filmov
tv
Java Fundamentals – Strings, Enums, Reference Casting, and Type Casting Explained Simply!
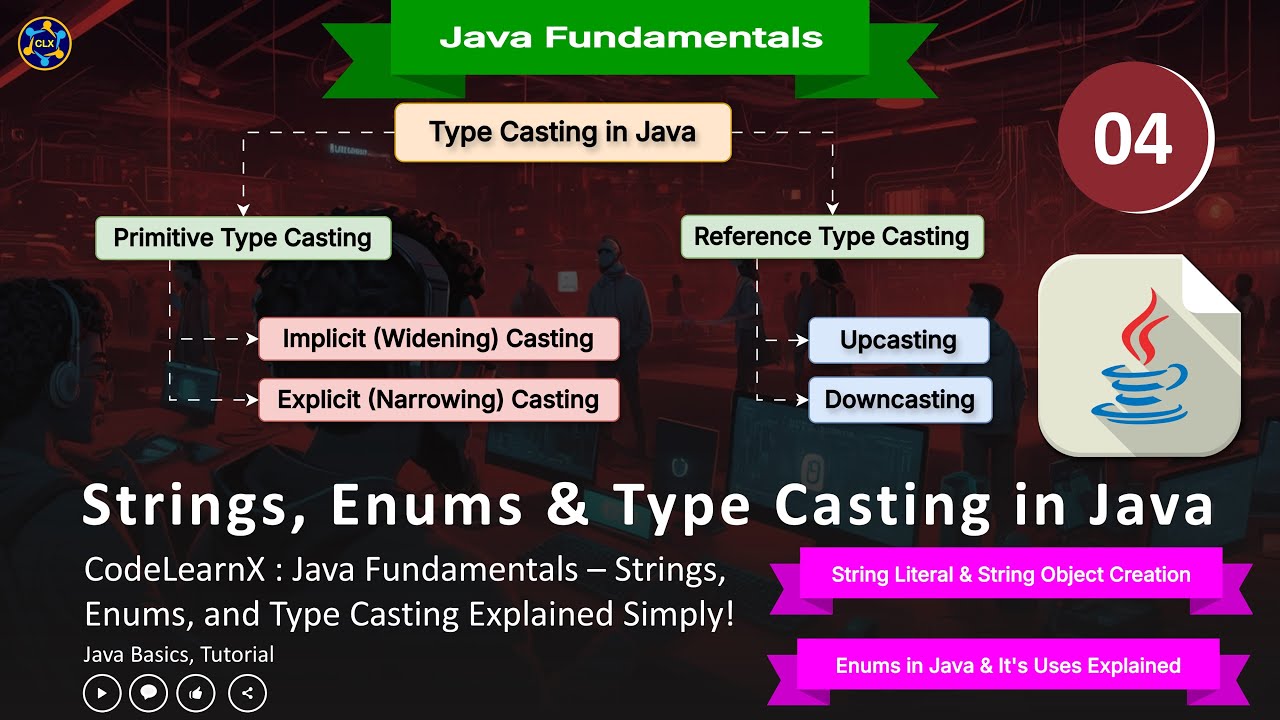
Показать описание
Welcome to this comprehensive tutorial on some of the most essential topics in Java programming—Strings, Enums, Type Casting, and Reference Casting. Whether you’re new to Java or just looking to deepen your understanding of these concepts, this video provides step-by-step explanations with practical examples to help you fully grasp these foundational topics. By the end of this tutorial, you'll be well-prepared to work with Strings, Enums, Type Casting, and Reference Casting in your own Java projects.
What You'll Learn in This Video:
In this video, we’ll walk through the following concepts in detail:
Java Strings: Strings are one of the most important data types in any programming language, and Java is no exception. We’ll start with a basic introduction to what Strings are and how to use them. You'll learn how to declare, initialize, and manipulate Strings effectively using Java’s built-in String class. Java provides a wealth of methods to work with Strings, such as .length(), .substring(), .charAt(), and .indexOf().
We’ll also explore some advanced string manipulation techniques, such as string concatenation, string comparison (.equals() vs ==), and how Strings are immutable in Java. We will discuss how this immutability impacts memory management and performance, and why Java Strings are stored in a special memory area known as the String Pool.
Enums in Java: Enums (short for Enumerations) are a special type in Java used to represent fixed sets of constants. If you’ve ever needed to define a group of related constants—like days of the week, directions, or status codes—Enums are the perfect tool.
Type Casting in Java: Type casting is a technique that allows you to convert one data type into another, either explicitly or implicitly. In this section, you’ll understand the difference between implicit (widening) and explicit (narrowing) type casting.
Implicit Type Casting occurs when you assign a smaller data type (like int) to a larger data type (like double). Java automatically handles this type of casting without any additional code because there’s no risk of data loss.
Explicit Type Casting occurs when you need to convert a larger data type to a smaller one, such as converting a double to an int. This kind of conversion requires you to manually specify the target type because there’s potential for data loss, and Java wants you to be aware of it.
