filmov
tv
Majority Element II - Leetcode 229 - Python
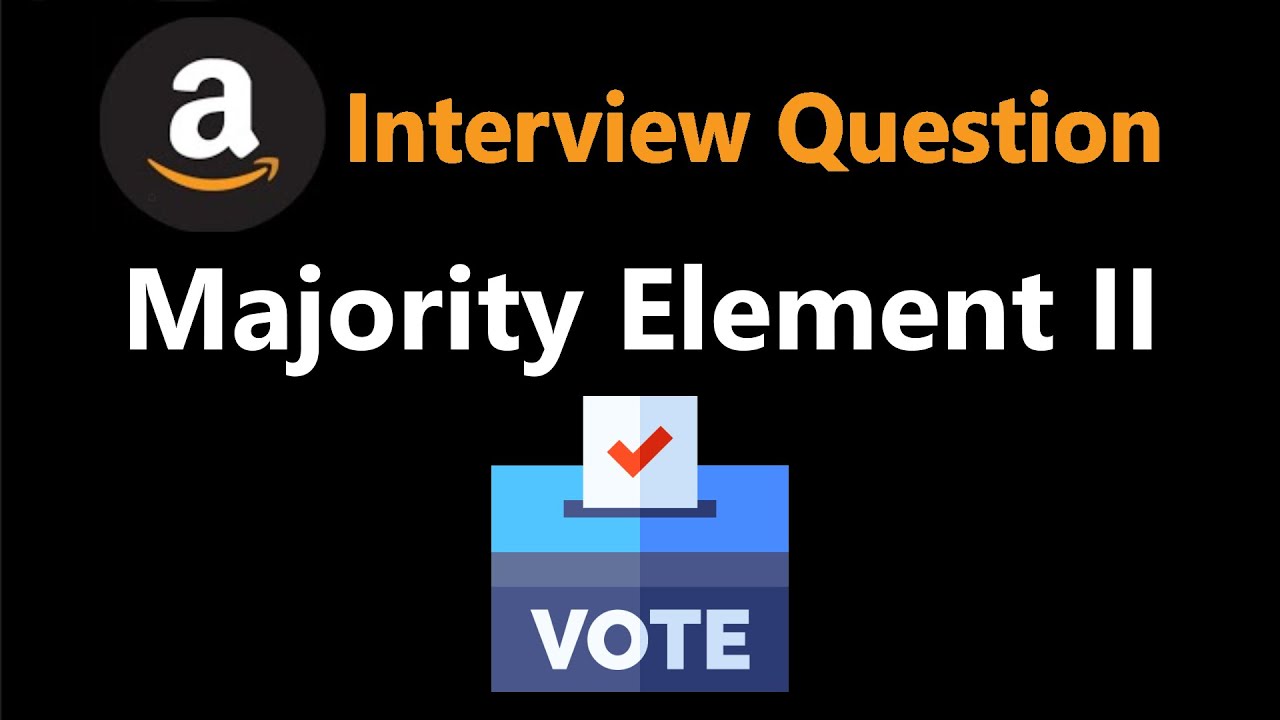
Показать описание
0:00 - Read the problem
0:30 - Drawing Explanation
10:05 - Coding Explanation
leetcode 229
#neetcode #leetcode #python
Majority Element II - Leetcode 229 - Python
Leetcode - Majority Element II (Python)
Majority Element II | Brute-Better-Optimal
Leetcode 229 - Majority Element II (Moore Voting Algorithm)
Majority Element II - LeetCode 229 - Python - Boyer Moore
Majority Element - Leetcode 169
229. Majority Element II - Day 5/31 Leetcode October Challenge
Majority Element II Leetcode || Leetcode Daily Challenge
Majority Element I | Majority Element II | Boyer-Moore | Made Simple | Leetcode 229 | Leetcode 169
Majority Element II | LeetCode 229 | Majority Element (Greater than N/3 Times)
Majority Element II - LeetCode #229 | Python, JavaScript, Java, C++
Majority element II (LeetCode 229: Explanation + Code)
Majority Element I | Brute-Better-Optimal | Moore's Voting Algorithm | Intuition 🔥|Brute to Opt...
Majority Element II
Majority Element II | Leet code 229 | Theory explained + Python code
Majority element II - LeetCode 229 problem - Java - Guide step by step
Majority Element II Leetcode 229
Majority Element 2 and 1 || Intuition + Example + Code || Find elements occuring more than n/3 times
LeetCode 229 : Majority Element II || MEDIUM || JAVA || Detailed Solution
Majority Element (LeetCode 169) | Full solution with 4 different methods | Interview Essential
Majority Element II | GOOGLE Interview Question | Brute-Better-Optimal
LeetCode 229 Majority Element II - HashMap Solution
Majority Element II | Решение на Python | LeetCode 229
229. Majority Element II - Leetcode Question Walkthrough
Комментарии