filmov
tv
Display a number on the TM1637 display with Arduino
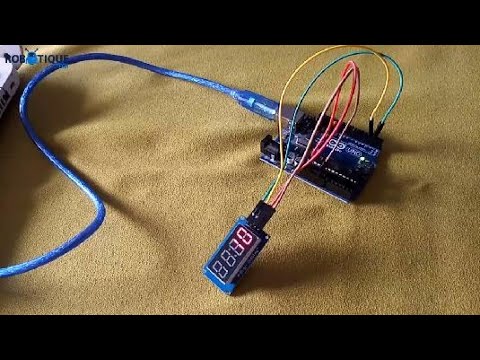
Показать описание
Displaying a number on a TM1637 display with Arduino is a simple task that can be achieved by using a library that provides functions to control the display. The library can be downloaded from the internet and installed in the Arduino development environment.
The basic steps to display a number on a TM1637 display with Arduino are:
1- Connect the TM1637 display to the Arduino board. The TM1637 display typically has four pins: VCC, GND, DIO, and CLK. The VCC and GND pins are connected to a power source (usually 3.3V or 5V), the DIO pin is connected to a digital I/O pin on the Arduino board, and the CLK pin is connected to another digital I/O pin on the Arduino board.
2- Install the TM1637 library for Arduino and include it in the code.
3- Initialize the display by creating an instance of the TM1637Display class and passing the pin numbers for the DIO and CLK pins.
4- Use the functions provided by the library to set the number to be displayed on the display.
5- Use the showNumberDec() function to display the number on the TM1637 display.
Optionally, use the setBrightness() function to adjust the brightness of the display.
Here's an example code that displays the number "1234" on a 4-digit TM1637 display using an Arduino:
#include "TM1637.h"
// Define CLK and DIO pins for the display
#define CLK 2
#define DIO 3
// Initialize the TM1637 display object
TM1637 display(CLK, DIO);
void setup() {
// Initialize the display
// Set the brightness (0-7)
}
void loop() {
// Define the number to be displayed
int number = 1234;
// Convert the number to an array of digits
int digits[4];
digits[0] = number / 1000;
digits[1] = (number / 100) % 10;
digits[2] = (number / 10) % 10;
digits[3] = number % 10;
// Display the digits on the display
}
In this code, we first define the CLK and DIO pins for the TM1637 display, and initialize a TM1637 object with these pins. We then set the display brightness to 3 (out of 7) in the setup() function.
Note that the TM1637 library needs to be installed in your Arduino IDE for this code to work. You can install it by going to "Sketch - Include Library - Manage Libraries..." and searching for "TM1637".
The basic steps to display a number on a TM1637 display with Arduino are:
1- Connect the TM1637 display to the Arduino board. The TM1637 display typically has four pins: VCC, GND, DIO, and CLK. The VCC and GND pins are connected to a power source (usually 3.3V or 5V), the DIO pin is connected to a digital I/O pin on the Arduino board, and the CLK pin is connected to another digital I/O pin on the Arduino board.
2- Install the TM1637 library for Arduino and include it in the code.
3- Initialize the display by creating an instance of the TM1637Display class and passing the pin numbers for the DIO and CLK pins.
4- Use the functions provided by the library to set the number to be displayed on the display.
5- Use the showNumberDec() function to display the number on the TM1637 display.
Optionally, use the setBrightness() function to adjust the brightness of the display.
Here's an example code that displays the number "1234" on a 4-digit TM1637 display using an Arduino:
#include "TM1637.h"
// Define CLK and DIO pins for the display
#define CLK 2
#define DIO 3
// Initialize the TM1637 display object
TM1637 display(CLK, DIO);
void setup() {
// Initialize the display
// Set the brightness (0-7)
}
void loop() {
// Define the number to be displayed
int number = 1234;
// Convert the number to an array of digits
int digits[4];
digits[0] = number / 1000;
digits[1] = (number / 100) % 10;
digits[2] = (number / 10) % 10;
digits[3] = number % 10;
// Display the digits on the display
}
In this code, we first define the CLK and DIO pins for the TM1637 display, and initialize a TM1637 object with these pins. We then set the display brightness to 3 (out of 7) in the setup() function.
Note that the TM1637 library needs to be installed in your Arduino IDE for this code to work. You can install it by going to "Sketch - Include Library - Manage Libraries..." and searching for "TM1637".