filmov
tv
Plus One - Leetcode 66 - Python
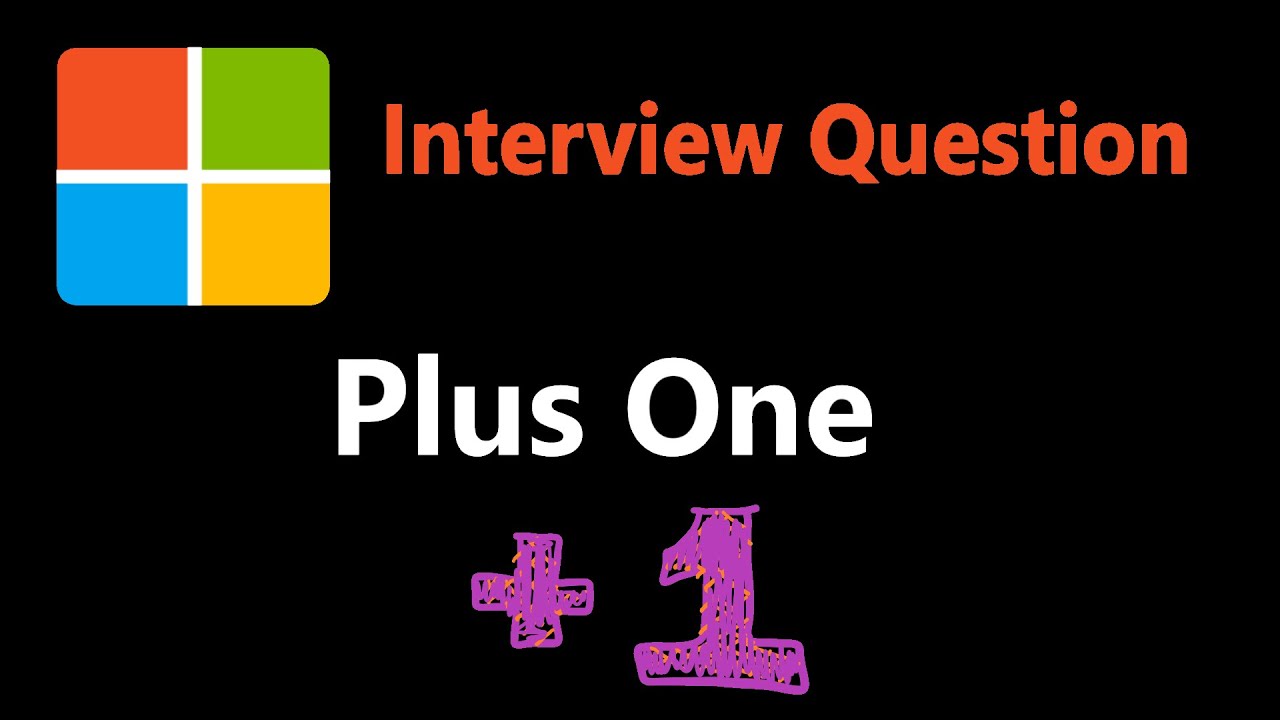
Показать описание
0:00 - Read the problem
1:40 - Drawing Explanation
4:50 - Coding Explanation
leetcode 66
#sorted #array #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Plus One - Leetcode 66 - Python
Plus one | LeetCode problem 66
LeetCode 66. Plus One Solution Explained - Java
Plus One | LeetCode 66 | C++, Java, Python
Solving LeetCode 66 in JavaScript (Plus One)
LeetCode 66: Plus One - Interview Prep Ep 51
Plus One - LeetCode 66 (Easy logic Explanation!)
LeetCode #66 - PlusOne
Lesson 12, №66, Plus One
Leetcode 66. Plus One
66 Plus One LeetCode (Google Interview Question) JavaScript
66. Plus One | LEETCODE EASY | CODE EXPLAINER
Plus one LeetCode Solution Java ( leetcode 66 )
Microsoft Question | LeetCode 66. Plus One | English | code io
PLUS ONE | PYTHON | LEETCODE # 66 (FACEBOOK & GOOGLE INTERVIEW QUESTION)
plus one | plus one leetcode | leetcode 66 | iterative & recursive
LeetCode #66: Plus One | Beginner Level
Plus One | Day 6 | Addition Optimization [ July LeetCoding Challenge ] [ Leetcode #66 ] [ 2020 ]
LeetCode 66: Plus One
Plus One | LeetCode 66 | Add One to Number Represented as Array | Programming Tutorials
Leetcode - Plus One (Python)
Python Programming Practice: LeetCode #66 -- Plus One
Plus One - LeetCode 66 - Solution Explained
Plus One - LeetCode #66 - Python, JavaScript, Java, C++
Комментарии