filmov
tv
C Tic Tac Toe game ⭕
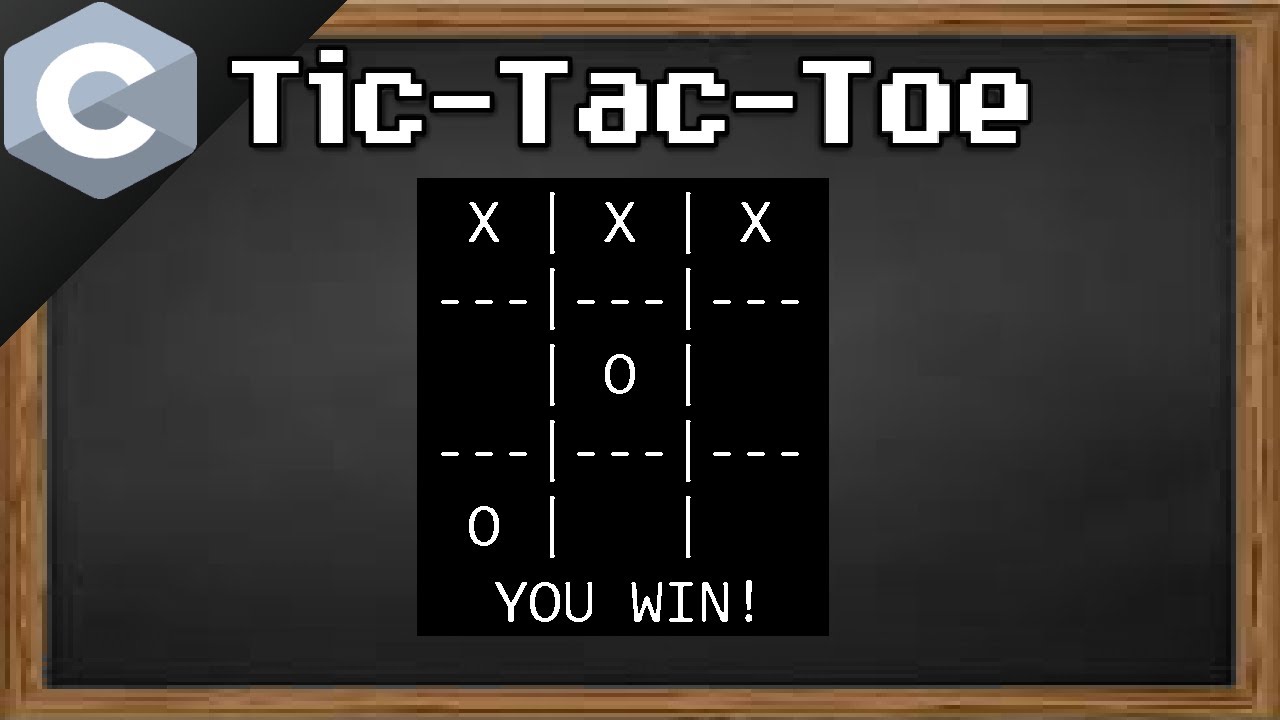
Показать описание
C tic tac toe game for beginners tutorial example explained
#C #game #tictactoe
This is a tictactoe game written in C designed for beginners
(This doesn't contain the useof pointers or other advanced C topics)
#C #game #tictactoe
This is a tictactoe game written in C designed for beginners
(This doesn't contain the useof pointers or other advanced C topics)
C Tic Tac Toe game ⭕
TIC TAC TOE GAME IN C PROGRAMMING || MINI PROJECT IN C
Tic Tac Toe in C programming
Tic Tac Toe | C Programming Mini-Project | Beginners tutorial
[Part 1] Tic-tac-toe Game in C with SDL -- Procedural vs Functional
Tic Tac Toe in C Language📺
How To Make a Tic Tac Toe Game in C++ - QUICK AND EASY TUTORIAL
Tic Tac Toe Game in C++
Minimax Algorithm for Tic Tac Toe (Coding Challenge 154)
Tic-Tac-Toe Game Mini Project In C Programming
Tic Tac Toe game written in C language using Mac OSX
tic tac toe using c programming language
Tic Tac Toe Game in C
Tic Tac Toe Game In C++ Code | How To Make Tic Tac Toe In c++ | C++ Tutorial | Simplilearn
Tic Tac Toe Game in C Programming | TechVidya| Mini project in C language| Step by step tutorial
C Programming, how to code Tic Tac Toe game with ncurses
Tic Tac Toe game 🎮 by C programming || #shorts #game #tictactoe #tictactoegame #coding
Tic Tac Toe Game using C
Tic Tac Toe game using c/c++ and graphics.h library , heavy functionality with easy code
TIC TAC TOE Game Presentation using C Program (Dev ++)
The TIC TAC TOE Drop 🎯 VS @mrblack
Simple tictactoe game with C programming || C Programming || Games || Tictactoe
The Tic Tac Toe Game In C With Output
Tic Tac Toe game using Qt C++
Комментарии