filmov
tv
Embracing Functional Programming in C++
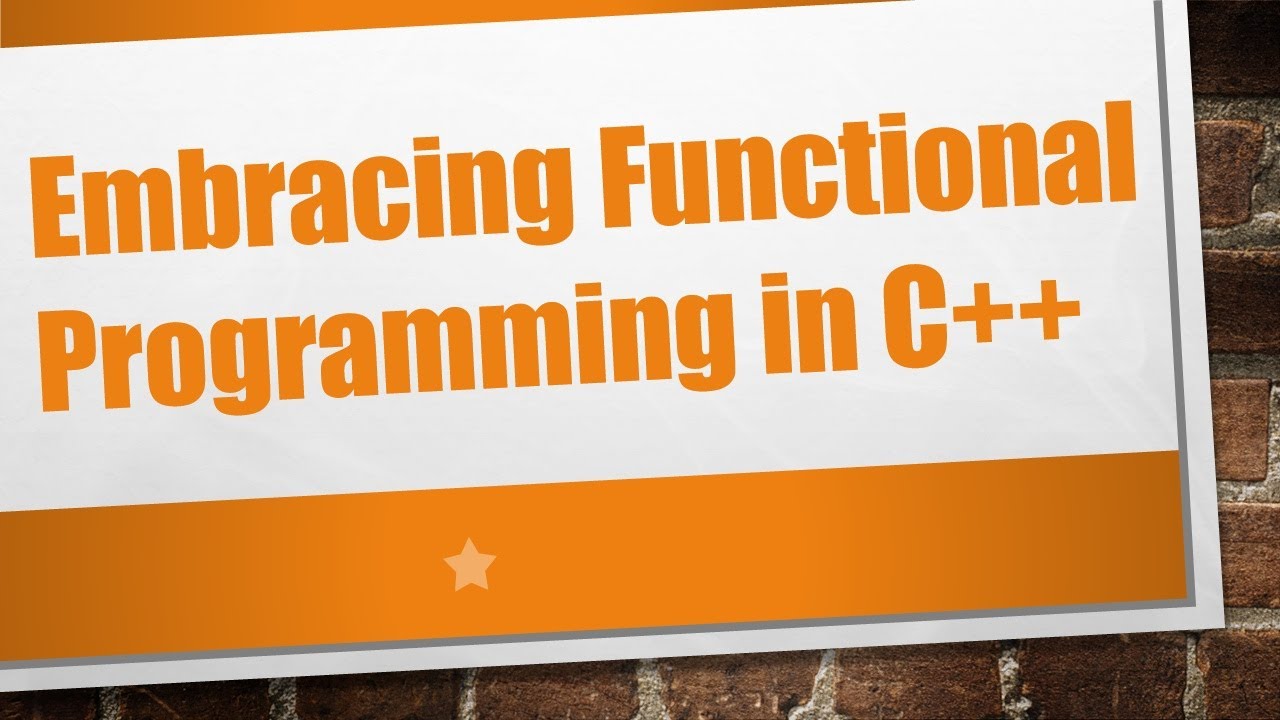
Показать описание
Dive into functional programming in C++ to understand how combining functional paradigms with object-oriented features can enhance your coding practices and software performance.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Embracing Functional Programming in C++
Functional programming has been garnering significant attention in recent years, transcending its origins in academia to become a popular approach in the modern software development landscape. When people think of functional programming, languages like Haskell, Erlang, or Scala often come to mind. However, C++, traditionally seen as an imperative and object-oriented language, has steadily adopted functional programming features over the years. This guide explores how you can leverage these features to enhance your C++ projects.
Understanding Functional Programming
At its core, functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. The core principles include:
Pure Functions: Functions that always produce the same output for the same input and have no side-effects.
Immutability: Data objects are immutable once created.
First-Class and Higher-Order Functions: Functions are treated as first-class citizens and can be assigned to variables, passed as arguments, and returned from other functions.
Declarative Code: Describing what needs to be done rather than how to do it.
