filmov
tv
Mockito: How to Verify a Method Was Called on an Object Created Within a Method
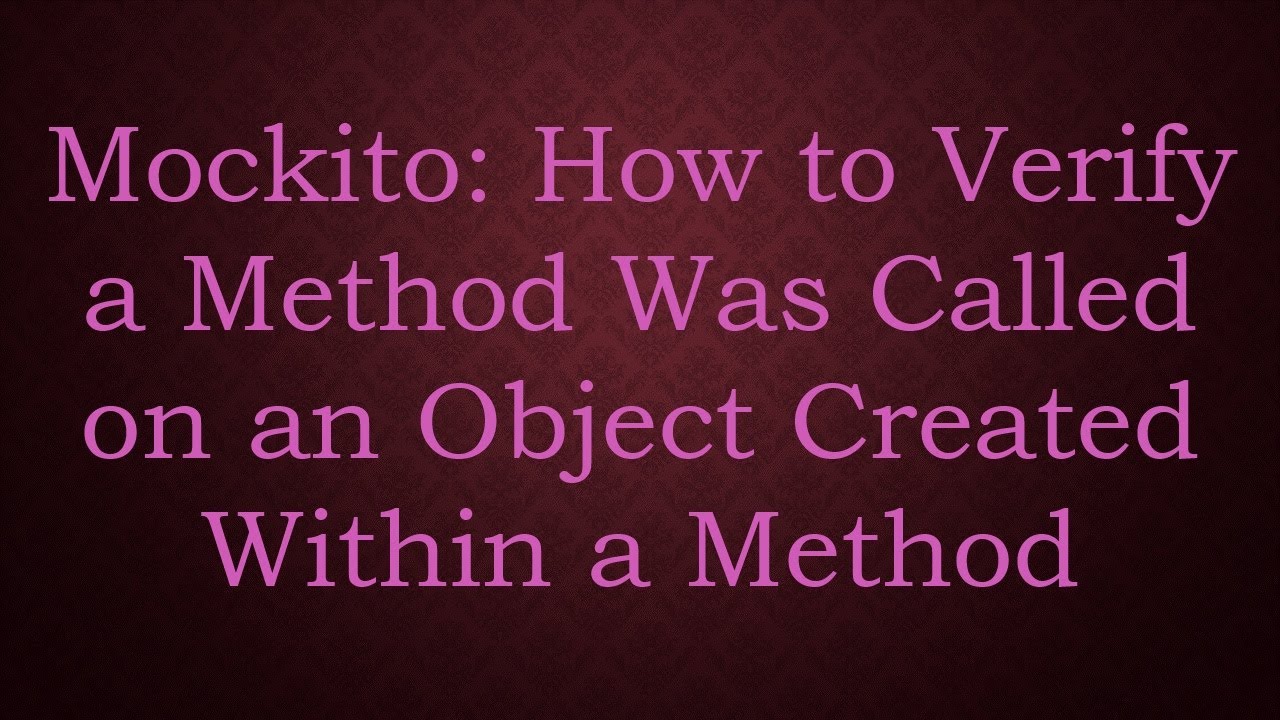
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to use Mockito to verify if a method was called on an object created within another method. This guide covers essential steps and best practices for effective unit testing in Java.
---
When writing unit tests, particularly in Java, Mockito is a powerful tool for creating mock objects and verifying interactions with them. However, a common challenge arises when you need to verify that a method was called on an object that was instantiated within another method. This guide will guide you through the steps to achieve this using Mockito.
Understanding the Scenario
Consider a situation where you have a class with a method that creates an object and calls one of its methods. Here's a simplified example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, performAction creates an instance of MyDependency and calls its doSomething method. The challenge is to verify that doSomething was indeed called.
Steps to Verify Method Call
Refactor to Use a Factory or Provider:
The direct creation of MyDependency within performAction makes it difficult to mock. To address this, you can introduce a factory or provider for MyDependency:
[[See Video to Reveal this Text or Code Snippet]]
Create the Test Class:
With the factory in place, you can now write a test to verify the method call using Mockito:
[[See Video to Reveal this Text or Code Snippet]]
Running the Test:
When you run this test, Mockito will verify that doSomething was called on the dependencyMock instance created by the mocked MyDependencyFactory.
Additional Considerations
Avoid Over-Mocking: Only mock dependencies that are complex or have external interactions. Simple objects might not need to be mocked.
Use Annotations: You can use Mockito annotations to simplify your test setup:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Verifying method calls on objects created within a method can be challenging, but by refactoring your code to use factories or providers, you can leverage Mockito to achieve effective unit tests. This approach not only makes your tests more manageable but also improves the design of your code by promoting dependency injection.
By following the steps outlined in this guide, you can ensure that your methods are behaving as expected and that your dependencies are being used correctly. Happy testing!
---
Summary: Learn how to use Mockito to verify if a method was called on an object created within another method. This guide covers essential steps and best practices for effective unit testing in Java.
---
When writing unit tests, particularly in Java, Mockito is a powerful tool for creating mock objects and verifying interactions with them. However, a common challenge arises when you need to verify that a method was called on an object that was instantiated within another method. This guide will guide you through the steps to achieve this using Mockito.
Understanding the Scenario
Consider a situation where you have a class with a method that creates an object and calls one of its methods. Here's a simplified example:
[[See Video to Reveal this Text or Code Snippet]]
In this example, performAction creates an instance of MyDependency and calls its doSomething method. The challenge is to verify that doSomething was indeed called.
Steps to Verify Method Call
Refactor to Use a Factory or Provider:
The direct creation of MyDependency within performAction makes it difficult to mock. To address this, you can introduce a factory or provider for MyDependency:
[[See Video to Reveal this Text or Code Snippet]]
Create the Test Class:
With the factory in place, you can now write a test to verify the method call using Mockito:
[[See Video to Reveal this Text or Code Snippet]]
Running the Test:
When you run this test, Mockito will verify that doSomething was called on the dependencyMock instance created by the mocked MyDependencyFactory.
Additional Considerations
Avoid Over-Mocking: Only mock dependencies that are complex or have external interactions. Simple objects might not need to be mocked.
Use Annotations: You can use Mockito annotations to simplify your test setup:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Verifying method calls on objects created within a method can be challenging, but by refactoring your code to use factories or providers, you can leverage Mockito to achieve effective unit tests. This approach not only makes your tests more manageable but also improves the design of your code by promoting dependency injection.
By following the steps outlined in this guide, you can ensure that your methods are behaving as expected and that your dependencies are being used correctly. Happy testing!