filmov
tv
Understanding the enum class Definition Process in Python: Why __call__ is Used Instead of __new__
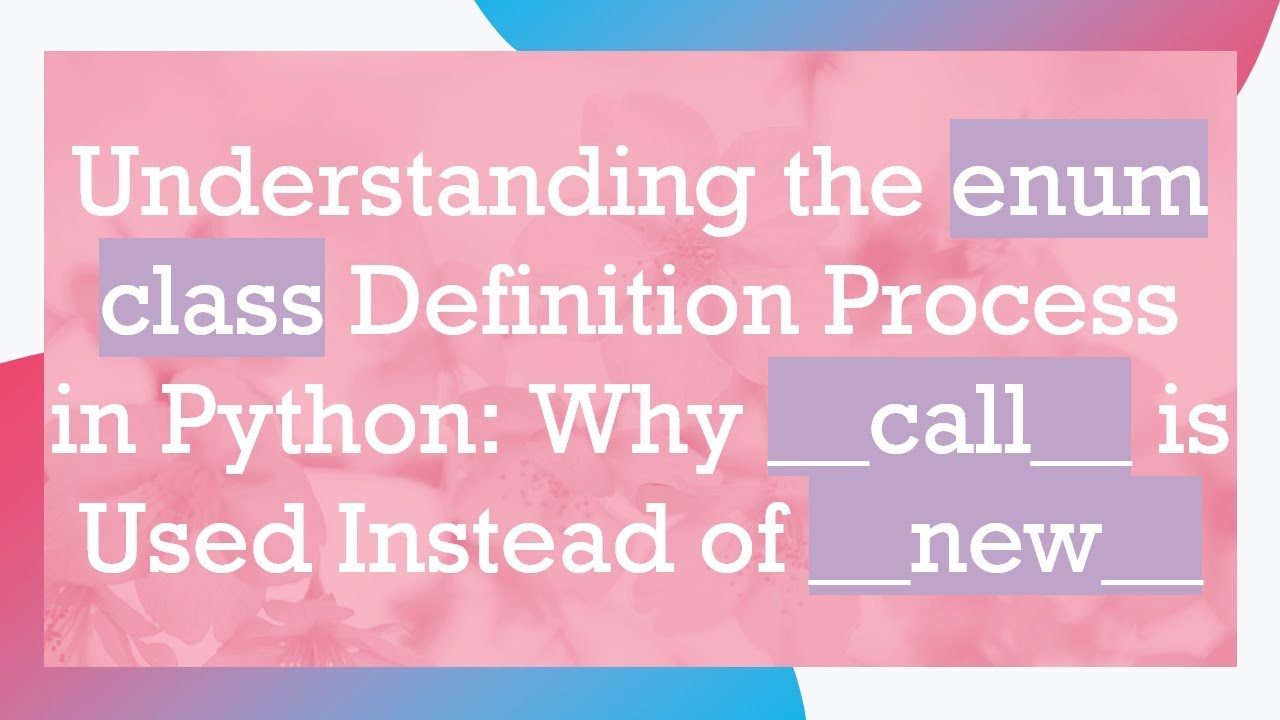
Показать описание
Explore the complexities of Python's `enum class` definition process, focusing on the roles of `__call__` and `__new__` in `EnumMeta` and `Enum`.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: enum class definition process
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the enum class Definition Process in Python: Why __call__ is Used Instead of __new__
When diving into the world of Python enums, you may stumble upon some intricate behaviors in their implementation, particularly regarding the Enum class. A common point of confusion arises around the instantiation process of an Enum class — specifically, understanding why the __call__ method from the metaclass (EnumMeta) is invoked rather than the __new__ method in the Enum. In this post, we’ll clarify this process step-by-step, ensuring that you grasp the essentials of Enum classes in Python.
What Are Enums in Python?
Enums, or Enumerations, are a way to define a set of symbolic names (members) bound to unique, constant values. They are especially useful when you want to represent a fixed set of related constants in a more readable way. For example:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, Color.RED, Color.GREEN, and Color.BLUE are members of our Color enum.
Breakdown of the Enum Class Instantiation
Let’s abbreviate a key aspect of the Enum's underlying process. When you create a new Enum class, two significant components come into play:
Enum class itself
EnumMeta metaclass
The Role of __new__
Every class in Python defines its own __new__ method which is responsible for creating an instance of that class. To put it simply:
__new__ is called when you’re instantiating an object, allowing you to control how new instances are created.
For example:
[[See Video to Reveal this Text or Code Snippet]]
Here, the Buffer class has a custom __new__ that manages instance creation.
