filmov
tv
How to Refresh JWT Tokens Effectively in Python
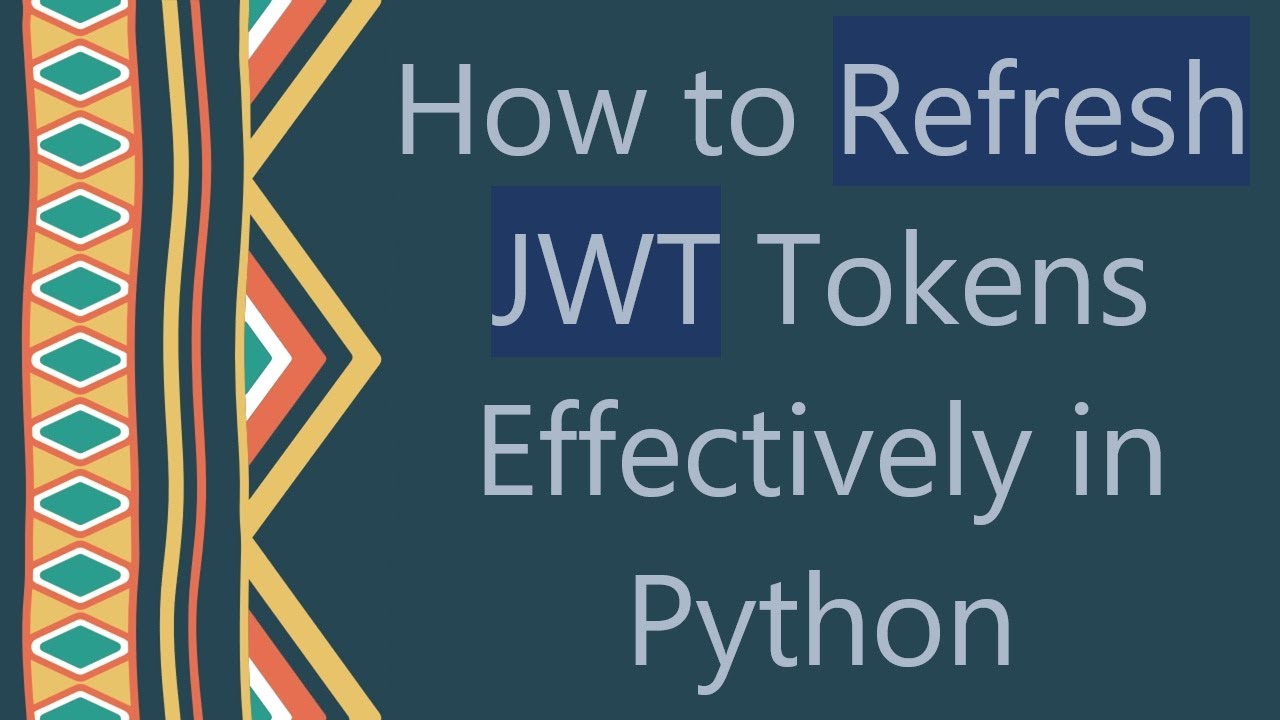
Показать описание
Summary: Learn how to refresh JWT tokens in Python, including step-by-step instructions on handling expired tokens using JWT refresh tokens. Improve your authentication flow in Python applications.
---
How to Refresh JWT Tokens Effectively in Python
In this guide, we will delve into how to refresh JWT (JSON Web Tokens) in Python applications. JWTs are widely used for authentication and authorization purposes in modern web applications. Understanding how to refresh JWT tokens, especially expired ones, is crucial for maintaining a seamless and secure user experience. Let's get started!
Understanding JWT and Refresh Tokens
JWT tokens are used to securely transmit information between parties. They are stateless and self-contained, often containing user identity information and claims. However, like all good things, JWT tokens have a lifespan. When a token expires, the user needs a new token to continue accessing protected resources. This is where JWT refresh tokens come into play.
A refresh token is a special token used to obtain a new JWT without requiring the user to log in again. By using refresh tokens, you can improve security and user experience by ensuring continuous access without frequent interruptions.
Handling Token Expiration
How to Refresh an Expired JWT Token
When a JWT token expires, you can use a refresh token to get a new token. Here is a typical flow in Python:
User Authentication: The user logs in and receives both a JWT token and a refresh token.
Token Validation: When the JWT token expires, the client sends the refresh token to the server to get a new JWT token.
Token Issuance: The server validates the refresh token and, if valid, issues a new JWT token.
Step-by-Step Guide
Step 1: Generate JWT and Refresh Tokens
When a user logs in, generate and send both tokens to the client:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Refreshing an Expired JWT Token
When the JWT token expires, the client sends a request with the refresh token to get a new JWT:
[[See Video to Reveal this Text or Code Snippet]]
Best Practices
Rotate Refresh Tokens: Issue a new refresh token whenever a new JWT token is generated. This way, even if an attacker gets hold of a refresh token, it will be invalidated soon.
Secure Storage: Store tokens securely in the client-side, preferably using secure HTTP-only cookies to prevent XSS attacks.
Use Short-Lived JWTs: Short-lived JWT tokens reduce the time window an attacker has in case of token theft.
Monitor & Revoke Tokens: Implement mechanisms to monitor and revoke tokens for compromised user accounts or when an unusual activity is detected.
By following these steps and best practices, you can securely manage JWT and refresh tokens in your Python applications, ensuring an uninterrupted and secure user experience.
Happy coding!
---
How to Refresh JWT Tokens Effectively in Python
In this guide, we will delve into how to refresh JWT (JSON Web Tokens) in Python applications. JWTs are widely used for authentication and authorization purposes in modern web applications. Understanding how to refresh JWT tokens, especially expired ones, is crucial for maintaining a seamless and secure user experience. Let's get started!
Understanding JWT and Refresh Tokens
JWT tokens are used to securely transmit information between parties. They are stateless and self-contained, often containing user identity information and claims. However, like all good things, JWT tokens have a lifespan. When a token expires, the user needs a new token to continue accessing protected resources. This is where JWT refresh tokens come into play.
A refresh token is a special token used to obtain a new JWT without requiring the user to log in again. By using refresh tokens, you can improve security and user experience by ensuring continuous access without frequent interruptions.
Handling Token Expiration
How to Refresh an Expired JWT Token
When a JWT token expires, you can use a refresh token to get a new token. Here is a typical flow in Python:
User Authentication: The user logs in and receives both a JWT token and a refresh token.
Token Validation: When the JWT token expires, the client sends the refresh token to the server to get a new JWT token.
Token Issuance: The server validates the refresh token and, if valid, issues a new JWT token.
Step-by-Step Guide
Step 1: Generate JWT and Refresh Tokens
When a user logs in, generate and send both tokens to the client:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Refreshing an Expired JWT Token
When the JWT token expires, the client sends a request with the refresh token to get a new JWT:
[[See Video to Reveal this Text or Code Snippet]]
Best Practices
Rotate Refresh Tokens: Issue a new refresh token whenever a new JWT token is generated. This way, even if an attacker gets hold of a refresh token, it will be invalidated soon.
Secure Storage: Store tokens securely in the client-side, preferably using secure HTTP-only cookies to prevent XSS attacks.
Use Short-Lived JWTs: Short-lived JWT tokens reduce the time window an attacker has in case of token theft.
Monitor & Revoke Tokens: Implement mechanisms to monitor and revoke tokens for compromised user accounts or when an unusual activity is detected.
By following these steps and best practices, you can securely manage JWT and refresh tokens in your Python applications, ensuring an uninterrupted and secure user experience.
Happy coding!