filmov
tv
LeetCode 560. Subarray Sum Equals K Explanation and Solution
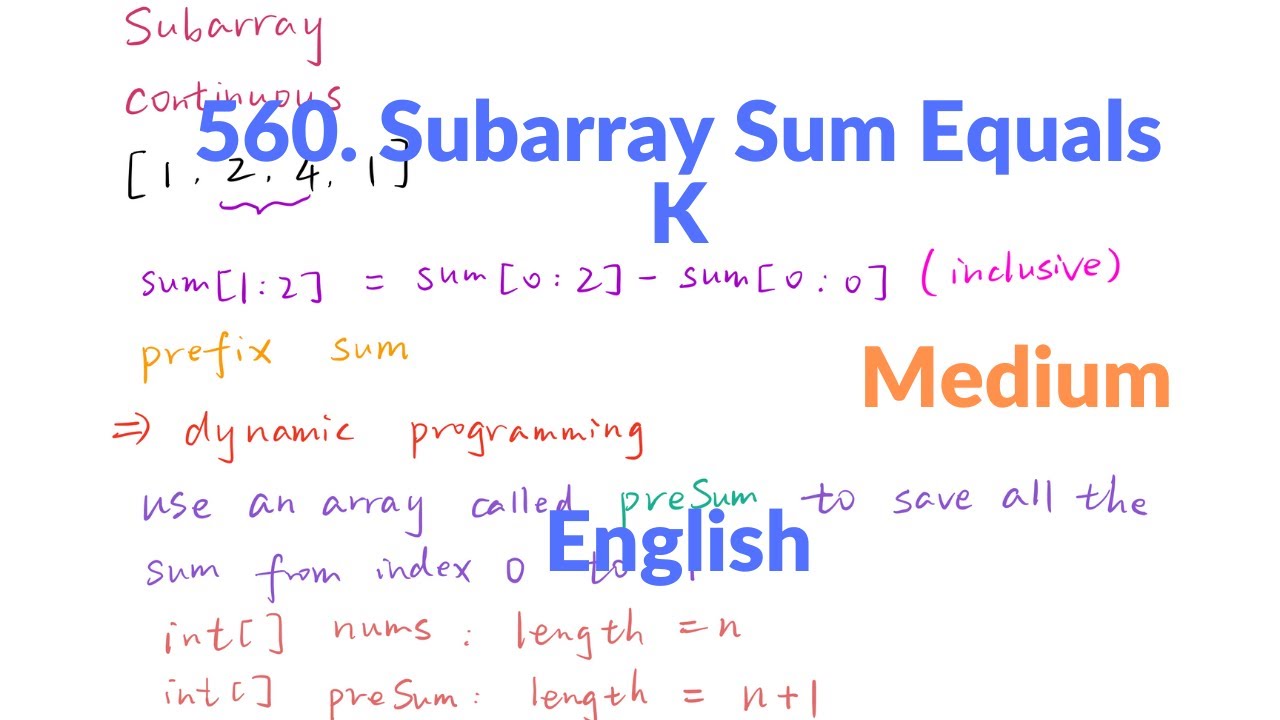
Показать описание
Thanks in advance. :D
Happy coding every day!
Subarray Sum Equals K - Prefix Sums - Leetcode 560 - Python
LeetCode 560 | Subarray Sum Equals K | Solution Explained (Java + Whiteboard)
Subarray Sum Equals K | Prefix Sums | Leetcode 560 | Animation | Intuition
LeetCode Subarray Sum Equals K Solution Explained - Java
Subarray sum equals K | Number of subarrays with sum equals K | Leetcode #560
Subarray Sum Equals K - LeetCode 560 - Coding Interview Questions
Meta Coding Interview - Subarray Sum Equals K - Leetcode 560
SUBARRAY SUM EQUALS K | LEETCODE 560 | PYTHON SOLUTION
Subarray Sum Equals K | Intuitive Explanation + Code (LeetCode 560)
LeetCode Day 22 - Subarray Sum Equals K
leetcode 560. #shorts Maximum Size Subarray Sum Equals k
Leetcode 560. Subarray Sum Equals K
Leetcode 560 - Subarray Sum Equals K (JAVA Solution Explained!)
Subarray Sum Equals K - 560. LeetCode - Java
Subarray Sum Equals K - LeetCode 560 - Python
25. Subarray Sum Equals K | Leetcode 560 | Array | Prefix Sum
560. Subarray Sum Equals K(Leetcode Solution)
Count Subarray sum Equals K | Brute - Better -Optimal
Google Coding Interview Question - Leetcode 560. Subarray Sum Equals K
560. Subarray Sum Equals K - Day 10/28 Leetcode February Challenge
LeetCode 560. Subarray Sum Equals K Explanation and Solution
[SOLVED!] Subarray Sum Equals K - LeetCode 560 - Java
LeetCode - 560. Subarray Sum Equals K | Prefix sum | Java
Subarray Sum Equals K | LeetCode 560 | Coders Camp
Комментарии