Комментарии