filmov
tv
Mastering the Art of Importing Packages in Python
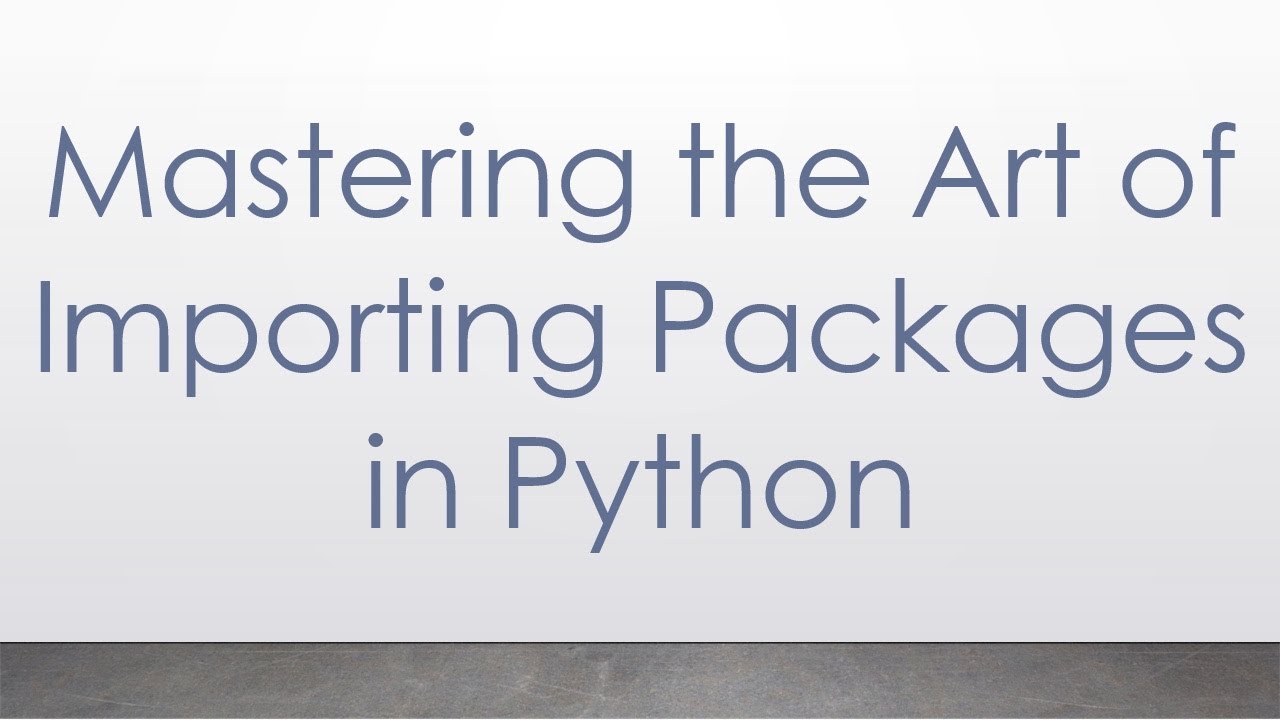
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to effectively import packages in Python to enhance your coding efficiency. Discover numerous techniques, tips, and best practices for utilizing Python packages.
---
Mastering the Art of Importing Packages in Python
When coding in Python, one of the most powerful features available to developers is the ability to import packages. Modules and packages allow you to leverage existing code, enhance productivity, and maintain clean, readable scripts. In this guide, we will explore various techniques and best practices for importing packages in Python.
Understanding Modules vs. Packages
In Python, a module is a single file (with the .py extension) that can be imported to add functionality to your projects. A package, on the other hand, is a collection of modules organized in directories that include an __init__.py file.
Importing Modules and Packages
Basic Imports
The simplest way to import a module or package is using the import statement:
[[See Video to Reveal this Text or Code Snippet]]
This example imports the entire math module and allows you to use its sqrt function.
Importing Specific Attributes
You can also import specific functions, classes, or variables from a module:
[[See Video to Reveal this Text or Code Snippet]]
This approach can make your code cleaner and more efficient by only bringing in what you need.
