filmov
tv
C++ Programming All-in-One Tutorial Series (10 HOURS!)
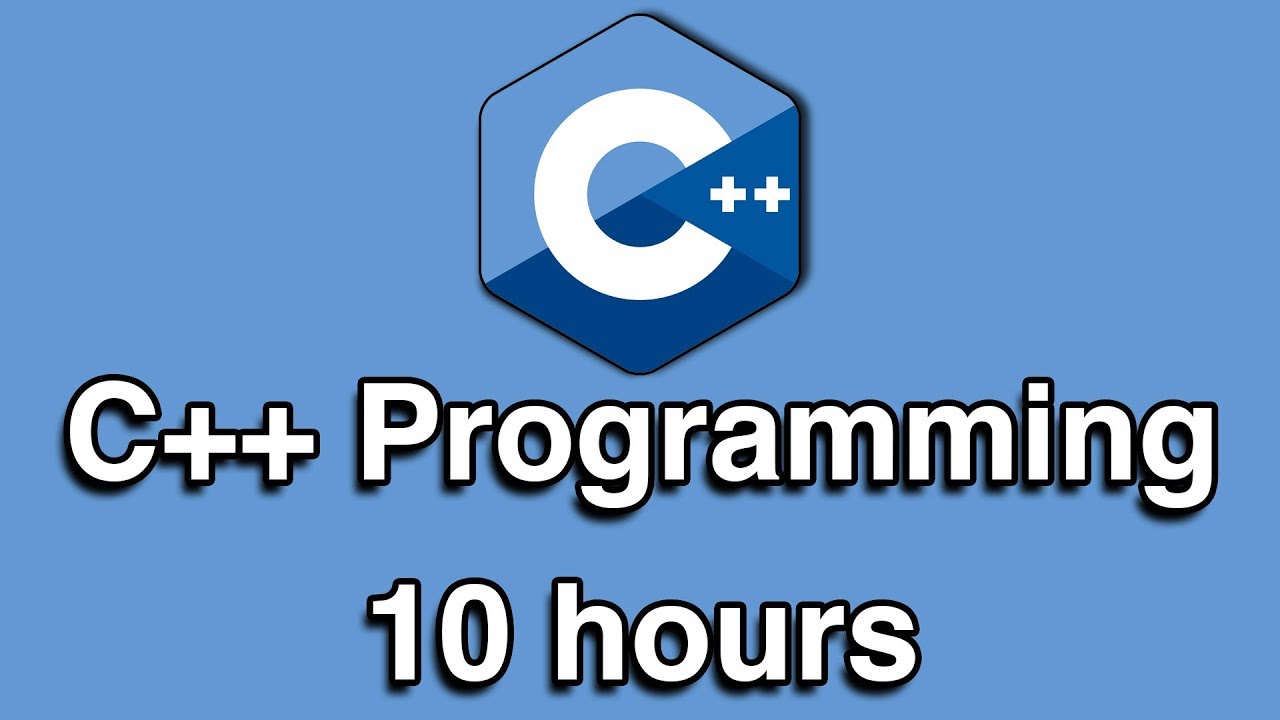
Показать описание
Timestamps:
00:00 - Introduction
09:31 - Installing g++
15:37 - C++ Concepts
22:31 - More C++ Concepts
30:48 - Using Directive and Declaration
37:33 - Variable Declaration and Initialization
40:40 - Using Variables with cout
44:46 - User Input with cin
47:57 - Conventions and Style Guides
56:23 - Intro to Functions
1:01:28 - Intro to Creating Custom Functions
1:09:05 - Pow Function
1:13:13 - Creating Custom Functions
1:22:20 - Creating Void Functions
1:29:11 - Intro to C++ Data Types
1:37:17 - Integral Data Types and Signed vs Unsigned
1:44:38 - Integral Data Types, sizeof, limit
1:49:35 - char Data Type
1:56:52 - Escape Sequences
2:02:21 - bool Data Type
2:06:54 - Floating Point Numbers
2:15:00 - Constant const, macro, and enum
2:21:00 - Numeric Functions
2:28:37 - String Class and C Strings
2:37:47 - get line for Strings
2:40:52 - String Modifier Methods
2:47:56 - String Operation Methods
2:54:36 - Literals
2:59:42 - Hex and Octal
3:04:06 - Operator Precedence and Associativity
3:11:53 - Reviewing Key Concepts
3:17:54 - Control Flow
3:27:32 - If Statement Practice
3:32:33 - Logical and Comparison Operators
3:42:27 - Switch Statement and Enum
3:51:00 - Intro to Loops
3:58:01 - For Loops (How to Calculate Factorial)
4:05:06 - While Loop and Factorial Calculator
4:12:29 - Do While Loop
4:20:04 - Break and Continue
4:25:14 - Conditional Operator
4:29:04 - Intro to Our App
4:33:00 - Creating a Menu
4:38:11 - Creating a Guessing Game
4:45:27 - Intro to Arrays and Vectors
4:54:15 - Working with Arrays
5:04:21 - Passing Arrays to Functions
5:11:11 - Fill Array from Input
5:20:42 - Using and Array to Keep Track of Guessing
5:25:55 - Intro to Vectors
5:33:00 - Creating a Vector
5:36:39 - Passing Vectors to Functions
5:39:55 - Refactor Guessing Game to Use Vectors
5:43:47 - STL Array
5:47:47 - STL Arrays in Practice
5:51:45 - Refactor Guessing Game to Use Templatized Array
5:55:13 - Array vs Vector vs STL Array
6:01:49 - Range Based for Loop
6:07:03 - Intro to IO Streams
6:15:45 - Writing to Files with ofstream
6:24:43 - Readings from Files with ifstream
6:31:13 - Saving High Scores to File
6:39:46 - Functions and Constructors
6:47:53 - Refactoring IO to Function Call and Testing
6:54:31 - Multidimensional Arrays and Nested Vectors
6:59:29 - Const Modifier
7:04:33 - Pass by Reference and Pass By Value
7:11:41 - Swap Function with Pass by Reference
7:14:27 - Intro to Function Overloading
7:19:35 - Function Overloading Examples
7:26:22 - Default Arguments
7:33:24 - Intro to Multifile Compilation
7:40:56 - Multifile Compilation
7:48:15 - Makefiles
7:54:44 - Creating a Simple Makefile
8:01:30 - Intro to Namespaces
8:05:33 - Creating a Namespace
8:10:24 - Intro to Function Templates
8:15:24 - Creating a Function Template
8:18:40 - Overloading Function Templates
8:23:18 - Intro to Object Oriented Programming
8:30:39 - Intro to Structs
8:35:59 - Creating a Struct
8:41:48 - Classes and Object
8:49:58 - Creating a Class
8:52:45 - Working with Objects
9:00:11 - Intro to Constructors
9:05:18 - Constructors and Destructors
9:09:53 - Encapsulation
9:15:40 - Getters and Setters
9:22:47 - Static Data Members
9:29:59 - Intro to Operator Overloading
9:34:07 - Operator Overloading == and +
9:41:13 - Overloading Insert and Extraction Operators
9:49:07 - Friend Functions and Operator Overloading
9:56:14 - Class Across Files
10:03:31 - Inheritance and Polymorphism
10:08:49 - Base Classes and Subclasses Inheritance
10:16:54 - Polymorphism
10:23:15 - Conclusion
~~~~~~~~~~~~~~~ CONNECT ~~~~~~~~~~~~~~~
Комментарии