filmov
tv
Difficult Apple Coding Interview Question - JavaScript
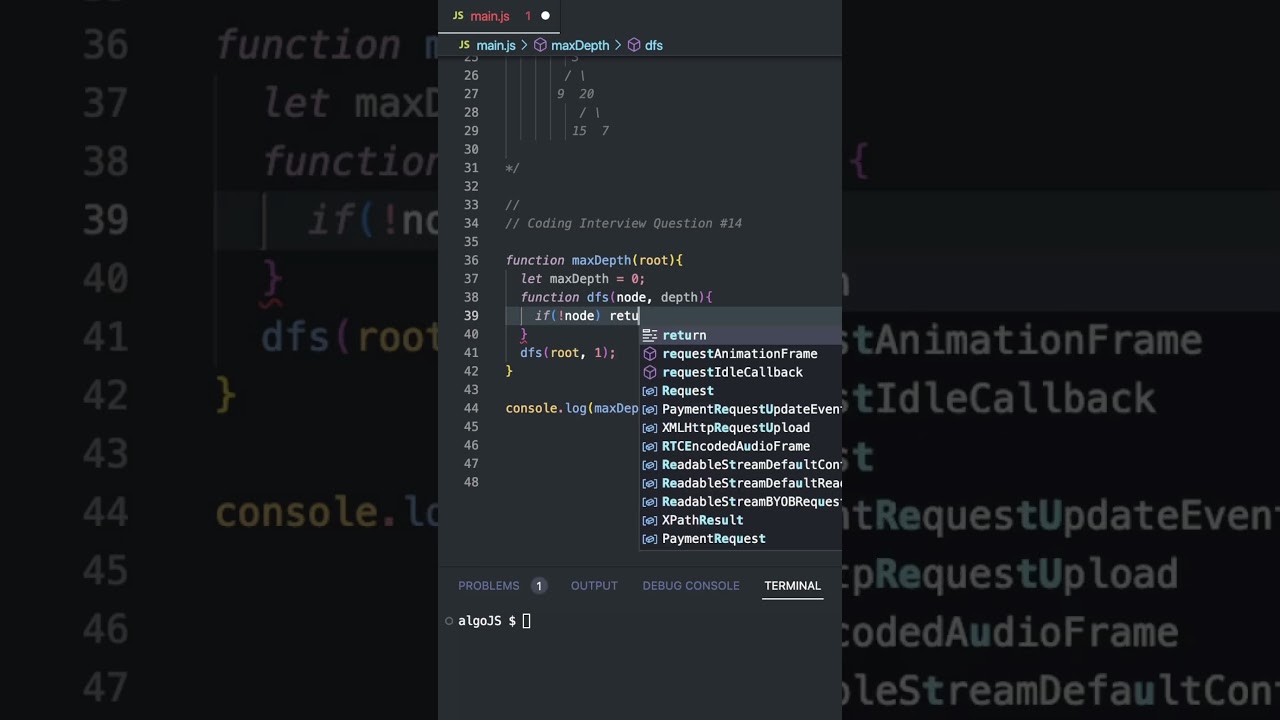
Показать описание
Solution to a very popular coding interview question asked recently by Apple - Maximum Depth of a Binary Tree.
#softwareengineering #javascript #shorts
#softwareengineering #javascript #shorts
Difficult Apple Coding Interview Question - JavaScript
How To Solve This Apple Coding Interview Question With Recursion
Apple Interview Puzzle
Apple Coding Interview Question! | Leetcode 1207 - Unique Number of Occurrences
How to NOT Fail a Technical Interview
Worst Coding Interview
Puzzles for Software Engineers - Apple #2
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
Most Asked Coding Interview Question (Don't Skip !!😮) #shorts
Apple Machine Learning Coding Interview: Calculating Minimum Distance
Top Apple Interview Coding questions | Perfect eLearning
Coding Interview | Software Engineer @ Apple
Apple Quality Engineer Interview Questions and Answers - Apple Test Engineer Interview Questions
Can you Solve this Google Interview Question? | Puzzle for Software Developers
My Experience Interviewing with Apple as an Engineer
How to answer 36 Questions Asked in 97% Apple Interviews
Python interview with an Apple engineer: Count islands
ANYONE can Crack Coding Interviews by Doing THIS
Guide to Apple IOS Engineer Interview Process, Questions and Tips
Apple Quality Engineer Interview Questions and Answers - Apple Test Engineer Interview Questions
Most Tech Interview Prep is GARBAGE. (From a Principal Engineer at Amazon)
Can you answer this Apple job interview question?
Coding Interviews Be Like
Solving JavaScript's Most NOTORIOUS Interview Question
Комментарии