Renaming Imports
Sometimes, module names can be long or clash with other names in your code. In such cases, you can rename the imported module using the as keyword:
[[See Video to Reveal this Text or Code Snippet]]
Renaming can make your scripts easier to read and manage, particularly when working with popular libraries like NumPy or Pandas.
Importing All Attributes
While it’s possible to import all attributes from a module using the asterisk *, this method is generally discouraged due to potential namespace cluttering:
[[See Video to Reveal this Text or Code Snippet]]
Only use this approach if you are sure it won’t cause conflicts and if the module's namespace is well-understood.
Best Practices
Group and Order Imports
Group your imports logically. Standard library imports should come first, followed by third-party packages, and finally, local application imports:
[[See Video to Reveal this Text or Code Snippet]]
Avoid Circular Imports
Circular imports happen when two modules depend on each other. This can lead to errors and is best avoided. One way to manage this is by refactoring the interdependent code into a third module.
Use Virtual Environments
Virtual environments help manage package dependencies effectively. They create isolated environments for your Python projects, ensuring that each project can have its own dependencies, independent of the system-wide packages.
Use Absolute Imports
Python 3 strongly encourages the use of absolute imports (using full path) over relative imports:
[[See Video to Reveal this Text or Code Snippet]]
Absolute imports are more readable and reduce the risk of import misinterpretation.
Conclusion
Importing packages in Python is a fundamental skill that can greatly enhance your development process. By following best practices and understanding the various techniques, you can write more efficient, readable, and maintainable code. Armed with these insights, you're well-prepared to effectively utilize the rich ecosystem of Python packages in your projects.
---
Summary: Learn how to effectively import packages in Python to enhance your coding efficiency. Discover numerous techniques, tips, and best practices for utilizing Python packages.
---
Mastering the Art of Importing Packages in Python
When coding in Python, one of the most powerful features available to developers is the ability to import packages. Modules and packages allow you to leverage existing code, enhance productivity, and maintain clean, readable scripts. In this guide, we will explore various techniques and best practices for importing packages in Python.
Understanding Modules vs. Packages
In Python, a module is a single file (with the .py extension) that can be imported to add functionality to your projects. A package, on the other hand, is a collection of modules organized in directories that include an __init__.py file.
Importing Modules and Packages
Basic Imports
The simplest way to import a module or package is using the import statement:
[[See Video to Reveal this Text or Code Snippet]]
This example imports the entire math module and allows you to use its sqrt function.
Importing Specific Attributes
You can also import specific functions, classes, or variables from a module:
[[See Video to Reveal this Text or Code Snippet]]
This approach can make your code cleaner and more efficient by only bringing in what you need.
Renaming Imports
Sometimes, module names can be long or clash with other names in your code. In such cases, you can rename the imported module using the as keyword:
[[See Video to Reveal this Text or Code Snippet]]
Renaming can make your scripts easier to read and manage, particularly when working with popular libraries like NumPy or Pandas.
Importing All Attributes
While it’s possible to import all attributes from a module using the asterisk *, this method is generally discouraged due to potential namespace cluttering:
[[See Video to Reveal this Text or Code Snippet]]
Only use this approach if you are sure it won’t cause conflicts and if the module's namespace is well-understood.
Best Practices
Group and Order Imports
Group your imports logically. Standard library imports should come first, followed by third-party packages, and finally, local application imports:
[[See Video to Reveal this Text or Code Snippet]]
Avoid Circular Imports
Circular imports happen when two modules depend on each other. This can lead to errors and is best avoided. One way to manage this is by refactoring the interdependent code into a third module.
Use Virtual Environments
Virtual environments help manage package dependencies effectively. They create isolated environments for your Python projects, ensuring that each project can have its own dependencies, independent of the system-wide packages.
Use Absolute Imports
Python 3 strongly encourages the use of absolute imports (using full path) over relative imports:
[[See Video to Reveal this Text or Code Snippet]]
Absolute imports are more readable and reduce the risk of import misinterpretation.
Conclusion
Importing packages in Python is a fundamental skill that can greatly enhance your development process. By following best practices and understanding the various techniques, you can write more efficient, readable, and maintainable code. Armed with these insights, you're well-prepared to effectively utilize the rich ecosystem of Python packages in your projects.