filmov
tv
Understanding When to Use strings, integers, and floats in Python
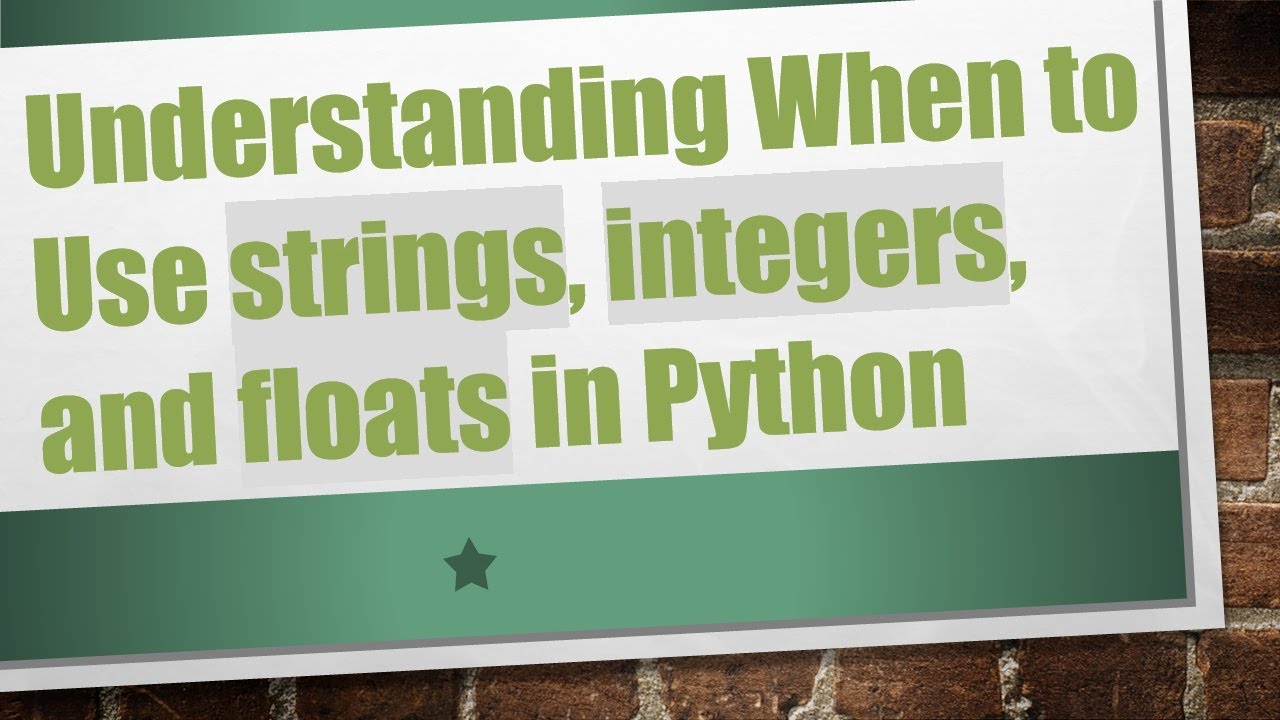
Показать описание
Learn how to effectively use `strings`, `integers`, and `floats` in Python, and understand when to change data types for better programming practices.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to use strings, integers, and float
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding When to Use strings, integers, and floats in Python
When programming in Python, one of the fundamental concepts you’ll encounter is the use of data types—specifically strings, integers, and floats. Understanding when and how to use these data types can greatly enhance your coding efficiency and make your code more readable and logical. In this guide, we’ll explore each of these data types, discuss when to use them, and clarify some common points of confusion.
What are Data Types?
Data types in programming are classifications that dictate what kind of data can be stored and manipulated within a program. Python features several data types, but for this discussion, we will focus on:
Strings (str): Used for representing textual data.
Integers (int): Used for whole numbers.
Floats (float): Used for numbers with decimal points.
