filmov
tv
1038. Binary Search Tree to Greater Sum Tree - English Version
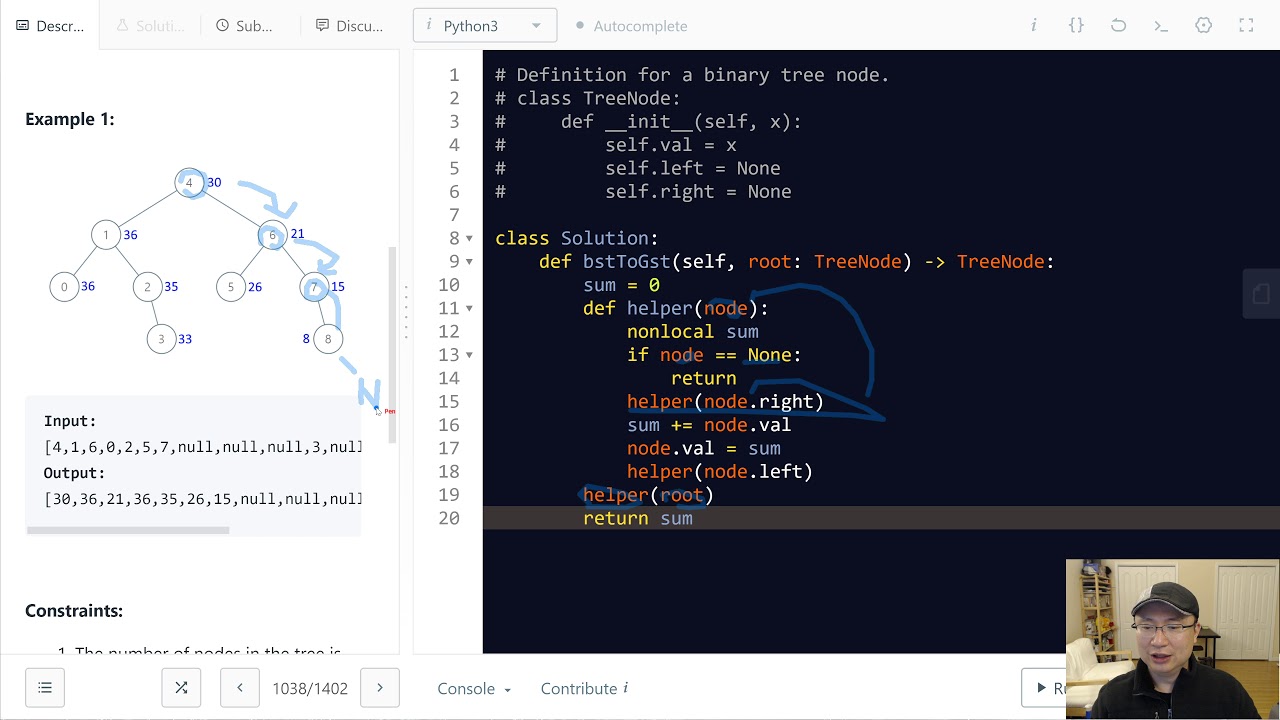
Показать описание
Convert BST to Greater Tree - Leetcode 538 - Python
LeetCode #1038 - Binary Search Tree to Greater Sum Tree
1038. Binary Search Tree to Greater Sum Tree (Leetcode Medium)
Binary Search Tree to Greater Sum Tree - Leetcode 1038 - Java
1038. Binary Search Tree to Greater Sum Tree - English Version
1038. Binary Search Tree to Greater Sum Tree
1038 Binary Search Tree to Greater Sum Tree
1038 Binary Search Tree to Greater Sum Tree
LeetCode 1038. Binary Search Tree to Greater Sum Tree Solution Explained - Java
1038. Binary Search Tree to Greater Sum Tree | Recursive | Iterative | Morris Traversal
LeetCode 1038. Binary Search Tree to Greater Sum Tree solution with TypeScript & JavaScript
leetCode 1038 - Binary Search Tree to Greater Sum Tree | JSer - algorithm & JavaScript
LeetCode 1038 | Binary Search Tree to Greater Sum Tree
LeetCode 1038 | Binary Search Tree to Greater Sum Tree | Recursion | Java
leetcode 1038 Binary Search Tree to Greater Sum Tree BST, traversal
✅ Binary Search Tree to Greater Sum Tree - LeetCode 1038 - Reverse Inorder Traversal - Explained
LeetCode | 1038 Binary Search Tree to Greater Sum Tree
LeetCode 1038 Binary Search Tree to Greater Sum Tree
Leetcode 1038 Binary Search Tree to Greater Sum Tree
1038. Binary Search Tree to Greater Sum Tree #leetcode
Leetcode - Convert BST to Greater Tree (Python)
Binary Search Tree to Greater Sum Tree | Brute | Better | Optimal | Leetcode 1038 | codestorywithMIK
1038. Binary Search Tree to Greater Sum Tree #leetcode
1038. Binary Search Tree to Greater Sum Tree (LeetCode, Java)
Комментарии