filmov
tv
Abstract classes in JavaScript
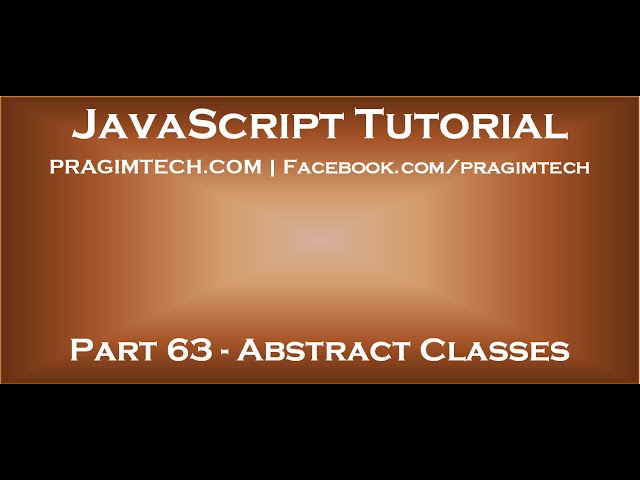
Показать описание
Link for all dot net and sql server video tutorial playlists
Link for slides, code samples and text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
In this video we will discuss, how to implement abstract classes concept in JavaScript.
Object oriented programming languages like C# and Java, support abstract classes. Abstract classes are incomplete. So, trying to create an instance of an abstract class raises a compiler error. Abstract classes can only be used as base classes.
Let us first look at a simple C# example.
using System;
namespace Demo
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
// Error: Cannot create an instance of an abstract class
// Shape s = new Shape();
Circle circle = new Circle();
Response.Write(circle is Shape + "[br/]"); // Returns true
Response.Write(circle is Circle + "[br/]"); // Returns true
}
}
public abstract class Shape
{
public string shapeName = "None";
public string draw()
{
}
}
public class Circle : Shape
{
// Code specific to Circl class
}
}
Since JavaScript is also an object oriented programming language, it also supports the concept of abstract classes. Here is an example.
[script type="text/javascript"]
// Create a Shape object which is abstract
var Shape = function ()
{
throw new Error("Cannot create an instance of abstract class");
}
// Error : Cannot create an instance of abstract class
// var shape = new Shape();
// Add draw function to the Shape prototype
// Objects derived from Shape should be able to call draw() method
{
}
// Create a Circle object
var Circle = function (shapeName)
{
}
// Make shape the parent for Circle
var circle = new Circle("Circle");
// Since Circle inherits from abstract Shape object, it can call draw() method
alert(circle instanceof Circle); // Returns true
alert(circle instanceof Shape); // Returns true
[/script]
Link for slides, code samples and text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
In this video we will discuss, how to implement abstract classes concept in JavaScript.
Object oriented programming languages like C# and Java, support abstract classes. Abstract classes are incomplete. So, trying to create an instance of an abstract class raises a compiler error. Abstract classes can only be used as base classes.
Let us first look at a simple C# example.
using System;
namespace Demo
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
// Error: Cannot create an instance of an abstract class
// Shape s = new Shape();
Circle circle = new Circle();
Response.Write(circle is Shape + "[br/]"); // Returns true
Response.Write(circle is Circle + "[br/]"); // Returns true
}
}
public abstract class Shape
{
public string shapeName = "None";
public string draw()
{
}
}
public class Circle : Shape
{
// Code specific to Circl class
}
}
Since JavaScript is also an object oriented programming language, it also supports the concept of abstract classes. Here is an example.
[script type="text/javascript"]
// Create a Shape object which is abstract
var Shape = function ()
{
throw new Error("Cannot create an instance of abstract class");
}
// Error : Cannot create an instance of abstract class
// var shape = new Shape();
// Add draw function to the Shape prototype
// Objects derived from Shape should be able to call draw() method
{
}
// Create a Circle object
var Circle = function (shapeName)
{
}
// Make shape the parent for Circle
var circle = new Circle("Circle");
// Since Circle inherits from abstract Shape object, it can call draw() method
alert(circle instanceof Circle); // Returns true
alert(circle instanceof Shape); // Returns true
[/script]
Комментарии