filmov
tv
20 Advanced Coding Tips For Big Unity Projects
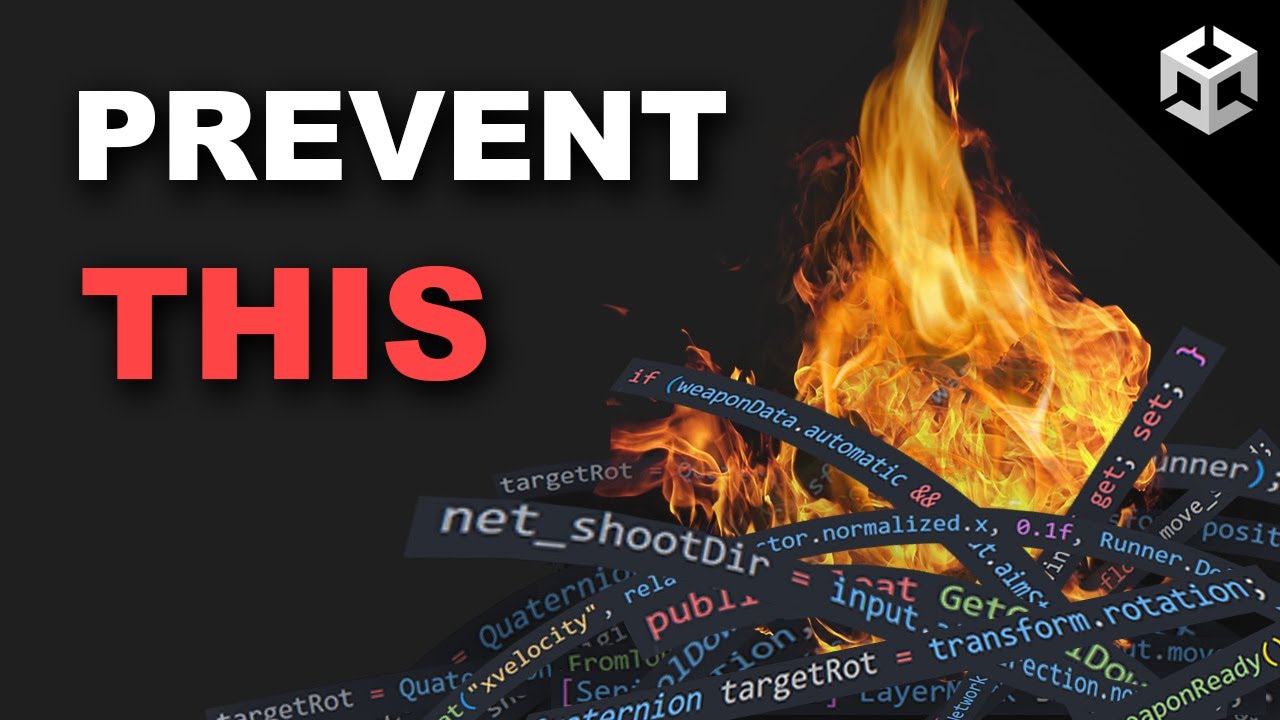
Показать описание
End spaghetti code! Learn the tools you need to write scalable, well-structured, clean code. So many game developers are forced to scrap their ambitious Unity games because they don't know these tips. As a young, self taught game developer, I didn’t discover these tools and techniques for years. Hopefully this video will help you to skip the learning curve and expose you to some of the more advanced programming devices that don’t get enough attention from the plethora of beginner Unity tutorials.
