filmov
tv
Unity Tutorial: Roll A Ball - Part 2: Player & Camera Movement
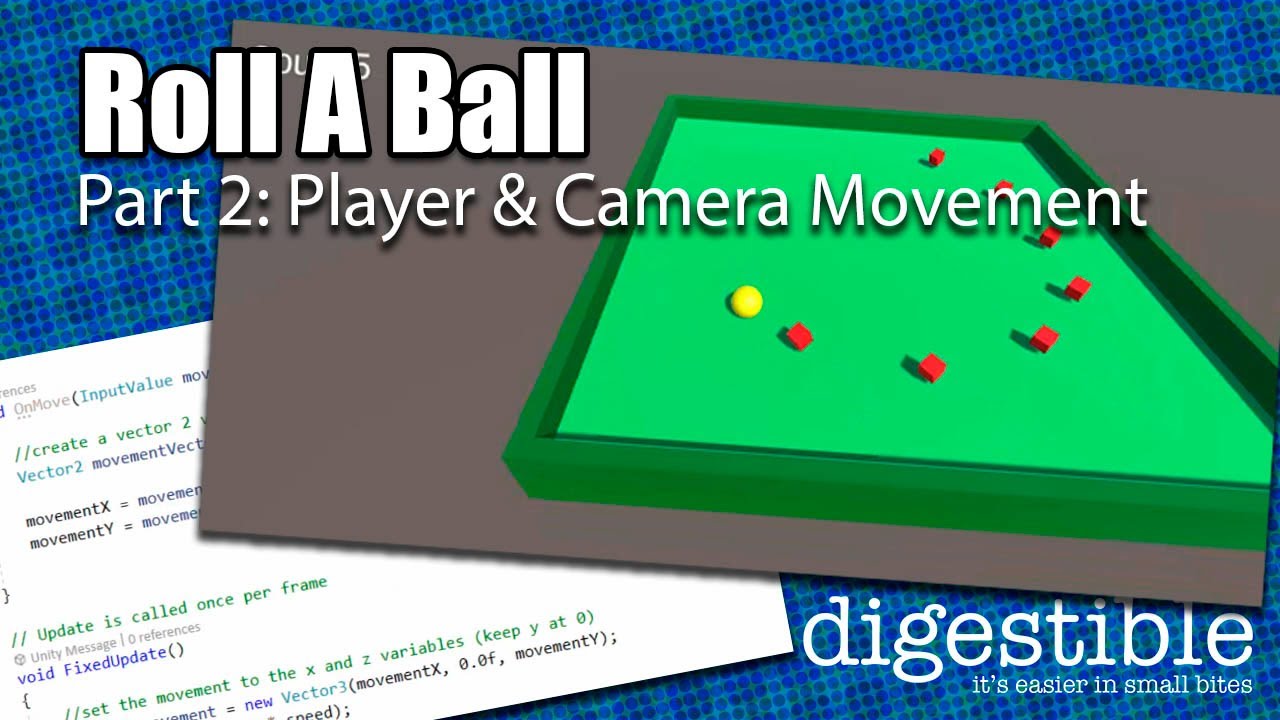
Показать описание
This tutorial is based off of Unity's classic "Roll A Ball" but it is in a shorter format so you can learn the same concepts in less time. It shows how to build a rolling ball game from scratch in Unity and C#. Concepts include player movement, Unity's new Input System, Collisions, Scoring, and UI with TextMeshPro text elements.
The code and concept comes from Unity's site. I am just presenting it a little differently
The code and concept comes from Unity's site. I am just presenting it a little differently
Unity Tutorial: Roll A Ball - part 1: Playfield Setup (Make your first Unity Game!)
Unity Tutorial: Roll A Ball - Part 2: Player & Camera Movement
Roll-a-Ball: 1.1 Create a new Unity project
Make an Easy 3D Game, Roll a Damn Ball in Unity
ROLL A BALL CREATE BY UNITY
Unity Roll-A-Ball Tutorial 2020 - Player Control / Player Movement Fix
Make a Roll a Ball Game in UNITY Pt 1
Unity: Roll a Ball Tutorial 1 of 8 Setting up the Game Unity Official Tutorials
Xtreme Roll a Ball! - Part 1: Setup Unity Project
Unity Tutorial - Roll A Ball Implementation
Ball Movement for a Roll a Ball Type of Game - Unity Visual Scripting Tutorial
My Curious Experience with the Unity Roll-A-Ball Tutorial (& Tweaks I made in the process)
Unity: Roll a Ball Tutorial 4 of 8 Setting up the Play Area Unity Official Tutorials
Roll A Ball Game (Unity + WebGL)
Roll-A-Ball In Unity - Under 2 Minutes | Beginners
Unity Tutorial: Roll A Ball - Part 4: Scoring & UI
How to roll a ball using Playmaker in Unity
Count and Display Scores | Advancing Roll-a-Ball | Unity Tutorial
Setting up the game 01 Roll a ball Unity Official Tutorials
Unity: Roll a Ball Tutorial 8 of 8 Building the Game Unity Official Tutorials
Unity game project | Roll-a-ball 3D
Unity - Roll-a-ball (Unity Tutorial)
'Roll-a-ball' Con 'Unity Learn'
Tweak on the Roll a Ball Unity game
Комментарии