The Role of EnumMeta
In contrast, EnumMeta is a metaclass responsible for creating Enum classes themselves. This is crucial because when you define a new Enum class like Color, it is not just a plain class but an instance of EnumMeta. This behavior results in two layers of instantiation:
EnumMeta.__new__ creates an instance of the Enum class (like Color).
Enum.__new__ is later invoked to create instances representing the members (like Color.RED).
Why Is __call__ Used Instead of __new__?
So, why does __call__ of EnumMeta get activated before __new__ of Enum? The __call__ method of a metaclass is executed when an instance of that metaclass is called, which happens when we are creating a new Enum class. Here's how it all fits together:
When you define a new Enum class, Python uses EnumMeta.__call__ to facilitate the class creation process.
Inside __call__, it triggers EnumMeta.__new__ to create the Enum class itself.
Only after the Enum class is created do we enter the territory of Enum.__new__, which is concerned with creating member instances of that Enum.
Members as Instances
It is also noteworthy that the Enum members are not merely attributes; they are truly instances of the Enum class. This nuance adds to the confusion but is part of what makes Enums powerful.
In Summary
To recap, when you instantiate an Enum class:
EnumMeta.__call__ is utilized to create the Enum class.
Enum.__new__ is called to create instances of the Enum’s members.
By understanding the distinction between metaclasses and classes themselves, you can gain clearer insights into the enum class definition process in Python. This knowledge lays a strong foundation for exploring more advanced functionalities within enums and metaclasses.
Feel free to share your thoughts or ask questions in the comments below!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: enum class definition process
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the enum class Definition Process in Python: Why __call__ is Used Instead of __new__
When diving into the world of Python enums, you may stumble upon some intricate behaviors in their implementation, particularly regarding the Enum class. A common point of confusion arises around the instantiation process of an Enum class — specifically, understanding why the __call__ method from the metaclass (EnumMeta) is invoked rather than the __new__ method in the Enum. In this post, we’ll clarify this process step-by-step, ensuring that you grasp the essentials of Enum classes in Python.
What Are Enums in Python?
Enums, or Enumerations, are a way to define a set of symbolic names (members) bound to unique, constant values. They are especially useful when you want to represent a fixed set of related constants in a more readable way. For example:
[[See Video to Reveal this Text or Code Snippet]]
In the above code, Color.RED, Color.GREEN, and Color.BLUE are members of our Color enum.
Breakdown of the Enum Class Instantiation
Let’s abbreviate a key aspect of the Enum's underlying process. When you create a new Enum class, two significant components come into play:
Enum class itself
EnumMeta metaclass
The Role of __new__
Every class in Python defines its own __new__ method which is responsible for creating an instance of that class. To put it simply:
__new__ is called when you’re instantiating an object, allowing you to control how new instances are created.
For example:
[[See Video to Reveal this Text or Code Snippet]]
Here, the Buffer class has a custom __new__ that manages instance creation.
The Role of EnumMeta
In contrast, EnumMeta is a metaclass responsible for creating Enum classes themselves. This is crucial because when you define a new Enum class like Color, it is not just a plain class but an instance of EnumMeta. This behavior results in two layers of instantiation:
EnumMeta.__new__ creates an instance of the Enum class (like Color).
Enum.__new__ is later invoked to create instances representing the members (like Color.RED).
Why Is __call__ Used Instead of __new__?
So, why does __call__ of EnumMeta get activated before __new__ of Enum? The __call__ method of a metaclass is executed when an instance of that metaclass is called, which happens when we are creating a new Enum class. Here's how it all fits together:
When you define a new Enum class, Python uses EnumMeta.__call__ to facilitate the class creation process.
Inside __call__, it triggers EnumMeta.__new__ to create the Enum class itself.
Only after the Enum class is created do we enter the territory of Enum.__new__, which is concerned with creating member instances of that Enum.
Members as Instances
It is also noteworthy that the Enum members are not merely attributes; they are truly instances of the Enum class. This nuance adds to the confusion but is part of what makes Enums powerful.
In Summary
To recap, when you instantiate an Enum class:
EnumMeta.__call__ is utilized to create the Enum class.
Enum.__new__ is called to create instances of the Enum’s members.
By understanding the distinction between metaclasses and classes themselves, you can gain clearer insights into the enum class definition process in Python. This knowledge lays a strong foundation for exploring more advanced functionalities within enums and metaclasses.
Feel free to share your thoughts or ask questions in the comments below!