//chapters
00:00 - Intro
01:12 - Variable Names
02:11 - Comments
02:58 - Encapsulate in Functions
03:36 - Plan Your Code
03:57 - C# Properties
04:38 - Serialize Field
05:02 - Component Architecture
06:00 - Enums
06:25 - Coroutines
07:32 - Invoke/Invoke Repeating
08:03 - Structs
08:50 - Singletons
10:38 - C# Events
12:34 - Unity Events
12:56 - Interfaces
14:34 - Inheritance
17:50 - Scriptable Objects
19:15 - Custom Editor Tools
20:25 - Use Version Control
21:00 - Refactor Often
21:38 - Outro
//socials
TikTok: tesseractyt
//long description
So you finally decided to begin work on your “dream game”, a fantasy MMO RPG sandbox battle royale powered by a blockchain economy. What could possibly go wrong? Then, two months later, progress comes to a grinding halt. You have scripts that are a thousand lines long, you’ve forgotten what your old code does, adding new features means you have to rewrite three old ones, every script relies on every other one, and overall, your project becomes an unorganized, unmanageable, confusing, dumpster fire of spaghetti code. Tragically, you are forced to scrap the project and give up on your game dev dreams. Sound familiar?
There are hundreds of hours of Unity tutorials online, but very few are geared towards more advanced developers aiming to create large scale commercial games. That’s why I’ve compiled a list of some of the most valuable unity coding tips that I’ve learned over the years, along with examples of how I’ve actually used these techniques in my own game. Hopefully, by the end of the video, you’ll have the tools you need to write scalable, well structured, clean code that won’t come back to bite you down the road.
//music
Evan King - Nightmares and Violent Shapes
Internet Historian: Sthlm Sunset - Ehrling, A.X - Ehrling, Night Out - LiQWYD
Music from Uppbeat (free for Creators!):
License code: VVIYH4NIZH0ARSHF
License code: AMYFJECHAZAJGCMI
License code: Z6V3SJ5TMSRFUKKU
License code: F9CFDRM8JFBQIVOD
//hashtags
#unity #unity3d #unitytutorial #gamedevelopment #coding #programming #indiegame #cleancode #codingtips
//chapters
00:00 - Intro
01:12 - Variable Names
02:11 - Comments
02:58 - Encapsulate in Functions
03:36 - Plan Your Code
03:57 - C# Properties
04:38 - Serialize Field
05:02 - Component Architecture
06:00 - Enums
06:25 - Coroutines
07:32 - Invoke/Invoke Repeating
08:03 - Structs
08:50 - Singletons
10:38 - C# Events
12:34 - Unity Events
12:56 - Interfaces
14:34 - Inheritance
17:50 - Scriptable Objects
19:15 - Custom Editor Tools
20:25 - Use Version Control
21:00 - Refactor Often
21:38 - Outro
//socials
TikTok: tesseractyt
//long description
So you finally decided to begin work on your “dream game”, a fantasy MMO RPG sandbox battle royale powered by a blockchain economy. What could possibly go wrong? Then, two months later, progress comes to a grinding halt. You have scripts that are a thousand lines long, you’ve forgotten what your old code does, adding new features means you have to rewrite three old ones, every script relies on every other one, and overall, your project becomes an unorganized, unmanageable, confusing, dumpster fire of spaghetti code. Tragically, you are forced to scrap the project and give up on your game dev dreams. Sound familiar?
There are hundreds of hours of Unity tutorials online, but very few are geared towards more advanced developers aiming to create large scale commercial games. That’s why I’ve compiled a list of some of the most valuable unity coding tips that I’ve learned over the years, along with examples of how I’ve actually used these techniques in my own game. Hopefully, by the end of the video, you’ll have the tools you need to write scalable, well structured, clean code that won’t come back to bite you down the road.
//music
Evan King - Nightmares and Violent Shapes
Internet Historian: Sthlm Sunset - Ehrling, A.X - Ehrling, Night Out - LiQWYD
Music from Uppbeat (free for Creators!):
License code: VVIYH4NIZH0ARSHF
License code: AMYFJECHAZAJGCMI
License code: Z6V3SJ5TMSRFUKKU
License code: F9CFDRM8JFBQIVOD
//hashtags
#unity #unity3d #unitytutorial #gamedevelopment #coding #programming #indiegame #cleancode #codingtips
Комментарии