filmov
tv
OrderedDict Vs Dictionary | Python 4 You | Lecture 272
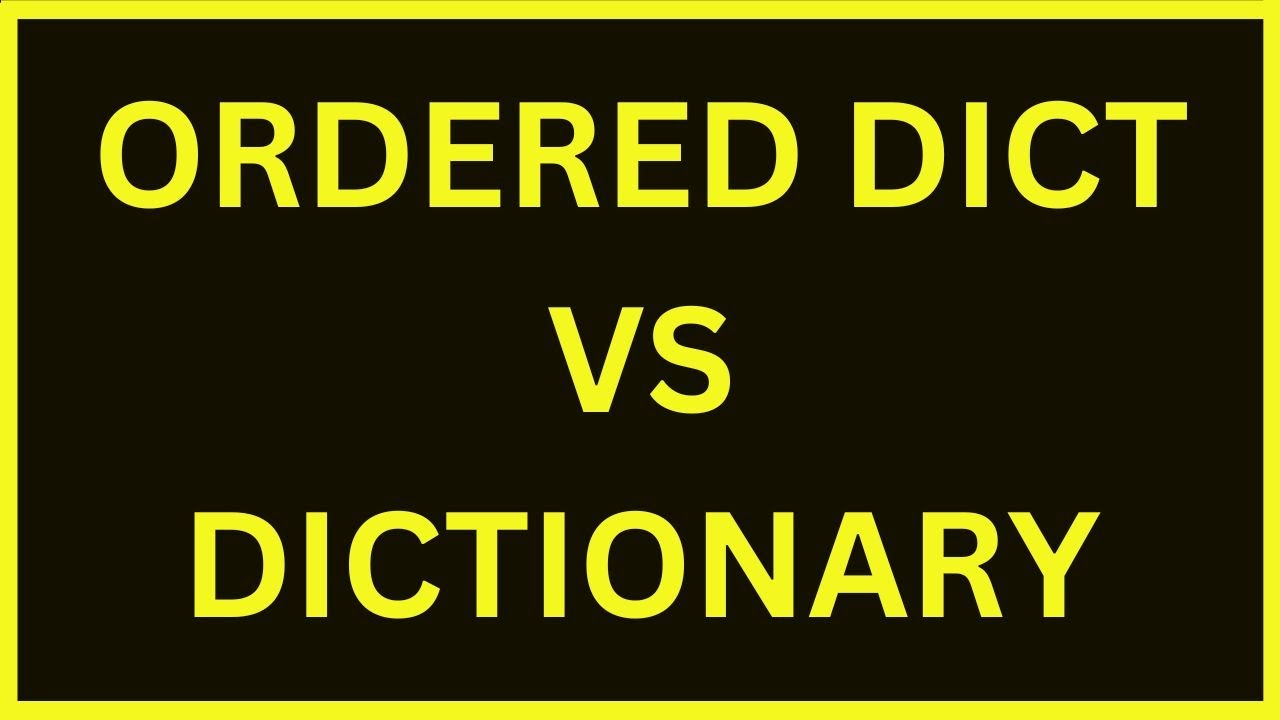
Показать описание
Decoding Python's Data Structures: Unraveling the Differences Between OrderedDict and dict
Introduction:
In the Python programming universe, dictionaries (dict) and ordered dictionaries (OrderedDict) are essential data structures, each serving a unique purpose. As developers, understanding the distinctions between these two structures is crucial for efficient and effective programming. This article aims to demystify the differences between OrderedDict and dict, shedding light on when to choose one over the other and how each can impact your code.
Basics of dict and OrderedDict:
dict (Dictionary):
The standard Python dictionary (dict) is an unordered collection of key-value pairs.
Introduced in Python 1.4, dictionaries provide a versatile way to store and retrieve data through a unique key.
OrderedDict:
OrderedDict is a subclass of dict introduced in Python 2.7, explicitly designed to maintain the order of key-value pairs based on insertion order.
It preserves the sequence in which elements are added, offering a predictable order during iteration.
Understanding the Differences:
Order Preservation:
The primary distinction lies in how these structures handle order. In a dict, the order of elements is not guaranteed, and iterating over a dictionary may yield results in an arbitrary order. On the other hand, OrderedDict ensures that the order of elements reflects the sequence in which they were added.
Performance Implications:
OrderedDicts, by nature of preserving order, may have a slightly higher memory overhead and slower insertions compared to dicts. However, the impact on performance is generally negligible for small to moderately sized datasets.
Use Cases:
Dictionaries (dict) are suitable for scenarios where order is not important, and quick access to values based on keys is the primary requirement. They are efficient for tasks like lookups and data retrieval.
OrderedDicts are preferred when the order of elements is significant, such as maintaining the sequence of configuration parameters or ensuring consistent serialization to formats like JSON.
Python Version Compatibility:
The standard dict is available in all Python versions, making it a universal choice. OrderedDict, while introduced in Python 2.7, is available in Python 3 as well. Developers working on legacy systems or with older Python versions may find OrderedDict a valuable addition.
