filmov
tv
Solving the ValueError in AWS Lambda with Python: A Guide to Handling Unpacking Errors
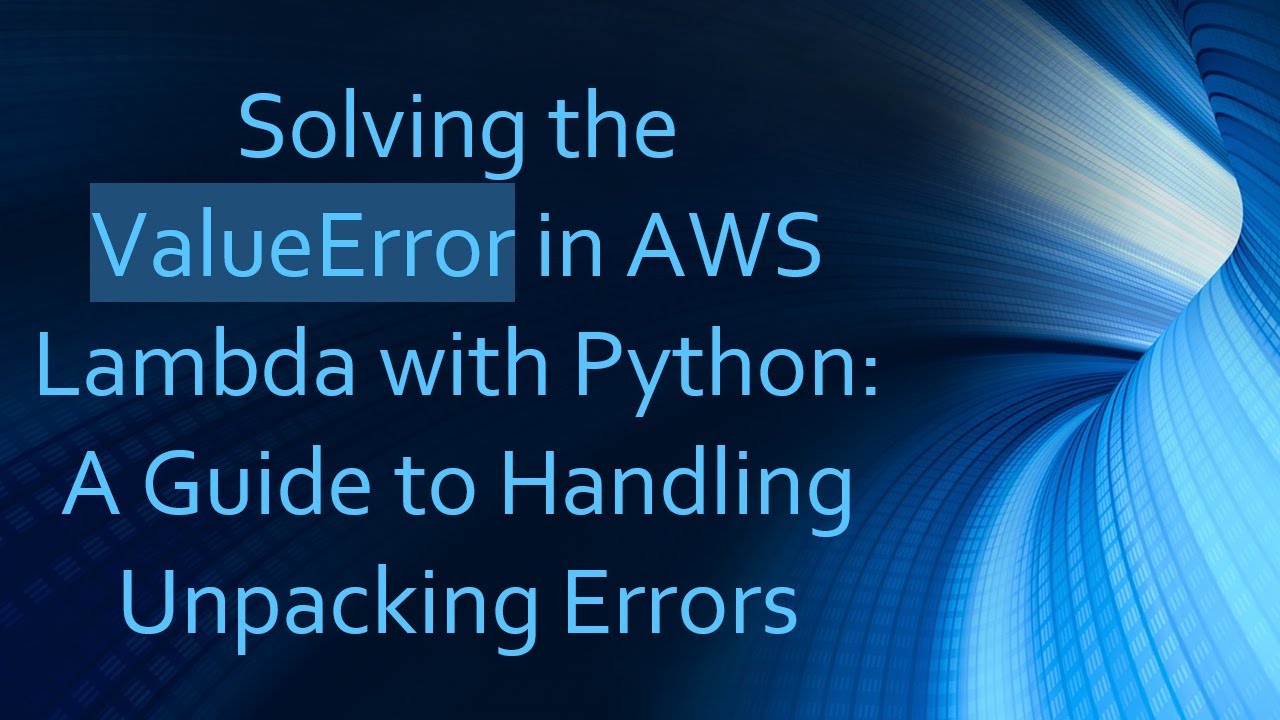
Показать описание
Discover how to resolve the `not enough values to unpack` error while testing AWS Lambda functions with Python. We cover practical solutions to ensure your Lambda functions run smoothly without any unpacking mistakes.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error when testing Python lambda in AWS console
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the ValueError in AWS Lambda with Python: A Guide to Handling Unpacking Errors
When developing Lambda functions in Python, encountering errors is a normal part of the process. One common issue developers face is the ValueError message "not enough values to unpack (expected 2, got 1)" while testing their Lambda function in the AWS console. This guide will walk you through understanding the problem and provide you with a clear solution.
Understanding the Problem
Here's a brief overview of the problem:
You have implemented a Lambda function that processes events from AWS Kinesis streams using Python.
When you test this function, it throws a ValueError indicating that it couldn’t unpack values as expected.
This usually happens during the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
The expectation is that split("/stream") will always return two elements: the table_arn and some other value. However, if the string does not contain the /stream substring, your program will encounter a ValueError.
Error Analysis
To understand why this error occurs, let’s break it down:
Splitting the String: The split method breaks a string into a list based on the specified delimiter. In this case, /stream is the delimiter.
Uneven Split: If record["eventSourceARN"] does not contain /stream, the split function returns a list with fewer than two elements. Hence, trying to unpack it into two variables (table_arn and _) raises the ValueError.
Example of the Problem
Here’s a simplified representation of the error:
[[See Video to Reveal this Text or Code Snippet]]
Solution
The goal is to make your code more robust by ensuring it can handle unexpected cases without throwing errors. Here’s how to approach the solution:
Step 1: Check Split Results
Instead of directly unpacking the results from the split operation, you should first check how many elements it returns. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Error Handling
By implementing a check, you can prevent the ValueError and handle abnormal situations gracefully:
Log the Error: Print a message or log the error so you understand why it occurred.
Return a Specific Response: If the structure is not as expected, return an error response from the Lambda function.
Conclusion
In conclusion, the ValueError can be managed effectively by ensuring you inspect the output of the split method before trying to unpack it. This simple change to your Lambda function will not only solve the immediate problem but also make your code more reliable in handling unexpected scenarios.
Follow these guidelines to enhance your AWS Lambda function’s robustness, ensuring smoother execution during testing and production.
If you have any more questions or need further assistance with AWS Lambda and Python, feel free to reach out.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Error when testing Python lambda in AWS console
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the ValueError in AWS Lambda with Python: A Guide to Handling Unpacking Errors
When developing Lambda functions in Python, encountering errors is a normal part of the process. One common issue developers face is the ValueError message "not enough values to unpack (expected 2, got 1)" while testing their Lambda function in the AWS console. This guide will walk you through understanding the problem and provide you with a clear solution.
Understanding the Problem
Here's a brief overview of the problem:
You have implemented a Lambda function that processes events from AWS Kinesis streams using Python.
When you test this function, it throws a ValueError indicating that it couldn’t unpack values as expected.
This usually happens during the following line of code:
[[See Video to Reveal this Text or Code Snippet]]
The expectation is that split("/stream") will always return two elements: the table_arn and some other value. However, if the string does not contain the /stream substring, your program will encounter a ValueError.
Error Analysis
To understand why this error occurs, let’s break it down:
Splitting the String: The split method breaks a string into a list based on the specified delimiter. In this case, /stream is the delimiter.
Uneven Split: If record["eventSourceARN"] does not contain /stream, the split function returns a list with fewer than two elements. Hence, trying to unpack it into two variables (table_arn and _) raises the ValueError.
Example of the Problem
Here’s a simplified representation of the error:
[[See Video to Reveal this Text or Code Snippet]]
Solution
The goal is to make your code more robust by ensuring it can handle unexpected cases without throwing errors. Here’s how to approach the solution:
Step 1: Check Split Results
Instead of directly unpacking the results from the split operation, you should first check how many elements it returns. Here’s an example:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Error Handling
By implementing a check, you can prevent the ValueError and handle abnormal situations gracefully:
Log the Error: Print a message or log the error so you understand why it occurred.
Return a Specific Response: If the structure is not as expected, return an error response from the Lambda function.
Conclusion
In conclusion, the ValueError can be managed effectively by ensuring you inspect the output of the split method before trying to unpack it. This simple change to your Lambda function will not only solve the immediate problem but also make your code more reliable in handling unexpected scenarios.
Follow these guidelines to enhance your AWS Lambda function’s robustness, ensuring smoother execution during testing and production.
If you have any more questions or need further assistance with AWS Lambda and Python, feel free to reach out.