filmov
tv
Solving the HTML5 For Loop Issue with Flask and Python
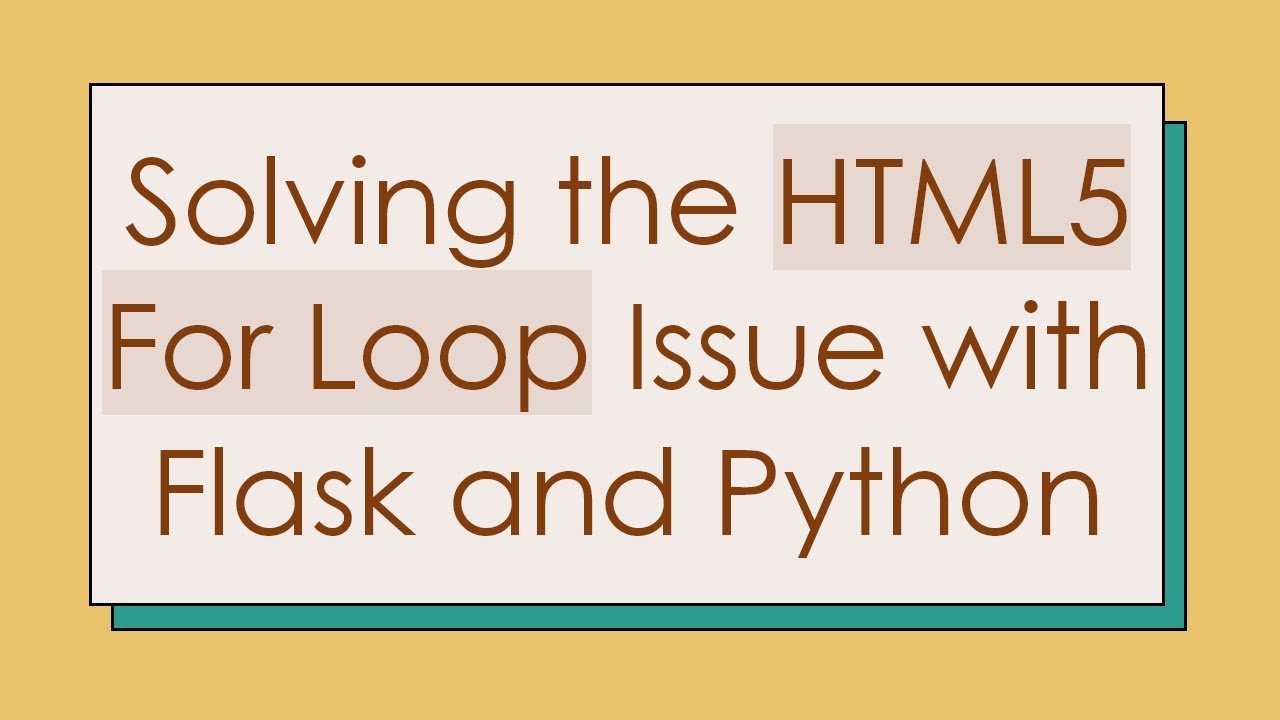
Показать описание
Discover the solution to the common issue of displaying images in Flask using an HTML5 for loop. We break down the correct method to iterate through lists of tuples and correctly render images in your web application.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Problem using HTML5 For Loop with flask and Python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Problem: Displaying Images with Flask
When working with Flask and HTML, one common challenge developers face is effectively iterating through lists of data to display images dynamically. This issue might arise if you are using a for loop in your HTML5 template to cycle through items, such as tuples containing file paths to images. Many developers struggle with rendering images correctly, even when providing accurate paths manually.
In this guide, we’ll review a specific issue you might encounter when trying to iterate through a list of tuples in a Flask application and how to resolve it effectively.
The Challenge
The developer in question was trying to output images stored in tuples through a for loop in their HTML file. Here’s the example list they were working with:
[[See Video to Reveal this Text or Code Snippet]]
When trying to render the images using a for loop, the image paths were not being displayed correctly even though they were valid. The HTML code snippet that the developer used looked similar to this:
[[See Video to Reveal this Text or Code Snippet]]
This approach led to problems since it attempted to nest the Jinja template syntax inappropriately.
The Solution
To correct the issue and ensure that images display as intended, the developer found a simple solution. Instead of embedding the template syntax for the filename within another set of braces, they modified it as follows:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Correction
Understanding url_for
url_for is a built-in Flask function that generates the URL for a specific endpoint. In the context of rendering static images, it constructs the correct path based on the filename you provide. This method ensures that the filename points correctly to the static directory of your Flask application.
What Changed in the Code?
Removing Extra Braces: The nested braces were causing issues in rendering. By directly passing item[0] to url_for, you get the correct URL without extra syntax errors.
Simplifying Syntax: It makes the code cleaner and easier to read, resulting in better maintenance and fewer bugs.
Conclusion
By following the correct method to render your images within a Flask application, you can easily avoid the common pitfalls related to HTML5 for loops. Always ensure that you are using clean, simple expressions with Flask’s url_for function to manage your static files effectively.
Take this knowledge and apply it to your Flask projects, and you’ll find working with dynamic images in your applications is much easier! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Problem using HTML5 For Loop with flask and Python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Problem: Displaying Images with Flask
When working with Flask and HTML, one common challenge developers face is effectively iterating through lists of data to display images dynamically. This issue might arise if you are using a for loop in your HTML5 template to cycle through items, such as tuples containing file paths to images. Many developers struggle with rendering images correctly, even when providing accurate paths manually.
In this guide, we’ll review a specific issue you might encounter when trying to iterate through a list of tuples in a Flask application and how to resolve it effectively.
The Challenge
The developer in question was trying to output images stored in tuples through a for loop in their HTML file. Here’s the example list they were working with:
[[See Video to Reveal this Text or Code Snippet]]
When trying to render the images using a for loop, the image paths were not being displayed correctly even though they were valid. The HTML code snippet that the developer used looked similar to this:
[[See Video to Reveal this Text or Code Snippet]]
This approach led to problems since it attempted to nest the Jinja template syntax inappropriately.
The Solution
To correct the issue and ensure that images display as intended, the developer found a simple solution. Instead of embedding the template syntax for the filename within another set of braces, they modified it as follows:
[[See Video to Reveal this Text or Code Snippet]]
Breaking Down the Correction
Understanding url_for
url_for is a built-in Flask function that generates the URL for a specific endpoint. In the context of rendering static images, it constructs the correct path based on the filename you provide. This method ensures that the filename points correctly to the static directory of your Flask application.
What Changed in the Code?
Removing Extra Braces: The nested braces were causing issues in rendering. By directly passing item[0] to url_for, you get the correct URL without extra syntax errors.
Simplifying Syntax: It makes the code cleaner and easier to read, resulting in better maintenance and fewer bugs.
Conclusion
By following the correct method to render your images within a Flask application, you can easily avoid the common pitfalls related to HTML5 for loops. Always ensure that you are using clean, simple expressions with Flask’s url_for function to manage your static files effectively.
Take this knowledge and apply it to your Flask projects, and you’ll find working with dynamic images in your applications is much easier! Happy coding!