When to Use Each Data Type
Strings
Strings should be used when you need to represent data that contains characters—whether they are alphabetic or symbolic. Here are some situations where strings are most appropriate:
Names: e.g., "Alice", "Bob".
Addresses: e.g., "123 Main St, Springfield".
Character Constants: While enums are an option, strings can be handy for straightforward constants.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Integers
Use integers when you require whole number values. Integers are ideal for:
Counters: e.g., counting the number of iterations in a loop.
Indexes: Used for accessing positions within lists.
Phone Numbers: Generally, opt for strings here if you don’t need arithmetic operations.
Important Note: If you need to carry out arithmetic operations like addition, subtraction, multiplication, or division, you must use integers rather than strings.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Floats
Floats come into play when you need to represent numbers that require decimal precision. This is especially useful for:
Financial Data: Like salaries or account balances, e.g., 49.99 (dollars) or 1000.50 (cents).
Measurements: Such as height, weight, or distance, e.g., 5.2 meters.
When working with decimals, always use floats to ensure the integrity of the values.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Changing Data Types
There might be scenarios where you need to change from one data type to another while working in Python. This is often necessary to carry out specific operations or to meet the requirements of a function. For example, if you have a numeric string and you want to perform an addition, you will need to convert it to an integer or float.
Example of converting a string to an integer:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the proper usage of strings, integers, and floats in Python is essential for effective programming. By following the guidelines outlined in this post, you can make more informed decisions about which data type to use in different situations. Remember, always choose the data type that best fits your needs based on the nature of the data you are working with.
With practice, you'll find that managing and utilizing these data types will become second nature and will lead you to write more efficient and effective Python code. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to use strings, integers, and float
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding When to Use strings, integers, and floats in Python
When programming in Python, one of the fundamental concepts you’ll encounter is the use of data types—specifically strings, integers, and floats. Understanding when and how to use these data types can greatly enhance your coding efficiency and make your code more readable and logical. In this guide, we’ll explore each of these data types, discuss when to use them, and clarify some common points of confusion.
What are Data Types?
Data types in programming are classifications that dictate what kind of data can be stored and manipulated within a program. Python features several data types, but for this discussion, we will focus on:
Strings (str): Used for representing textual data.
Integers (int): Used for whole numbers.
Floats (float): Used for numbers with decimal points.
When to Use Each Data Type
Strings
Strings should be used when you need to represent data that contains characters—whether they are alphabetic or symbolic. Here are some situations where strings are most appropriate:
Names: e.g., "Alice", "Bob".
Addresses: e.g., "123 Main St, Springfield".
Character Constants: While enums are an option, strings can be handy for straightforward constants.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Integers
Use integers when you require whole number values. Integers are ideal for:
Counters: e.g., counting the number of iterations in a loop.
Indexes: Used for accessing positions within lists.
Phone Numbers: Generally, opt for strings here if you don’t need arithmetic operations.
Important Note: If you need to carry out arithmetic operations like addition, subtraction, multiplication, or division, you must use integers rather than strings.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Floats
Floats come into play when you need to represent numbers that require decimal precision. This is especially useful for:
Financial Data: Like salaries or account balances, e.g., 49.99 (dollars) or 1000.50 (cents).
Measurements: Such as height, weight, or distance, e.g., 5.2 meters.
When working with decimals, always use floats to ensure the integrity of the values.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Changing Data Types
There might be scenarios where you need to change from one data type to another while working in Python. This is often necessary to carry out specific operations or to meet the requirements of a function. For example, if you have a numeric string and you want to perform an addition, you will need to convert it to an integer or float.
Example of converting a string to an integer:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding the proper usage of strings, integers, and floats in Python is essential for effective programming. By following the guidelines outlined in this post, you can make more informed decisions about which data type to use in different situations. Remember, always choose the data type that best fits your needs based on the nature of the data you are working with.
With practice, you'll find that managing and utilizing these data types will become second nature and will lead you to write more efficient and effective Python code. Happy coding!