filmov
tv
Free Code Camp Walkthrough 21 | JS - Basic JavaScript
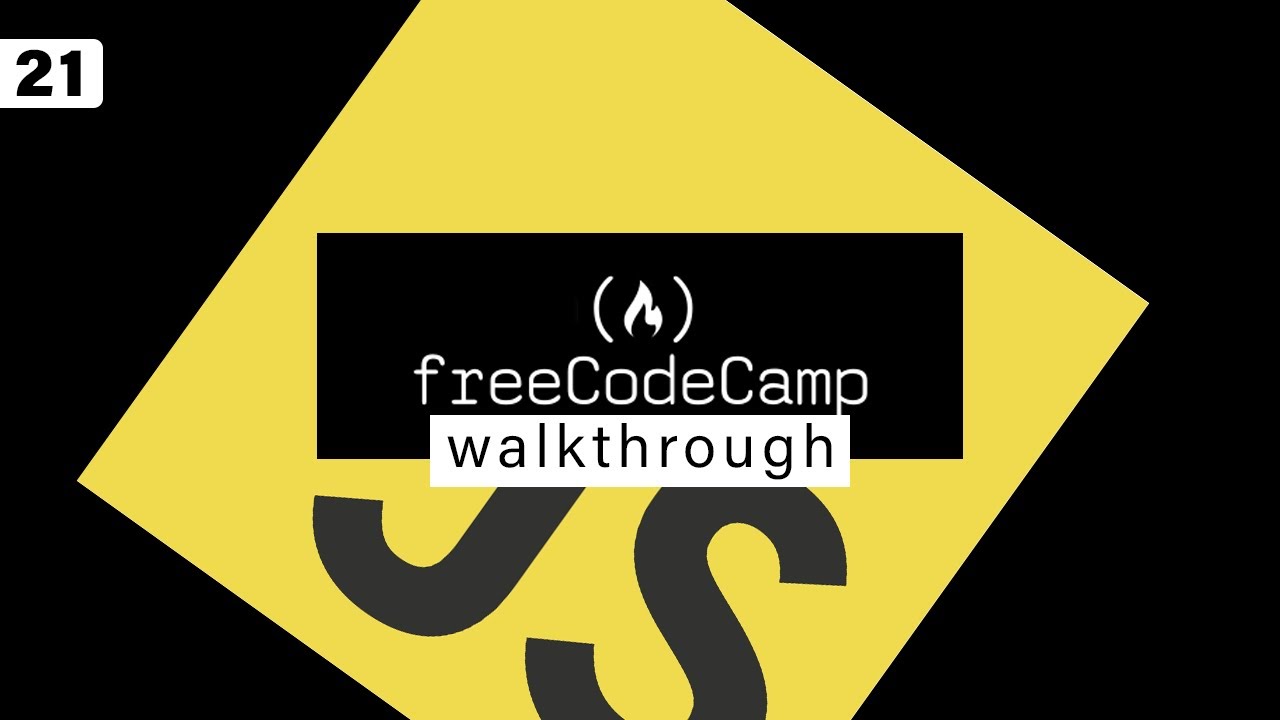
Показать описание
In this tutorial I'll show you how to get through the JS Basic JavaScript course on Free Code Camp.
-------------------------------------------------------------------
-------------------------------------------------------------------
Timestamps
0:00 Intro
0:39 Comments in JS
1:21 Declare Variables
1:55 Storing values with assignment operator
2:19 Assigning values with variables
2:52 Initializing variables with assignment operator
3:15 String variables
4:19 Unitialized variables
5:46 Case sensitivity
6:41 Difference between var and let
8:09 Read-only variable
9:46 Add two numbers
10:07 Subtract one number from another
10:19 Multiply two numbers
10:30 Divide one number
10:40 Increment a number
11:03 Decrement a number
11:20 Decimal number
11:48 Multiply decimals
12:06 Divide decimal by another one
12:23 Finding a remainder
13:41 Augmented addition
14:44 Augmented subtraction
15:32 Augmented multiplication
16:25 Augmented division
17:11 Escaping literal quotes
19:05 Quoting with single quotes
20:58 Escape sequences in strings
22:13 Concatenating with plus operator
23:10 Concatenating with plus equals operator
24:09 Constructing strings with variables
25:20 Appending variables
26:17 Find length of string
27:14 Braket notation to find 1st character
28:06 String immutability
29:15 Braket notation to find nth character
30:00 Braket notation to find last character
31:01 Braket notation to find nth to last character
31:51 Word blanks
33:53 Store multiple values in arrays
34:37 Nested arrays
35:26 Access array data
36:30 Modify array data
37:31 Access multi-dimensional arrays
39:08 push method
40:23 pop method
42:04 shift method
43:13 unshift method
45:00 Shopping list
47:00 Write functions
48:36 Arguments for functions
51:36 Return with functions
54:05 Global scope
55:52 Local scope
57:41 Global vs. local scope
58:57 Undefined values
1:00:31 Assignment with returned value
1:02:03 Stand in line
1:04:07 Boolean values
1:04:47 If statements
1:07:02 Equality operator
1:08:20 Strict equality operator
1:08:56 Comparing different values
1:10:01 Inequality operator
1:10:59 Strict inequality operator
1:12:04 Greater than operator
1:12:48 Greater than or equal operator
1:13:27 Less than operator
1:13:47 Less than or equal operator
1:14:14 Logical and operator
1:16:01 Logical or operator
1:17:42 Else statements
1:18:52 Else if statements
1:20:06 Logical order in if else statements
1:21:21 Chaining if else statements
1:23:01 Golf code
1:26:46 Switch statements
1:29:53 Default option in switch
1:31:41 Multiple identical options in switch
1:33:23 Replace if else with switch
1:35:43 Return boolean values from functions
1:36:55 Return early pattern for functions
1:38:33 Counting cards
1:42:43 Build JS objects
1:45:37 Dot notation for property access
1:47:05 Braket notation for property access
1:48:25 Variables for property access
1:50:37 Updating object properties
1:52:10 Add new properties to a JS object
1:53:08 Delete properties from objects
1:53:53 Using objects for lookups
1:57:42 Testing objects for properties
1:59:20 Manipulating complex objects
2:02:50 Accessing nested objects
2:05:30 Accessing nested arrays
2:08:08 Record Collection
2:16:24 While loops
2:20:16 For loops
2:24:05 Iterate odd numbers with a for loop
2:27:01 Count backwards with a for loop
2:28:50 Iterate through an array with a for loop
2:31:46 Nesting for loops
2:36:03 Do...while loop
2:38:31 Recursion
2:42:12 Profile lookup
2:48:10 Generate random fractions
2:48:59 Generate random whole numbers
2:51:24 Generate random whole numbers within a range
2:55:05 parseInt
2:55:58 parseInt with a radix
2:58:24 Conditional ternary operator
3:00:35 Multiple ternary operators
3:02:42 Recursion for countdown
3:06:12 Recursion for range of numbers
3:08:54 Outro
-------------------------------------------------------------------
