filmov
tv
Longest Palindrome - Leetcode 409 - Python
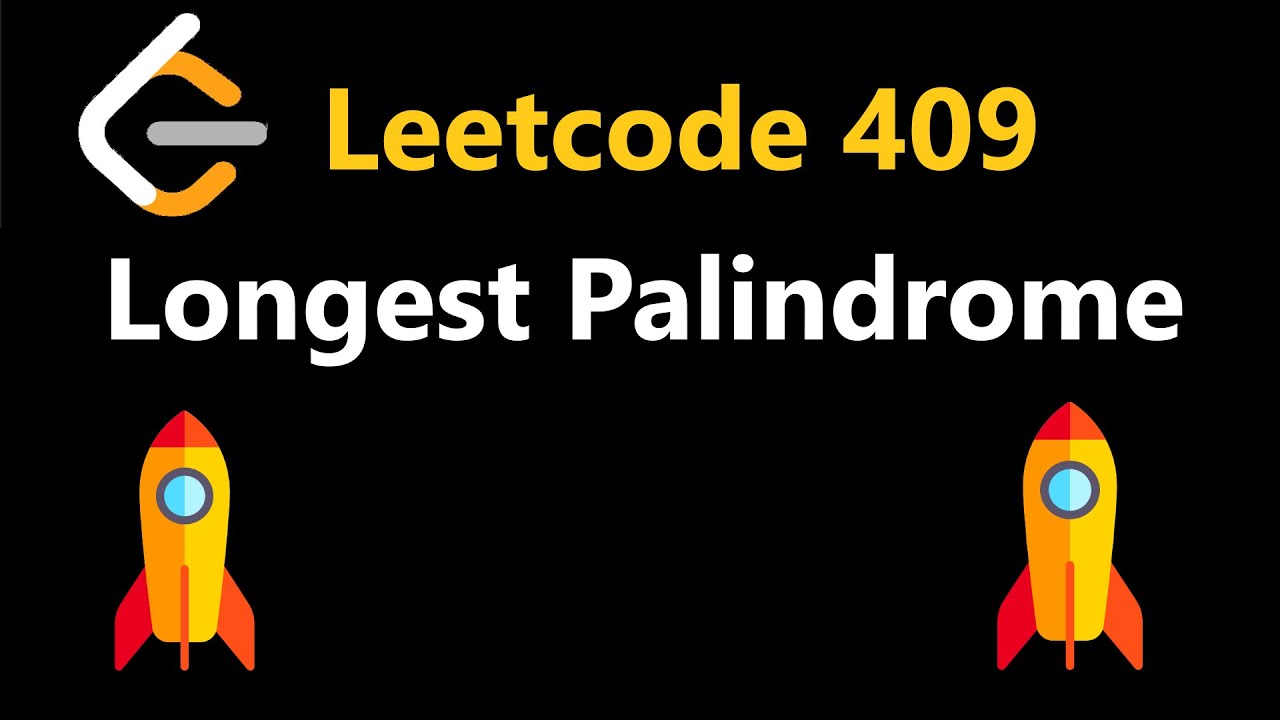
Показать описание
0:00 - Read the problem
0:30 - Drawing Explanation
6:57 - Coding Explanation
leetcode 409
#neetcode #leetcode #python
Longest Palindrome - Leetcode 409 - Python
Longest Palindrome | LeetCode 409 | C++, Java, Python
longest palindrome leetcode | leetcode 409 | With HashSet
Longest Palindrome | Leet code 409 | Theory explained + Python code
Longest Palindrome - Leetcode 409 - Java
Longest Palindrome | 3 Easy Approaches | Leetcode 409 | codestorywithMIK
palindromes were tricky until I learned this
Longest Palindromic Substring - Python - Leetcode 5
LeetCode Longest Palindrome Solution Explained - Java
409. Longest Palindrome (Leetcode Easy)
409. Longest Palindrome | LeetCode 75 | JavaScript | Easy Explanation | Linear Time Complexity
LeetCode 409 - Longest Palindrome
409. Longest Palindrome | Leetcode | Python
LeetCode: 409. Longest Palindrome
409. Longest Palindrome || Java || Leetcode || Hindi
Longest Palindrome - Leetcode 409 - Java
LeetCode 409 - Longest Palindrome - C++
Leetcode 409 - Longest Palindrome
Longest Palindrome - LeetCode 409 - Swift
Longest Palindromic Sub-string (LeetCode 5) | Full solution with examples | Study Algorithms
409. Longest Palindrome | Hash Table | Greedy | Hot Interview Question
Longest Palindrome - LeetCode 409 - JavaScript
leetcode 409 Longest Palindrome (Python)
LeetCode Problem 409: Longest Palindrome
Комментарии