filmov
tv
Dp 34 wildcard matching recursive to 1d array optimisation
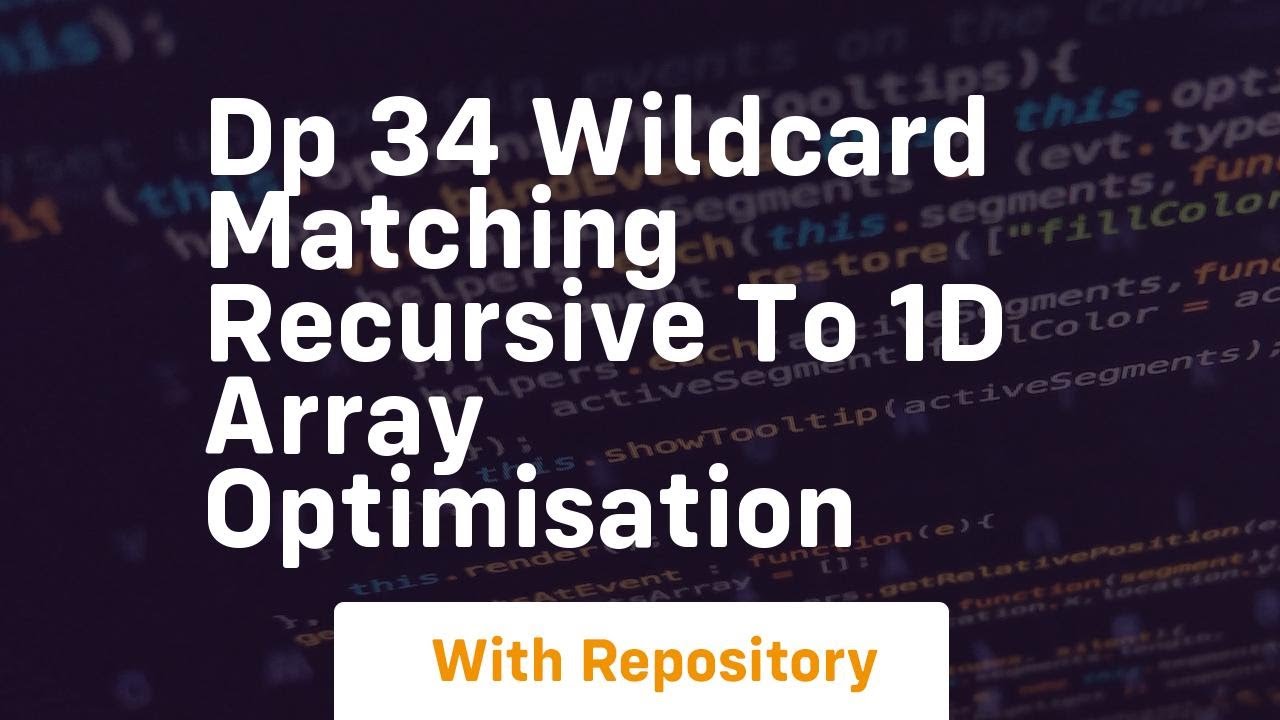
Показать описание
dp wildcard matching: from recursive to 1d array optimization
this tutorial dives deep into the classic "wildcard matching" problem, exploring various dp (dynamic programming) approaches. we'll start with a recursive solution, gradually optimize it using memoization, then transform it into a 2d dp table, and finally arrive at the highly efficient 1d array optimization. each step will be accompanied by detailed explanations and code examples in python.
**problem statement:**
given an input string `s` and a pattern `p`, implement wildcard pattern matching with support for `'?'` and `'*'`:
* `'?'` matches any single character.
* `'*'` matches any sequence of characters (including the empty sequence).
the matching should cover the entire input string (not partial).
**example:**
* `s = "aa"`, `p = "a"` - `false` (doesn't cover the entire string)
* `s = "aa"`, `p = "*"` - `true` (matches any sequence)
* `s = "cb"`, `p = "?a"` - `false` (`?` matches 'c', but 'a' doesn't match 'b')
* `s = "adceb"`, `p = "*a*b"` - `true` (first `*` matches "adc", second `*` matches "e")
* `s = "acdcb"`, `p = "a*c?b"` - `false`
**1. recursive approach (without memoization - brute force):**
this is the most straightforward but least efficient approach. we explore all possible matching combinations.
**explanation:**
* `is_match_recursive(s, p)`: the main function that initiates the matching process.
* `solve(i, j)`: a recursive helper function that checks if `s[i:]` matches `p[j:]`.
* **base cases:**
* if both `i` and `j` reach the end of their respective strings, it means we have a complete match.
* if `j` reaches the end of `p` but `i` hasn't reached the end of `s`, it means the pattern is exhausted before the string, so it's a mismatch.
* if `i` reaches the end of `s` but `j` hasn't reached the end of `p`, we need to check if the remaining part of `p` consists only of `'*'` characters. if so, it's a match (because `'*'` can match an ...
#Dp34 #WildcardMatching #numpy
Dp 34
wildcard matching
recursive optimization
1D array
dynamic programming
pattern matching
substring search
algorithm optimization
efficiency improvement
memory reduction
computational complexity
string comparison
backtracking
state space reduction
problem-solving techniques
this tutorial dives deep into the classic "wildcard matching" problem, exploring various dp (dynamic programming) approaches. we'll start with a recursive solution, gradually optimize it using memoization, then transform it into a 2d dp table, and finally arrive at the highly efficient 1d array optimization. each step will be accompanied by detailed explanations and code examples in python.
**problem statement:**
given an input string `s` and a pattern `p`, implement wildcard pattern matching with support for `'?'` and `'*'`:
* `'?'` matches any single character.
* `'*'` matches any sequence of characters (including the empty sequence).
the matching should cover the entire input string (not partial).
**example:**
* `s = "aa"`, `p = "a"` - `false` (doesn't cover the entire string)
* `s = "aa"`, `p = "*"` - `true` (matches any sequence)
* `s = "cb"`, `p = "?a"` - `false` (`?` matches 'c', but 'a' doesn't match 'b')
* `s = "adceb"`, `p = "*a*b"` - `true` (first `*` matches "adc", second `*` matches "e")
* `s = "acdcb"`, `p = "a*c?b"` - `false`
**1. recursive approach (without memoization - brute force):**
this is the most straightforward but least efficient approach. we explore all possible matching combinations.
**explanation:**
* `is_match_recursive(s, p)`: the main function that initiates the matching process.
* `solve(i, j)`: a recursive helper function that checks if `s[i:]` matches `p[j:]`.
* **base cases:**
* if both `i` and `j` reach the end of their respective strings, it means we have a complete match.
* if `j` reaches the end of `p` but `i` hasn't reached the end of `s`, it means the pattern is exhausted before the string, so it's a mismatch.
* if `i` reaches the end of `s` but `j` hasn't reached the end of `p`, we need to check if the remaining part of `p` consists only of `'*'` characters. if so, it's a match (because `'*'` can match an ...
#Dp34 #WildcardMatching #numpy
Dp 34
wildcard matching
recursive optimization
1D array
dynamic programming
pattern matching
substring search
algorithm optimization
efficiency improvement
memory reduction
computational complexity
string comparison
backtracking
state space reduction
problem-solving techniques