Practical Examples:
Using dict:
python code
my_dict = {'apple': 3, 'banana': 2, 'orange': 1}
print(my_dict)
# Output: {'apple': 3, 'banana': 2, 'orange': 1}
Using OrderedDict:
python code
from collections import OrderedDict
my_ordered_dict = OrderedDict([('apple', 3), ('banana', 2), ('orange', 1)])
print(my_ordered_dict)
# Output: OrderedDict([('apple', 3), ('banana', 2), ('orange', 1)])
Conclusion:
In the vast Python ecosystem, the choice between dict and OrderedDict depends on the specific requirements of your project. If the order of elements is critical, especially in scenarios like configuration management or serialization, OrderedDict is the go-to option. However, for general-purpose tasks where order is not a concern, the standard dict offers simplicity and efficiency.
As you navigate the world of Python programming, understanding the nuances of these data structures empowers you to make informed decisions, ensuring that your code is not just functional but also optimized for performance and readability. Whether you opt for the flexibility of dict or the ordered precision of OrderedDict, each structure has its place in the Python developer's toolkit.
People also search for these keywords:
python dictionary, difference between list set tuple and dictionary in python, python dictionary append, python dictionary get, dictionary, python dictionary methods, what is difference between list set tuple and dictionary, python dictionary keys, python dict to json, python dict update, python dict, python dictionary comprehension, python dict keys, python ordered dictionary, python dict comprehension, python lists and dictionaries, python dict pop
Useful Queries:
Difference between ordered dictionary and dictionary w3schools
Difference between ordered dictionary and dictionary in python
python ordered dictionary by key
ordered dictionary python
ordered dictionary c#
sort ordered dictionary python
ordereddict example
python ordereddict methods
#python4 #pythontutorial #pythonprogramming #python3 #viral #trending #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.
Introduction:
In the Python programming universe, dictionaries (dict) and ordered dictionaries (OrderedDict) are essential data structures, each serving a unique purpose. As developers, understanding the distinctions between these two structures is crucial for efficient and effective programming. This article aims to demystify the differences between OrderedDict and dict, shedding light on when to choose one over the other and how each can impact your code.
Basics of dict and OrderedDict:
dict (Dictionary):
The standard Python dictionary (dict) is an unordered collection of key-value pairs.
Introduced in Python 1.4, dictionaries provide a versatile way to store and retrieve data through a unique key.
OrderedDict:
OrderedDict is a subclass of dict introduced in Python 2.7, explicitly designed to maintain the order of key-value pairs based on insertion order.
It preserves the sequence in which elements are added, offering a predictable order during iteration.
Understanding the Differences:
Order Preservation:
The primary distinction lies in how these structures handle order. In a dict, the order of elements is not guaranteed, and iterating over a dictionary may yield results in an arbitrary order. On the other hand, OrderedDict ensures that the order of elements reflects the sequence in which they were added.
Performance Implications:
OrderedDicts, by nature of preserving order, may have a slightly higher memory overhead and slower insertions compared to dicts. However, the impact on performance is generally negligible for small to moderately sized datasets.
Use Cases:
Dictionaries (dict) are suitable for scenarios where order is not important, and quick access to values based on keys is the primary requirement. They are efficient for tasks like lookups and data retrieval.
OrderedDicts are preferred when the order of elements is significant, such as maintaining the sequence of configuration parameters or ensuring consistent serialization to formats like JSON.
Python Version Compatibility:
The standard dict is available in all Python versions, making it a universal choice. OrderedDict, while introduced in Python 2.7, is available in Python 3 as well. Developers working on legacy systems or with older Python versions may find OrderedDict a valuable addition.
Practical Examples:
Using dict:
python code
my_dict = {'apple': 3, 'banana': 2, 'orange': 1}
print(my_dict)
# Output: {'apple': 3, 'banana': 2, 'orange': 1}
Using OrderedDict:
python code
from collections import OrderedDict
my_ordered_dict = OrderedDict([('apple', 3), ('banana', 2), ('orange', 1)])
print(my_ordered_dict)
# Output: OrderedDict([('apple', 3), ('banana', 2), ('orange', 1)])
Conclusion:
In the vast Python ecosystem, the choice between dict and OrderedDict depends on the specific requirements of your project. If the order of elements is critical, especially in scenarios like configuration management or serialization, OrderedDict is the go-to option. However, for general-purpose tasks where order is not a concern, the standard dict offers simplicity and efficiency.
As you navigate the world of Python programming, understanding the nuances of these data structures empowers you to make informed decisions, ensuring that your code is not just functional but also optimized for performance and readability. Whether you opt for the flexibility of dict or the ordered precision of OrderedDict, each structure has its place in the Python developer's toolkit.
People also search for these keywords:
python dictionary, difference between list set tuple and dictionary in python, python dictionary append, python dictionary get, dictionary, python dictionary methods, what is difference between list set tuple and dictionary, python dictionary keys, python dict to json, python dict update, python dict, python dictionary comprehension, python dict keys, python ordered dictionary, python dict comprehension, python lists and dictionaries, python dict pop
Useful Queries:
Difference between ordered dictionary and dictionary w3schools
Difference between ordered dictionary and dictionary in python
python ordered dictionary by key
ordered dictionary python
ordered dictionary c#
sort ordered dictionary python
ordereddict example
python ordereddict methods
#python4 #pythontutorial #pythonprogramming #python3 #viral #trending #pythonforbeginners #pythonlectures #pythonprograms #pythonlatest #rehanblogger #python4you #pythonlatestversion #pythonlatestversion Learn python3.12.0 and latest version of python3.13. If you are searching for python3.13.0 lessons, you are at the right place as this course will be very helpful for python learners or python beginners.