filmov
tv
Understanding and Solving ZeroDivisionError in Python
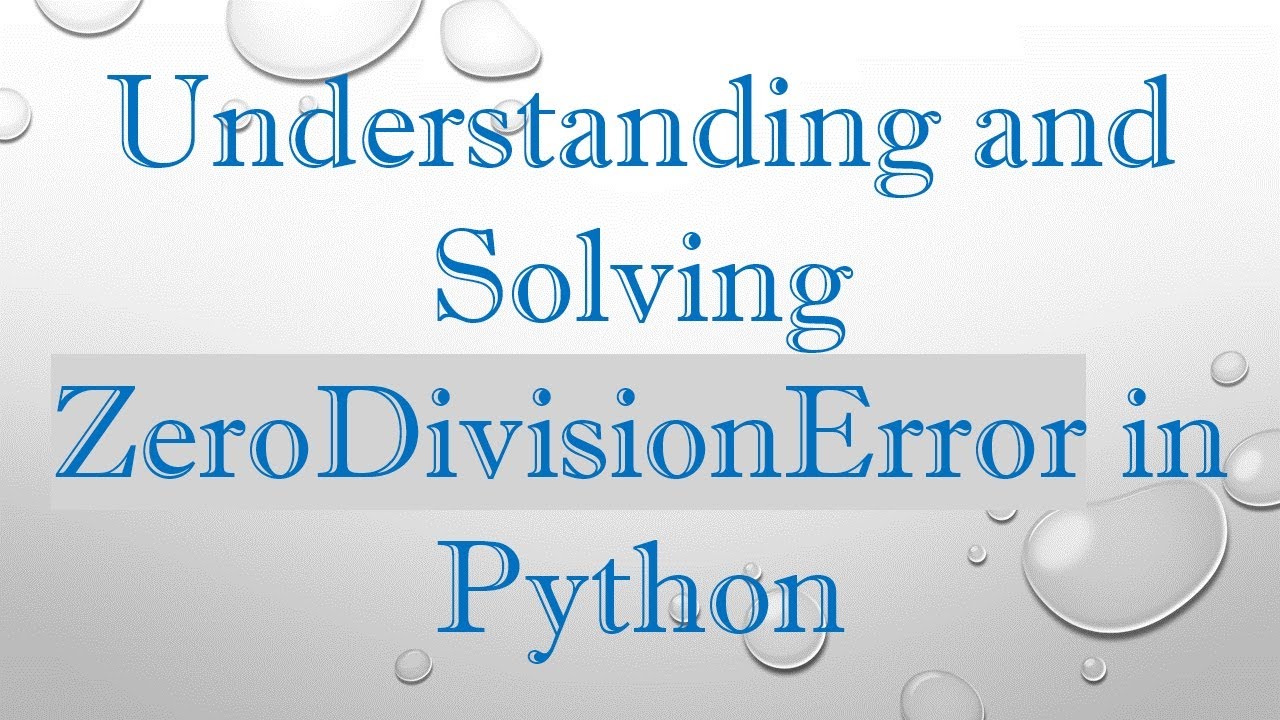
Показать описание
Learn why your Python script might be giving a ZeroDivisionError during a division operation and find helpful tips to fix this common error.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding and Solving ZeroDivisionError in Python
If you've been working with Python and encountered the ZeroDivisionError: division by zero, you are not alone. This error occurs when your Python script attempts to divide a number by zero, which is mathematically undefined.
What is ZeroDivisionError?
The ZeroDivisionError is a built-in exception in Python. When the interpreter encounters a division operation where the divisor is zero, it raises this exception to signal an error in your code. The error message typically looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Why Does This Happen?
Before understanding how to fix it, it's crucial to understand why this error occurs. Here's a basic example illustrating a scenario that might produce this error:
[[See Video to Reveal this Text or Code Snippet]]
In this snippet, the code attempts to divide 10 by 0, which is mathematically invalid. Therefore, Python raises the ZeroDivisionError exception.
How to Fix ZeroDivisionError?
Check Divisor
Ensure that the divisor in your division operation is not zero. You can put a simple conditional check before performing the division:
[[See Video to Reveal this Text or Code Snippet]]
Exception Handling
You can use Python's try-except blocks to handle the exception gracefully:
[[See Video to Reveal this Text or Code Snippet]]
This way, your script won't crash and will instead provide a clear message about the error.
User Inputs
When dealing with user inputs, always validate the input to ensure it’s not zero before performing any division:
[[See Video to Reveal this Text or Code Snippet]]
Default Values
In some scenarios, you might want to give a default value when the divisor is zero:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The ZeroDivisionError in Python signals an attempt to divide by zero, which is an essential error to handle for creating robust applications. By implementing checks, exception handling, and validating user inputs, you can effectively manage and avoid this common error in your Python scripts. Happy coding!
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding and Solving ZeroDivisionError in Python
If you've been working with Python and encountered the ZeroDivisionError: division by zero, you are not alone. This error occurs when your Python script attempts to divide a number by zero, which is mathematically undefined.
What is ZeroDivisionError?
The ZeroDivisionError is a built-in exception in Python. When the interpreter encounters a division operation where the divisor is zero, it raises this exception to signal an error in your code. The error message typically looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Why Does This Happen?
Before understanding how to fix it, it's crucial to understand why this error occurs. Here's a basic example illustrating a scenario that might produce this error:
[[See Video to Reveal this Text or Code Snippet]]
In this snippet, the code attempts to divide 10 by 0, which is mathematically invalid. Therefore, Python raises the ZeroDivisionError exception.
How to Fix ZeroDivisionError?
Check Divisor
Ensure that the divisor in your division operation is not zero. You can put a simple conditional check before performing the division:
[[See Video to Reveal this Text or Code Snippet]]
Exception Handling
You can use Python's try-except blocks to handle the exception gracefully:
[[See Video to Reveal this Text or Code Snippet]]
This way, your script won't crash and will instead provide a clear message about the error.
User Inputs
When dealing with user inputs, always validate the input to ensure it’s not zero before performing any division:
[[See Video to Reveal this Text or Code Snippet]]
Default Values
In some scenarios, you might want to give a default value when the divisor is zero:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The ZeroDivisionError in Python signals an attempt to divide by zero, which is an essential error to handle for creating robust applications. By implementing checks, exception handling, and validating user inputs, you can effectively manage and avoid this common error in your Python scripts. Happy coding!