filmov
tv
C++ Tutorial 18 - Simple Snake Game (Part 1)
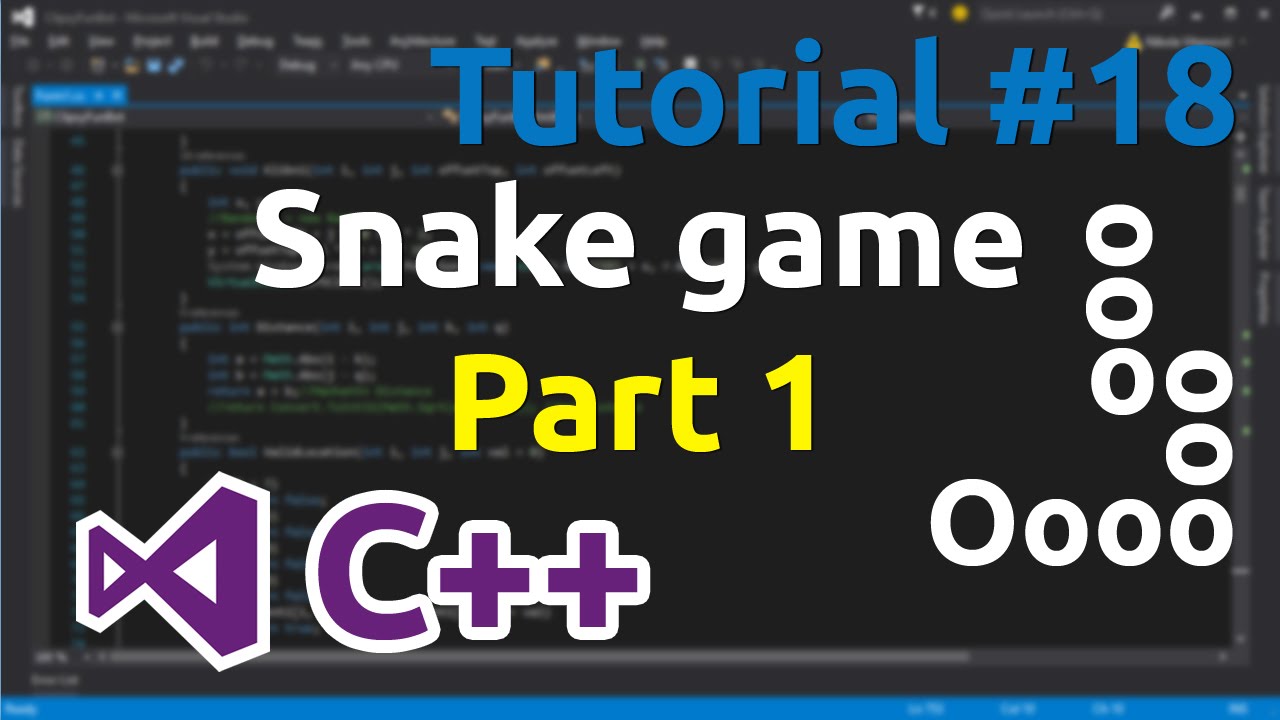
Показать описание
C++ Tutorial 18 - Simple Snake Game (Part 1)
Welcome to my simple game tutorial on C++. In this tutorial i will going to show you how to make a fun snake game.
We will structure the code of the game and do some basic drawing in the console.
Source code:
Download link for visual studio 2012 express:
Great C++ books that I recommend for beginners:
C++ Without Fear: A Beginner's Guide That Makes You Feel Smart (3rd Edition)
C++ Primer Plus (6th Edition) (Developer's Library)
Programming: Principles and Practice Using C++ (2nd Edition)
If you have any questions I'll be glad to answer, please leave a comment on the video.
Thanks for watching and please subscribe.
Welcome to my simple game tutorial on C++. In this tutorial i will going to show you how to make a fun snake game.
We will structure the code of the game and do some basic drawing in the console.
Source code:
Download link for visual studio 2012 express:
Great C++ books that I recommend for beginners:
C++ Without Fear: A Beginner's Guide That Makes You Feel Smart (3rd Edition)
C++ Primer Plus (6th Edition) (Developer's Library)
Programming: Principles and Practice Using C++ (2nd Edition)
If you have any questions I'll be glad to answer, please leave a comment on the video.
Thanks for watching and please subscribe.
C++ Tutorial 18 - Simple Snake Game (Part 1)
C Programming Tutorial for Beginners 18 - Functions in C
Programmieren in C Tutorial #18 - Eigene Header Datei erstellen
C tutorial for beginners 🕹️
C_18 Operators in C - Part 6 | Bitwise Operators | C Programming Tutorials
C Language Tutorial for Beginners (with Notes & Practice Questions)
C Programming Tutorial for Beginners 10 - If statement in C programming with example
C++ Tutorial for Beginners 6 - If and Else Statements
ROSÉ & Bruno Mars - APT. | Easy Piano Tutorial
C++ tutorial for beginners 👨💻
C Am F G - Piano Tutorial Very Easy - How To Play C Am F G
C Programming Tutorial | Learn C programming | C language
HOW TO Remove any Object | Davinci Resolve 18 Tutorial
C Programming Tutorial - 19 - if else
C++ Tutorial for Beginners 18 - C++ Pointers
How to Change Android Control Panel to iOS Control Center (Easy Tutorial)
C# Tutorial Deutsch - 4 Stunden Kurs zum C# lernen
C++ Tutorial for Beginners - Full Course
C Programming Tutorial 8 Pre and Post Increment
C# Tutorial For Beginners - Learn C# Basics in 1 Hour
C Programming Tutorial for Beginners 1 - Introduction to the C programming
C++ Tutorial for Beginners 18 - Arrays in C++
C++ Tutorial : C++ Full Course
C Programming Tutorial for Beginners 2 - First C Program | C Hello World! Example
Комментарии