filmov
tv
Introduction to Linked List | C++ Placement Course | Lecture 22
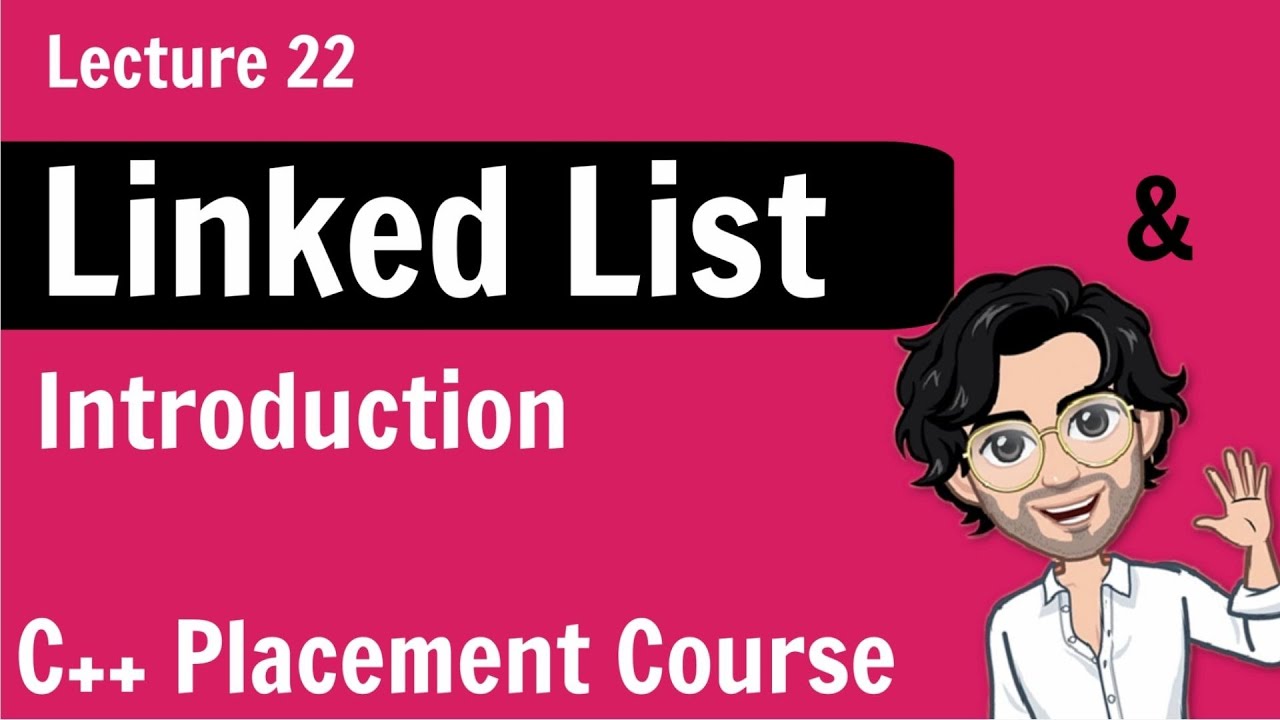
Показать описание
Introduction to Linked List
Introduction to Linked Lists (Data Structures & Algorithms #5)
Introduction to linked list
Learn Linked Lists in 13 minutes 🔗
2.1 Introduction to Linked List | Need of Linked List | DSA Tutorials
Linked lists in 4 minutes
Introduction to Linked Lists - Data Structures and Algorithms
Introduction to Linked Lists, Arrays vs Linked Lists, Advantages/Disadvantages - C++ Data Structures
Think Like a Programmer: An Introduction to Creative Problem Solving - Deep Book Review
#4 Introduction to Linked List | Data Structures
Introduction to Linked List | Data Structures & Algorithms | Java Placement Course
Lec-16: Introduction to Linked List | Types and Need of linked list | data structures
Introduction to Linked List in Data Structures (With Notes)
[c] Introduction to Linked Lists
How to Create a Linked List C++ Introduction to Linked Lists
Introduction to Linked List | C++ Placement Course | Lecture 22
Introduction to Linked List
How Insertion in Linked List Works ? 🤔😏
Introduction to Linked List data structure easy explain
Data Structures: Introduction to Linked Lists
Linked Lists Introduction
L1. Introduction to LinkedList | Traversal | Length | Search an Element
Introduction to Linked Lists
LinkedList 1 Introduction
Комментарии