Applying Functional Programming in C++
C++ has evolved considerably, particularly with the advent of the C++11 standard, and subsequent iterations have introduced features that allow functional programming techniques to be more easily applied. Here are some key features:
Lambda Expressions
Lambda expressions provide a concise way to define anonymous function objects (functors). They capture variables from the enclosing scope and can be used in place of function pointers or to pass functions as arguments.
[[See Video to Reveal this Text or Code Snippet]]
std::function and Higher-Order Functions
std::function is a versatile and type-safe wrapper for callable objects, supporting function pointers, lambda expressions, and other function objects.
[[See Video to Reveal this Text or Code Snippet]]
Immutable Data Structures
While C++ itself does not enforce immutability, conscientious use of const ensures data integrity. Libraries like boost::hana further support immutable data structures and functional programming constructs.
[[See Video to Reveal this Text or Code Snippet]]
Functional Features in Standard Library
The C++ Standard Library, particularly since C++11, has included a range of utilities and functions that support functional programming paradigms.
Algorithms: Functions like std::transform, std::accumulate, and std::copy_if allow for functional-style operations on collections.
Ranges: Introduced in C++20, ranges allow for a more declarative style of working with sequences, further simplifying code and enhancing readability.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Functional programming in C++ offers a powerful way to write clearer, more predictable, and more maintainable code. By leveraging features such as lambda expressions, std::function, and immutable data structures, developers can blend functional paradigms with C++'s robust object-oriented capabilities. This convergence not only enriches the language but also opens up new avenues for solving complex software challenges.
Whether you're a seasoned C++ developer or new to the language, exploring functional programming concepts can greatly expand your coding toolkit, leading to more robust and expressive software solutions.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Embracing Functional Programming in C++
Functional programming has been garnering significant attention in recent years, transcending its origins in academia to become a popular approach in the modern software development landscape. When people think of functional programming, languages like Haskell, Erlang, or Scala often come to mind. However, C++, traditionally seen as an imperative and object-oriented language, has steadily adopted functional programming features over the years. This guide explores how you can leverage these features to enhance your C++ projects.
Understanding Functional Programming
At its core, functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. The core principles include:
Pure Functions: Functions that always produce the same output for the same input and have no side-effects.
Immutability: Data objects are immutable once created.
First-Class and Higher-Order Functions: Functions are treated as first-class citizens and can be assigned to variables, passed as arguments, and returned from other functions.
Declarative Code: Describing what needs to be done rather than how to do it.
Applying Functional Programming in C++
C++ has evolved considerably, particularly with the advent of the C++11 standard, and subsequent iterations have introduced features that allow functional programming techniques to be more easily applied. Here are some key features:
Lambda Expressions
Lambda expressions provide a concise way to define anonymous function objects (functors). They capture variables from the enclosing scope and can be used in place of function pointers or to pass functions as arguments.
[[See Video to Reveal this Text or Code Snippet]]
std::function and Higher-Order Functions
std::function is a versatile and type-safe wrapper for callable objects, supporting function pointers, lambda expressions, and other function objects.
[[See Video to Reveal this Text or Code Snippet]]
Immutable Data Structures
While C++ itself does not enforce immutability, conscientious use of const ensures data integrity. Libraries like boost::hana further support immutable data structures and functional programming constructs.
[[See Video to Reveal this Text or Code Snippet]]
Functional Features in Standard Library
The C++ Standard Library, particularly since C++11, has included a range of utilities and functions that support functional programming paradigms.
Algorithms: Functions like std::transform, std::accumulate, and std::copy_if allow for functional-style operations on collections.
Ranges: Introduced in C++20, ranges allow for a more declarative style of working with sequences, further simplifying code and enhancing readability.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Functional programming in C++ offers a powerful way to write clearer, more predictable, and more maintainable code. By leveraging features such as lambda expressions, std::function, and immutable data structures, developers can blend functional paradigms with C++'s robust object-oriented capabilities. This convergence not only enriches the language but also opens up new avenues for solving complex software challenges.
Whether you're a seasoned C++ developer or new to the language, exploring functional programming concepts can greatly expand your coding toolkit, leading to more robust and expressive software solutions.