filmov
tv
Arduino Tutorial 12: Arduino Control Structures
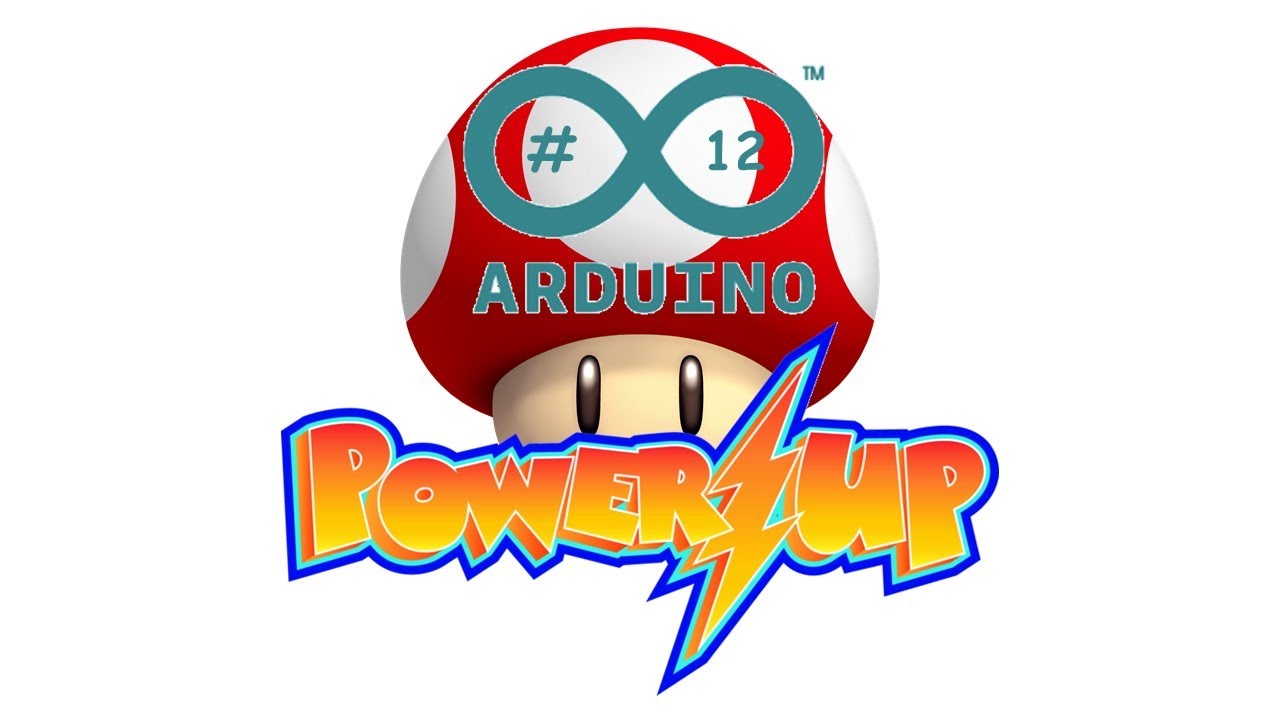
Показать описание
Control structures ( a.k.a. conditional statements & loops) let you do some amazing things with your Arduino and a little amount of code.
The if statement checks for a condition and executes the proceeding statement or set of statements if the condition is true.
The syntax for an if statement is simple and goes something like this:
If (condition)
{
//Do some stuff
}
An if…else statement is a power-up for your if statements. It allows you greater control over the flow of code than the basic if statement. This will allow you to test for more than one condition. Note that we can use as many else…if statements as we like.
The for loop is one of the most useful and common pieces of code out there. It’s often used with arrays, a subject that is a bit beyond the scope of basic programming but one you’ll want to become familiar with.
The syntax is shown below.
For (initialization; condition; incrementation) {
//code goes here…
}
At first, the for loop can be confusing and intimidating, but after a while you’ll become good friends.
The while loop is another gem you’ll often find yourself using.
It will loop continuously (and forever) until the expression inside the parenthesis becomes false.
The syntax is shown here.
While(condition) {
//do this stuff as long as condition is true…
}
Note that the condition is a Boolean expression and it either true or false. The condition can be many things like some sort of less than or equal comparison, the number 1 (which means true; putting a 1 in there will make the while loop run forever), or even a function.
That wraps up our intro to control structures. There are other control structures in the land of Arduino, however I wanted to cove the ones you’ll see the most. We’ll talk about some of the other ones in another tutorial.