filmov
tv
How to Use watch in Vue.js for Dynamic Array Updates
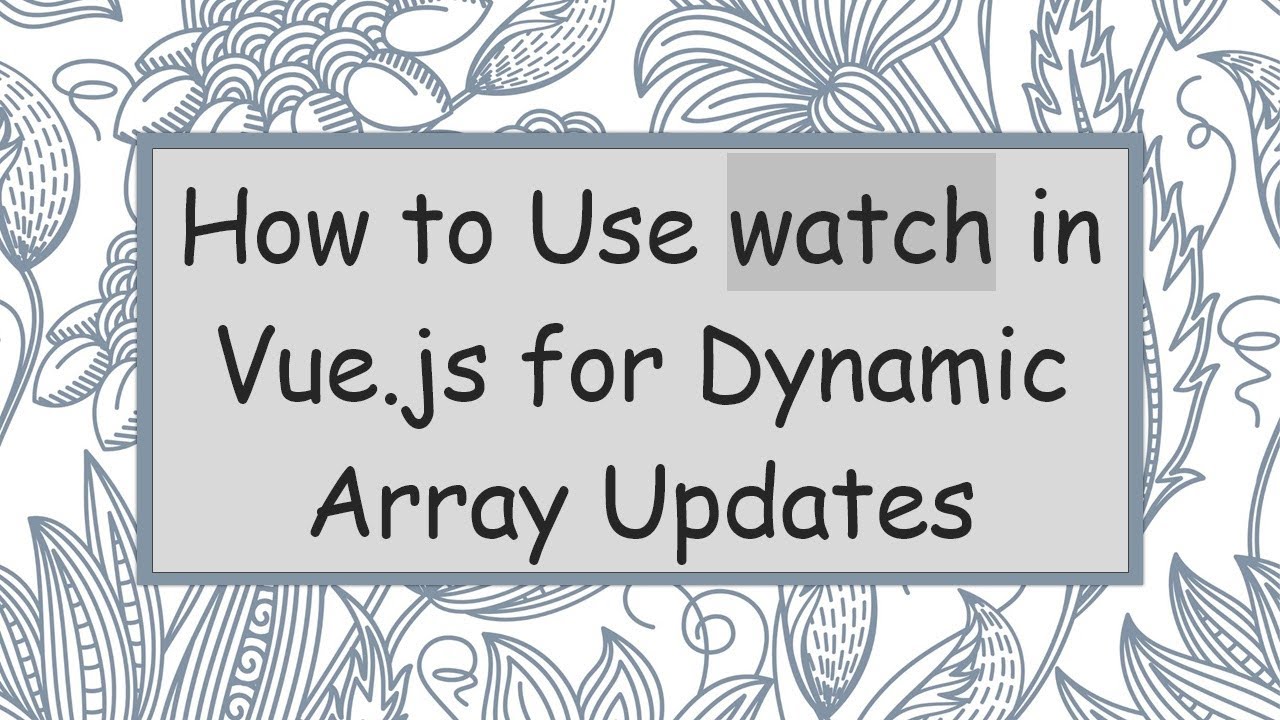
Показать описание
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to use watch in vuejs?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
In this guide, we will break down this common problem and provide a clear solution to ensure your watcher responds appropriately when items are added dynamically to an array.
Understanding the Problem
In the scenario described, the developer is trying to track changes in an array of tickers using Vue's watch feature. Here's a brief overview of the code:
[[See Video to Reveal this Text or Code Snippet]]
Here, the addClick method attempts to add a new ticker to the tickers array. The intention is for the watch on the tickers array to respond to this addition, automatically updating local storage. Instead, the watcher only fires when the page is refreshed.
The Watcher Code
[[See Video to Reveal this Text or Code Snippet]]
At first glance, everything appears to be in order, but the problem lies in how the array is being updated.
The Solution Explained
Update the Reference
The key issue here is that the push method modifies the existing array and keeps the reference of that array the same. Vue's reactivity system relies on detecting changes to the reference of the data being watched. When you push an item into an array, Vue doesn't notice a change in the reference, which is why the watcher doesn't activate.
Correct Way to Add Items
To solve this issue, you should create a new array that includes your current tickers plus the new ticker. This can be done using the spread operator (...), which creates a new reference that Vue will monitor.
Here's how to implement this:
Instead of:
[[See Video to Reveal this Text or Code Snippet]]
Use:
[[See Video to Reveal this Text or Code Snippet]]
Why This Works
By using the spread operator:
New Reference: You are effectively creating a new array, thus changing the reference. Vue will recognize this change.
Reactivity: Vue's reactivity system detects the new array and triggers the watcher, allowing the associated code (like updating local storage) to run as intended.
Summary
Always update the reference of the array when you need the watch to fire:
[[See Video to Reveal this Text or Code Snippet]]
With this technique, your Vue application will behave more predictably and responsively, improving the user experience without needing unnecessary page reloads.
By understanding and implementing these changes, you can leverage Vue's reactivity in powerful ways. Happy coding!
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to use watch in vuejs?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
In this guide, we will break down this common problem and provide a clear solution to ensure your watcher responds appropriately when items are added dynamically to an array.
Understanding the Problem
In the scenario described, the developer is trying to track changes in an array of tickers using Vue's watch feature. Here's a brief overview of the code:
[[See Video to Reveal this Text or Code Snippet]]
Here, the addClick method attempts to add a new ticker to the tickers array. The intention is for the watch on the tickers array to respond to this addition, automatically updating local storage. Instead, the watcher only fires when the page is refreshed.
The Watcher Code
[[See Video to Reveal this Text or Code Snippet]]
At first glance, everything appears to be in order, but the problem lies in how the array is being updated.
The Solution Explained
Update the Reference
The key issue here is that the push method modifies the existing array and keeps the reference of that array the same. Vue's reactivity system relies on detecting changes to the reference of the data being watched. When you push an item into an array, Vue doesn't notice a change in the reference, which is why the watcher doesn't activate.
Correct Way to Add Items
To solve this issue, you should create a new array that includes your current tickers plus the new ticker. This can be done using the spread operator (...), which creates a new reference that Vue will monitor.
Here's how to implement this:
Instead of:
[[See Video to Reveal this Text or Code Snippet]]
Use:
[[See Video to Reveal this Text or Code Snippet]]
Why This Works
By using the spread operator:
New Reference: You are effectively creating a new array, thus changing the reference. Vue will recognize this change.
Reactivity: Vue's reactivity system detects the new array and triggers the watcher, allowing the associated code (like updating local storage) to run as intended.
Summary
Always update the reference of the array when you need the watch to fire:
[[See Video to Reveal this Text or Code Snippet]]
With this technique, your Vue application will behave more predictably and responsively, improving the user experience without needing unnecessary page reloads.
By understanding and implementing these changes, you can leverage Vue's reactivity in powerful ways. Happy coding!