filmov
tv
Methods to Iterate Through a HashMap in Java
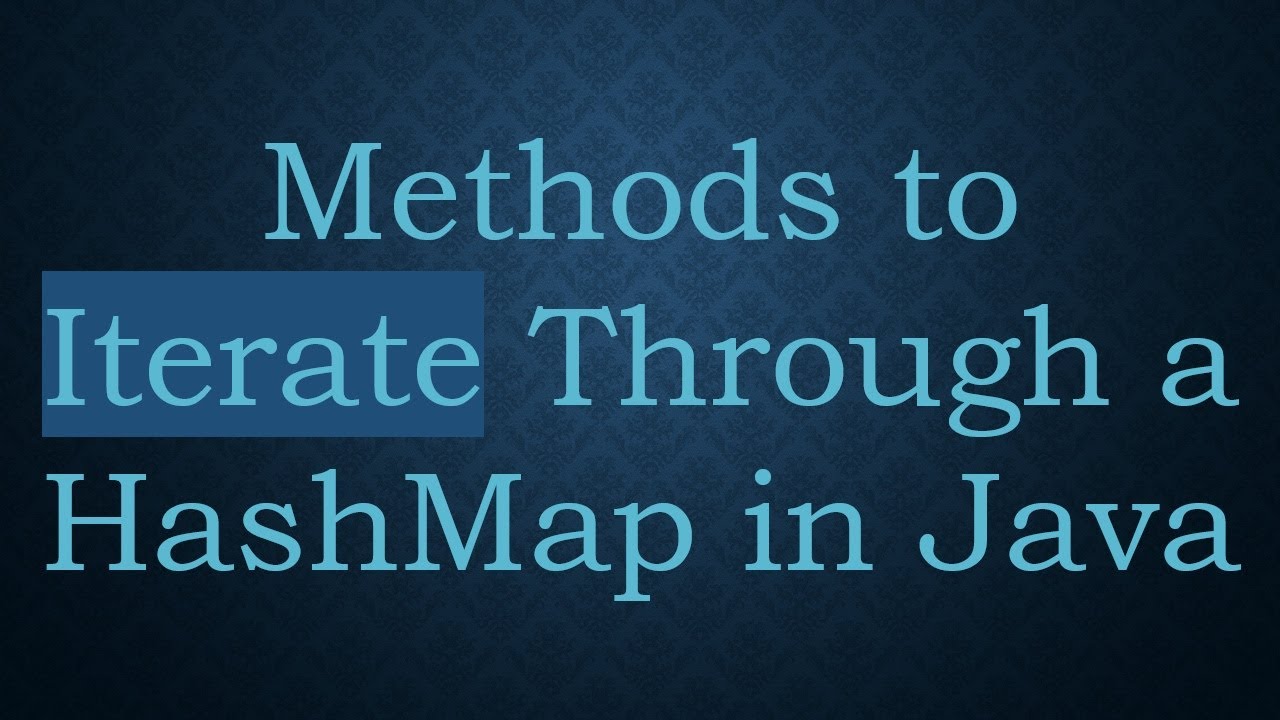
Показать описание
Summary: Learn different methods to iterate through a HashMap in Java for intermediate and advanced Java developers. Find out the best approaches for efficient data handling.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When working with collections in Java, the HashMap is one of the most important data structures due to its ability to store key-value pairs efficiently. Once you have your data stored, however, you might need to iterate through a HashMap to access or modify these pairs according to your requirements. Below are different methods that can be used to iterate through a HashMap:
Using an Iterator
The Iterator method is one of the oldest ways to traverse a HashMap. You first get the entry set from the HashMap, then use the Iterator to loop through it.
[[See Video to Reveal this Text or Code Snippet]]
Enhanced for-each Loop
Introduced in Java 1.5, the enhanced for-each loop provides a more readable way to iterate over a HashMap:
[[See Video to Reveal this Text or Code Snippet]]
Java 8 forEach() Method
Since Java 8, the forEach() method has been available on collections, including HashMap. It uses a lambda expression for iteration, offering a functional programming style approach:
[[See Video to Reveal this Text or Code Snippet]]
Using Stream API
Another Java 8 feature is the Stream API, which allows you to create a stream from the HashMap entry set and perform operations on it:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The method you choose to iterate through a HashMap may depend on readability, performance needs, or personal coding style preferences. The modern lambda expressions and Stream API offer a more concise and functional approach, which can be especially beneficial if you are already utilizing Java 8 or higher in your code. However, traditional Iterator and for-each loops can still be effective, particularly for those who value straightforward syntax and have no need for the additional features provided by Java 8.
Understanding these different techniques not only helps in effective manipulation of the HashMap but also increases your versatility as a Java developer. Whether your focus is on maintaining legacy systems or developing with the latest Java features, knowing these methods enriches your skill set.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When working with collections in Java, the HashMap is one of the most important data structures due to its ability to store key-value pairs efficiently. Once you have your data stored, however, you might need to iterate through a HashMap to access or modify these pairs according to your requirements. Below are different methods that can be used to iterate through a HashMap:
Using an Iterator
The Iterator method is one of the oldest ways to traverse a HashMap. You first get the entry set from the HashMap, then use the Iterator to loop through it.
[[See Video to Reveal this Text or Code Snippet]]
Enhanced for-each Loop
Introduced in Java 1.5, the enhanced for-each loop provides a more readable way to iterate over a HashMap:
[[See Video to Reveal this Text or Code Snippet]]
Java 8 forEach() Method
Since Java 8, the forEach() method has been available on collections, including HashMap. It uses a lambda expression for iteration, offering a functional programming style approach:
[[See Video to Reveal this Text or Code Snippet]]
Using Stream API
Another Java 8 feature is the Stream API, which allows you to create a stream from the HashMap entry set and perform operations on it:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The method you choose to iterate through a HashMap may depend on readability, performance needs, or personal coding style preferences. The modern lambda expressions and Stream API offer a more concise and functional approach, which can be especially beneficial if you are already utilizing Java 8 or higher in your code. However, traditional Iterator and for-each loops can still be effective, particularly for those who value straightforward syntax and have no need for the additional features provided by Java 8.
Understanding these different techniques not only helps in effective manipulation of the HashMap but also increases your versatility as a Java developer. Whether your focus is on maintaining legacy systems or developing with the latest Java features, knowing these methods enriches your skill set.