filmov
tv
JavaScript Tutorial #28 - Cookie vs Local Storage vs Session Storage
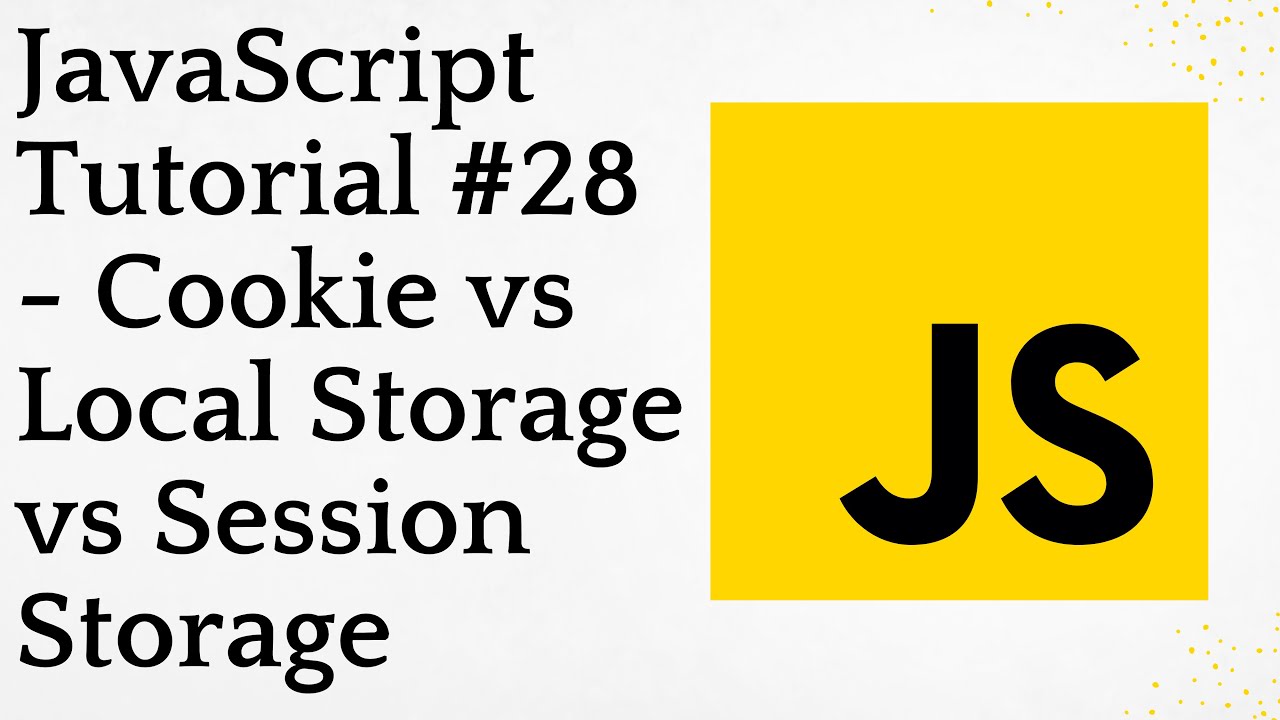
Показать описание
Welcome to Software Interview Prep! Our channel is dedicated to helping software engineers prepare for coding interviews and land their dream jobs. We provide expert tips and insights on everything from data structures and algorithms to system design and behavioral questions. Whether you're just starting out in your coding career or you're a seasoned pro looking to sharpen your skills, our videos will help you ace your next coding interview. Join our community of aspiring engineers and let's conquer the tech interview together!
----------------------------------------------------------------------------------------------------------------------------------------
Cookies, localStorage, and sessionStorage are three mechanisms in web development for storing data on a user's device, but they have distinct differences in terms of storage capacity, lifetime, accessibility, and use cases. Here's a comparison of these three storage options with code examples for each point:
1. **Storage Capacity**:
- **Cookies**:
- Cookies have a small storage capacity, typically limited to a few kilobytes (4KB to 5KB per cookie, depending on the browser).
- This limited capacity is suitable for storing small amounts of data like user preferences or authentication tokens.
- **localStorage**:
- localStorage offers a significantly larger storage capacity compared to cookies, typically around 5-10 MB.
- It is suitable for storing larger amounts of data that need to persist across browser sessions.
- **sessionStorage**:
- sessionStorage has a similar capacity to localStorage, but it's scoped to a single session.
