filmov
tv
Find Eventual Safe States - Leetcode 802 - Python
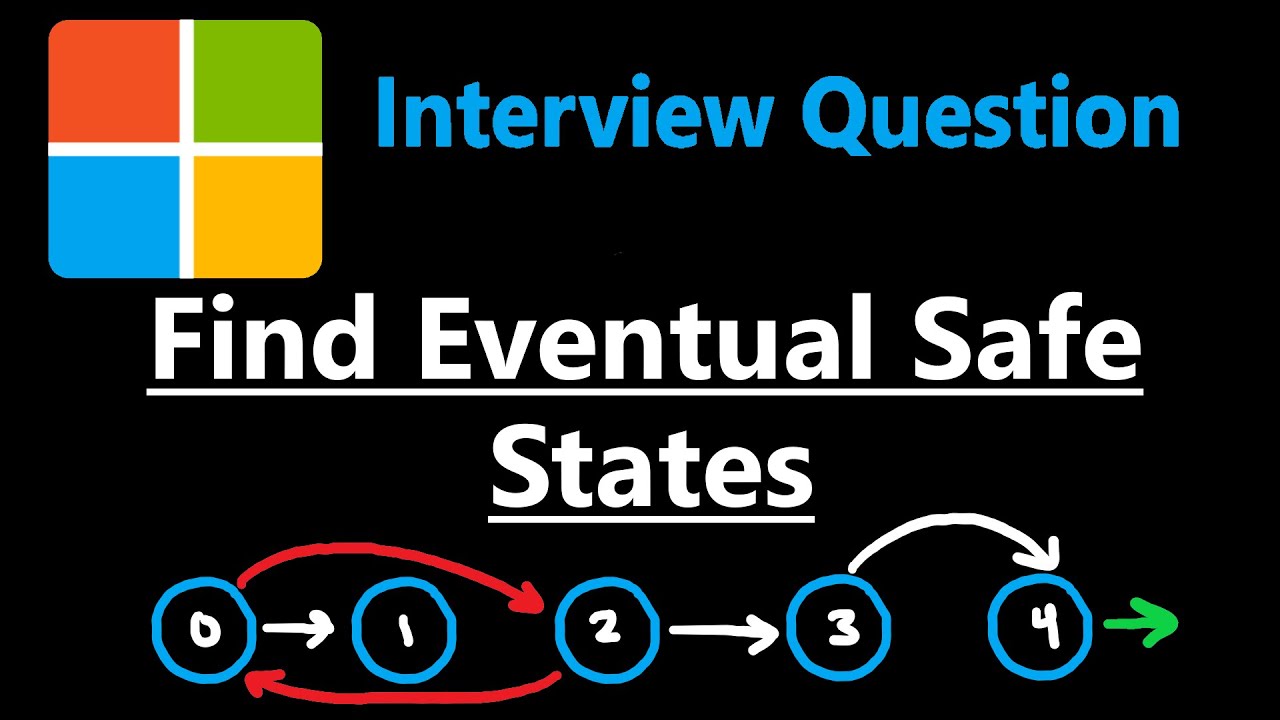
Показать описание
0:00 - Read the problem
0:55 - Drawing Explanation
5:10 - Walk Through Example
9:50 - Coding Explanation
leetcode 802
#microsoft #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Find Eventual Safe States - Leetcode 802 - Python
G-20. Find Eventual Safe States - DFS
G-25. Find Eventual Safe States - BFS - Topological Sort
Find Eventual Safe States | 2 Approaches | Intuition | Leetcode-802 | MICROSOFT | Explanation
802. Find Eventual Safe States - Day 12/31 Leetcode July Challenge
Find Eventual Safe States | Python, JavaScript, Java, C++ | LeetCode #802
Find Eventual Safe States | Detect Cycle in a Directed Graph | DFS | Hello world Graph Playlist Easy
LeetCode 802 Find Eventual Safe States
802. Find Eventual Safe States
LeetCode Medium 802 Find Eventual Safe States - Java Solution Explained Using DFS & Memoizati...
Find Eventual Safe States | Kahn's Algorithm | BFS| Python Solution
GFG POTD: 18/10/2023 | Eventual Safe States | Problem of the Day GeeksforGeeks
Find Eventual Safe States - Leetcode 802 - Python
LeetCode 802 - Find Eventual Safe States #leetcode #python #softwareengineer #interview #tech #code
Leetcode Contest 76 Problem 3 - Find Eventual Safe States
802. Find Eventual Safe States | BFS | DFS
Leetcode 802 Find Eventual Safe States Hindi
Find Eventual Safe States - LeetCode 802 - Python
Find Eventual Safe States - Leetcode 802 - JavaScript
Find Eventual Safe States | # 802 Leetcode | Python3
LeetCode - 802. Find Eventual Safe States | DFS | Java
802. Find Eventual Safe States (LeetCode, Java)
Google Preparation | Graphs | Question 13 | DAG, find-eventual-safe-states leetcode
802 Find Eventual Safe States
Комментарии