Music by Silent Partner
-------------------------------------------------------------------
-------------------------------------------------------------------
-------------------------------------------------------------------
Timestamps
0:00 Intro
0:39 Comments in JS
1:21 Declare Variables
1:55 Storing values with assignment operator
2:19 Assigning values with variables
2:52 Initializing variables with assignment operator
3:15 String variables
4:19 Unitialized variables
5:46 Case sensitivity
6:41 Difference between var and let
8:09 Read-only variable
9:46 Add two numbers
10:07 Subtract one number from another
10:19 Multiply two numbers
10:30 Divide one number
10:40 Increment a number
11:03 Decrement a number
11:20 Decimal number
11:48 Multiply decimals
12:06 Divide decimal by another one
12:23 Finding a remainder
13:41 Augmented addition
14:44 Augmented subtraction
15:32 Augmented multiplication
16:25 Augmented division
17:11 Escaping literal quotes
19:05 Quoting with single quotes
20:58 Escape sequences in strings
22:13 Concatenating with plus operator
23:10 Concatenating with plus equals operator
24:09 Constructing strings with variables
25:20 Appending variables
26:17 Find length of string
27:14 Braket notation to find 1st character
28:06 String immutability
29:15 Braket notation to find nth character
30:00 Braket notation to find last character
31:01 Braket notation to find nth to last character
31:51 Word blanks
33:53 Store multiple values in arrays
34:37 Nested arrays
35:26 Access array data
36:30 Modify array data
37:31 Access multi-dimensional arrays
39:08 push method
40:23 pop method
42:04 shift method
43:13 unshift method
45:00 Shopping list
47:00 Write functions
48:36 Arguments for functions
51:36 Return with functions
54:05 Global scope
55:52 Local scope
57:41 Global vs. local scope
58:57 Undefined values
1:00:31 Assignment with returned value
1:02:03 Stand in line
1:04:07 Boolean values
1:04:47 If statements
1:07:02 Equality operator
1:08:20 Strict equality operator
1:08:56 Comparing different values
1:10:01 Inequality operator
1:10:59 Strict inequality operator
1:12:04 Greater than operator
1:12:48 Greater than or equal operator
1:13:27 Less than operator
1:13:47 Less than or equal operator
1:14:14 Logical and operator
1:16:01 Logical or operator
1:17:42 Else statements
1:18:52 Else if statements
1:20:06 Logical order in if else statements
1:21:21 Chaining if else statements
1:23:01 Golf code
1:26:46 Switch statements
1:29:53 Default option in switch
1:31:41 Multiple identical options in switch
1:33:23 Replace if else with switch
1:35:43 Return boolean values from functions
1:36:55 Return early pattern for functions
1:38:33 Counting cards
1:42:43 Build JS objects
1:45:37 Dot notation for property access
1:47:05 Braket notation for property access
1:48:25 Variables for property access
1:50:37 Updating object properties
1:52:10 Add new properties to a JS object
1:53:08 Delete properties from objects
1:53:53 Using objects for lookups
1:57:42 Testing objects for properties
1:59:20 Manipulating complex objects
2:02:50 Accessing nested objects
2:05:30 Accessing nested arrays
2:08:08 Record Collection
2:16:24 While loops
2:20:16 For loops
2:24:05 Iterate odd numbers with a for loop
2:27:01 Count backwards with a for loop
2:28:50 Iterate through an array with a for loop
2:31:46 Nesting for loops
2:36:03 Do...while loop
2:38:31 Recursion
2:42:12 Profile lookup
2:48:10 Generate random fractions
2:48:59 Generate random whole numbers
2:51:24 Generate random whole numbers within a range
2:55:05 parseInt
2:55:58 parseInt with a radix
2:58:24 Conditional ternary operator
3:00:35 Multiple ternary operators
3:02:42 Recursion for countdown
3:06:12 Recursion for range of numbers
3:08:54 Outro
-------------------------------------------------------------------
Music by Silent Partner
-------------------------------------------------------------------
Комментарии