- Data stored in sessionStorage is cleared when the session ends (e.g., when the user closes the tab or browser).
2. **Lifetime**:
- **Cookies**:
- Cookies have an expiration date and can persist beyond the current session.
- You can set a specific expiration date for each cookie, and they remain on the user's device until the expiration date is reached or the user deletes them.
- **localStorage**:
- Data stored in localStorage has no built-in expiration date.
- It persists indefinitely until explicitly removed by the web application or the user clears browser storage.
- **sessionStorage**:
- Data stored in sessionStorage is temporary and tied to a single browsing session.
- It is automatically cleared when the session ends (e.g., when the user closes the tab or browser).
3. **Accessibility**:
- **Cookies**:
- Cookies are accessible both on the client side (from JavaScript) and the server side (via HTTP request headers).
- They are often used for server-side tasks like user authentication.
- **localStorage**:
- localStorage is accessible from client-side JavaScript and can be used to store and retrieve data without sending it to the server.
- **sessionStorage**:
- sessionStorage is also accessible from client-side JavaScript, similar to localStorage.
- Data stored in sessionStorage is scoped to the current browser tab or window and is not shared with other tabs or windows.
Here are code examples demonstrating the differences:
**Cookies**:
```javascript
// Create a cookie
// Read a cookie
// Modify a cookie
// Delete a cookie
```
**localStorage**:
```javascript
// Storing data in localStorage
// Retrieving data from localStorage
// Modifying data in localStorage
// Removing data from localStorage
```
**sessionStorage**:
```javascript
// Storing data in sessionStorage
// Retrieving data from sessionStorage
// Clearing sessionStorage (usually done automatically when the session ends)
```
Choose the storage mechanism that best suits your application's requirements, considering factors like data size, persistence, and accessibility.
----------------------------------------------------------------------------------------------------------------------------------------
Cookies, localStorage, and sessionStorage are three mechanisms in web development for storing data on a user's device, but they have distinct differences in terms of storage capacity, lifetime, accessibility, and use cases. Here's a comparison of these three storage options with code examples for each point:
1. **Storage Capacity**:
- **Cookies**:
- Cookies have a small storage capacity, typically limited to a few kilobytes (4KB to 5KB per cookie, depending on the browser).
- This limited capacity is suitable for storing small amounts of data like user preferences or authentication tokens.
- **localStorage**:
- localStorage offers a significantly larger storage capacity compared to cookies, typically around 5-10 MB.
- It is suitable for storing larger amounts of data that need to persist across browser sessions.
- **sessionStorage**:
- sessionStorage has a similar capacity to localStorage, but it's scoped to a single session.
- Data stored in sessionStorage is cleared when the session ends (e.g., when the user closes the tab or browser).
2. **Lifetime**:
- **Cookies**:
- Cookies have an expiration date and can persist beyond the current session.
- You can set a specific expiration date for each cookie, and they remain on the user's device until the expiration date is reached or the user deletes them.
- **localStorage**:
- Data stored in localStorage has no built-in expiration date.
- It persists indefinitely until explicitly removed by the web application or the user clears browser storage.
- **sessionStorage**:
- Data stored in sessionStorage is temporary and tied to a single browsing session.
- It is automatically cleared when the session ends (e.g., when the user closes the tab or browser).
3. **Accessibility**:
- **Cookies**:
- Cookies are accessible both on the client side (from JavaScript) and the server side (via HTTP request headers).
- They are often used for server-side tasks like user authentication.
- **localStorage**:
- localStorage is accessible from client-side JavaScript and can be used to store and retrieve data without sending it to the server.
- **sessionStorage**:
- sessionStorage is also accessible from client-side JavaScript, similar to localStorage.
- Data stored in sessionStorage is scoped to the current browser tab or window and is not shared with other tabs or windows.
Here are code examples demonstrating the differences:
**Cookies**:
```javascript
// Create a cookie
// Read a cookie
// Modify a cookie
// Delete a cookie
```
**localStorage**:
```javascript
// Storing data in localStorage
// Retrieving data from localStorage
// Modifying data in localStorage
// Removing data from localStorage
```
**sessionStorage**:
```javascript
// Storing data in sessionStorage
// Retrieving data from sessionStorage
// Clearing sessionStorage (usually done automatically when the session ends)
```
Choose the storage mechanism that best suits your application's requirements, considering factors like data size, persistence, and accessibility.