We’ll demonstrate these concepts with code examples to show when and how to apply type casting correctly. We’ll also discuss common pitfalls, such as data overflow and precision loss when performing narrowing conversions.
Reference Casting: In Java, there are two types of casting: primitive casting (which we discussed above) and reference casting. Reference casting involves converting one object reference to another. This is commonly seen when dealing with class hierarchies and inheritance. There are two types of reference casting: upcasting and downcasting.
Upcasting: This is when a subclass is cast to its superclass. Upcasting is always safe and does not require explicit casting. For example, casting a Dog object to an Animal reference is considered upcasting because Dog is a subclass of Animal.
Downcasting: This is when you cast a superclass reference back to a subclass reference. Unlike upcasting, downcasting requires explicit casting and should be done with caution, as it can throw a ClassCastException at runtime if the object being cast is not of the appropriate type.
--------------------------------------------------------------------
If you find this video valuable, give it a like.
If you know someone who needs to see it, share it.
If you have questions ask below in comment section.
----------------------------------------------------------------------
Why Watch This Video?
This video is designed for anyone who wants to improve their Java skills, whether you are:
A complete beginner learning Java for the first time
A student preparing for exams or coding interviews
A professional developer brushing up on Java fundamentals
Someone looking to expand your programming toolkit by learning how to work with Strings, Enums, Type Casting, and Reference Casting
We focus on making the material easy to follow with practical examples and clear explanations. If you want to deepen your understanding of Java and improve your programming skills, this video is for you!
Make sure you LIKE, SUBSCRIBE, COMMENT, and REQUEST A VIDEO! :)
-----------------------------------------------------------------------
Follow us on:-
-----------------------------------------------------------------------------
What You'll Learn in This Video:
In this video, we’ll walk through the following concepts in detail:
Java Strings: Strings are one of the most important data types in any programming language, and Java is no exception. We’ll start with a basic introduction to what Strings are and how to use them. You'll learn how to declare, initialize, and manipulate Strings effectively using Java’s built-in String class. Java provides a wealth of methods to work with Strings, such as .length(), .substring(), .charAt(), and .indexOf().
We’ll also explore some advanced string manipulation techniques, such as string concatenation, string comparison (.equals() vs ==), and how Strings are immutable in Java. We will discuss how this immutability impacts memory management and performance, and why Java Strings are stored in a special memory area known as the String Pool.
Enums in Java: Enums (short for Enumerations) are a special type in Java used to represent fixed sets of constants. If you’ve ever needed to define a group of related constants—like days of the week, directions, or status codes—Enums are the perfect tool.
Type Casting in Java: Type casting is a technique that allows you to convert one data type into another, either explicitly or implicitly. In this section, you’ll understand the difference between implicit (widening) and explicit (narrowing) type casting.
Implicit Type Casting occurs when you assign a smaller data type (like int) to a larger data type (like double). Java automatically handles this type of casting without any additional code because there’s no risk of data loss.
Explicit Type Casting occurs when you need to convert a larger data type to a smaller one, such as converting a double to an int. This kind of conversion requires you to manually specify the target type because there’s potential for data loss, and Java wants you to be aware of it.
We’ll demonstrate these concepts with code examples to show when and how to apply type casting correctly. We’ll also discuss common pitfalls, such as data overflow and precision loss when performing narrowing conversions.
Reference Casting: In Java, there are two types of casting: primitive casting (which we discussed above) and reference casting. Reference casting involves converting one object reference to another. This is commonly seen when dealing with class hierarchies and inheritance. There are two types of reference casting: upcasting and downcasting.
Upcasting: This is when a subclass is cast to its superclass. Upcasting is always safe and does not require explicit casting. For example, casting a Dog object to an Animal reference is considered upcasting because Dog is a subclass of Animal.
Downcasting: This is when you cast a superclass reference back to a subclass reference. Unlike upcasting, downcasting requires explicit casting and should be done with caution, as it can throw a ClassCastException at runtime if the object being cast is not of the appropriate type.
--------------------------------------------------------------------
If you find this video valuable, give it a like.
If you know someone who needs to see it, share it.
If you have questions ask below in comment section.
----------------------------------------------------------------------
Why Watch This Video?
This video is designed for anyone who wants to improve their Java skills, whether you are:
A complete beginner learning Java for the first time
A student preparing for exams or coding interviews
A professional developer brushing up on Java fundamentals
Someone looking to expand your programming toolkit by learning how to work with Strings, Enums, Type Casting, and Reference Casting
We focus on making the material easy to follow with practical examples and clear explanations. If you want to deepen your understanding of Java and improve your programming skills, this video is for you!
Make sure you LIKE, SUBSCRIBE, COMMENT, and REQUEST A VIDEO! :)
-----------------------------------------------------------------------
Follow us on:-
-----------------------------------------------------------------------------