filmov
tv
C++ file handling for beginners! The easiest way to read/write into text files!
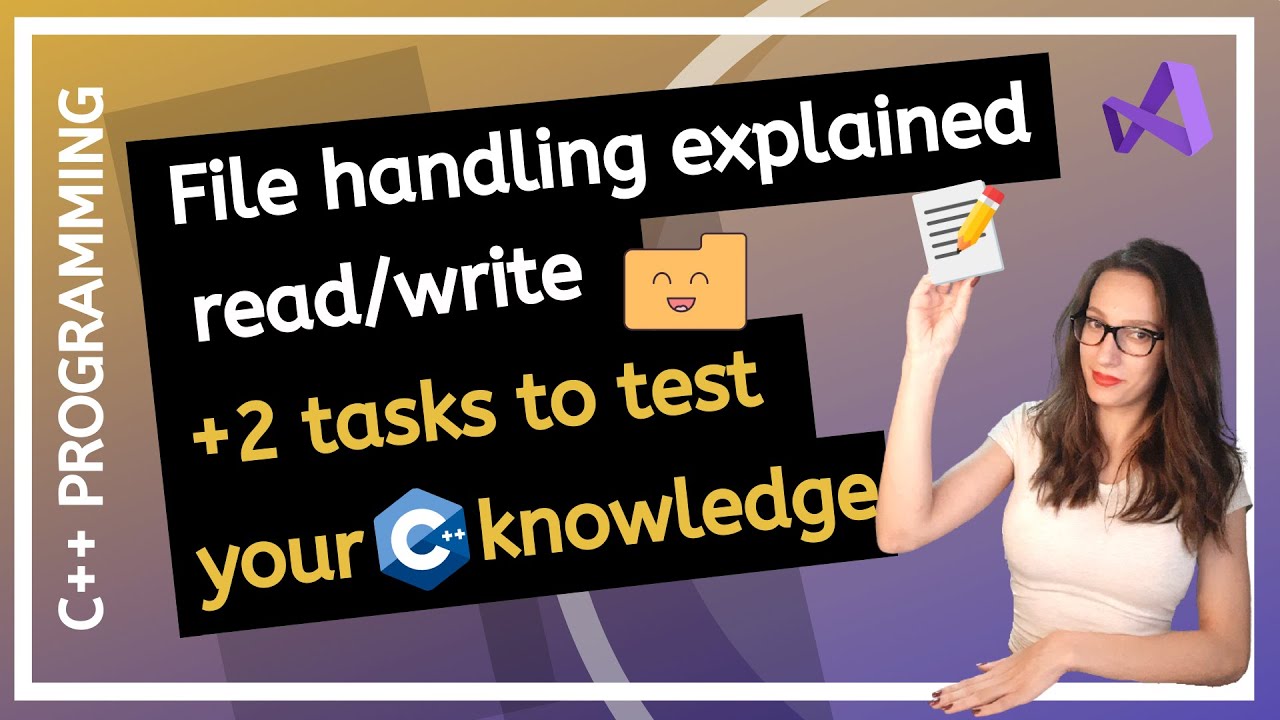
Показать описание
📚 Learn how to solve problems and build projects with these Free E-Books ⬇️
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
I use it to enhance the performance, features, and support for C, C#, and C++ development in Visual Studio.
It is a powerful, secure text editor designed specifically for programmers.
Files are used to store data permanently.
In this video, you'll learn how to read and write into text files using C++.
In order to work with files, first, include the fstream library.
Inside this library there are three data types:
ofstream - used for writing into files
ifstream - used for reading from files
fstream - used for both read and write
In this video, I'm demonstrating the use of fstream object in file handling.
In order to open a file we use open() function.
This function receives two parameters: file name and file open mode.
The modes in which file can be opened are below:
ios::in - opens the file to read(default for ifstream)
ios::out - opens the file to write(default for ofstream)
ios::binary - opens the file in binary mode
ios::app- opens the file to append new info at the end
ios::ate - opens and moves the control to the end of the file
ios::trunc - removes the data in the existing file
ios::nocreate - opens the file only if it already exists
ios::noreplace - opens the file only if it doesn't already exist
We can also combine different modes using symbol |
For example:
However, please don't feel obligated to do so. I appreciate every one of you, and I will continue to share valuable content with you regardless of whether you choose to support me in this way. Thank you for being part of the Code Beauty community! ❤️😇
Contents:
00:00 - Intro
01:02 - Write into a text file using C++
07:46 - Append to a text file using C++
10:35 - Read from a text file using C++
15:56 - Tasks to test your C++ knowledge!
Add me on:
Experience the power of practical learning, gain career-ready skills, and start building real applications!
This is a step-by-step course designed to take you from beginner to expert in no time!
💰 Here is a coupon to save 10% on your first payment (CODEBEAUTY_YT10).
Use it quickly, because it will be available for a limited time.
I use it to enhance the performance, features, and support for C, C#, and C++ development in Visual Studio.
It is a powerful, secure text editor designed specifically for programmers.
Files are used to store data permanently.
In this video, you'll learn how to read and write into text files using C++.
In order to work with files, first, include the fstream library.
Inside this library there are three data types:
ofstream - used for writing into files
ifstream - used for reading from files
fstream - used for both read and write
In this video, I'm demonstrating the use of fstream object in file handling.
In order to open a file we use open() function.
This function receives two parameters: file name and file open mode.
The modes in which file can be opened are below:
ios::in - opens the file to read(default for ifstream)
ios::out - opens the file to write(default for ofstream)
ios::binary - opens the file in binary mode
ios::app- opens the file to append new info at the end
ios::ate - opens and moves the control to the end of the file
ios::trunc - removes the data in the existing file
ios::nocreate - opens the file only if it already exists
ios::noreplace - opens the file only if it doesn't already exist
We can also combine different modes using symbol |
For example:
However, please don't feel obligated to do so. I appreciate every one of you, and I will continue to share valuable content with you regardless of whether you choose to support me in this way. Thank you for being part of the Code Beauty community! ❤️😇
Contents:
00:00 - Intro
01:02 - Write into a text file using C++
07:46 - Append to a text file using C++
10:35 - Read from a text file using C++
15:56 - Tasks to test your C++ knowledge!
Add me on:
Комментарии