filmov
tv
python for loop with increment
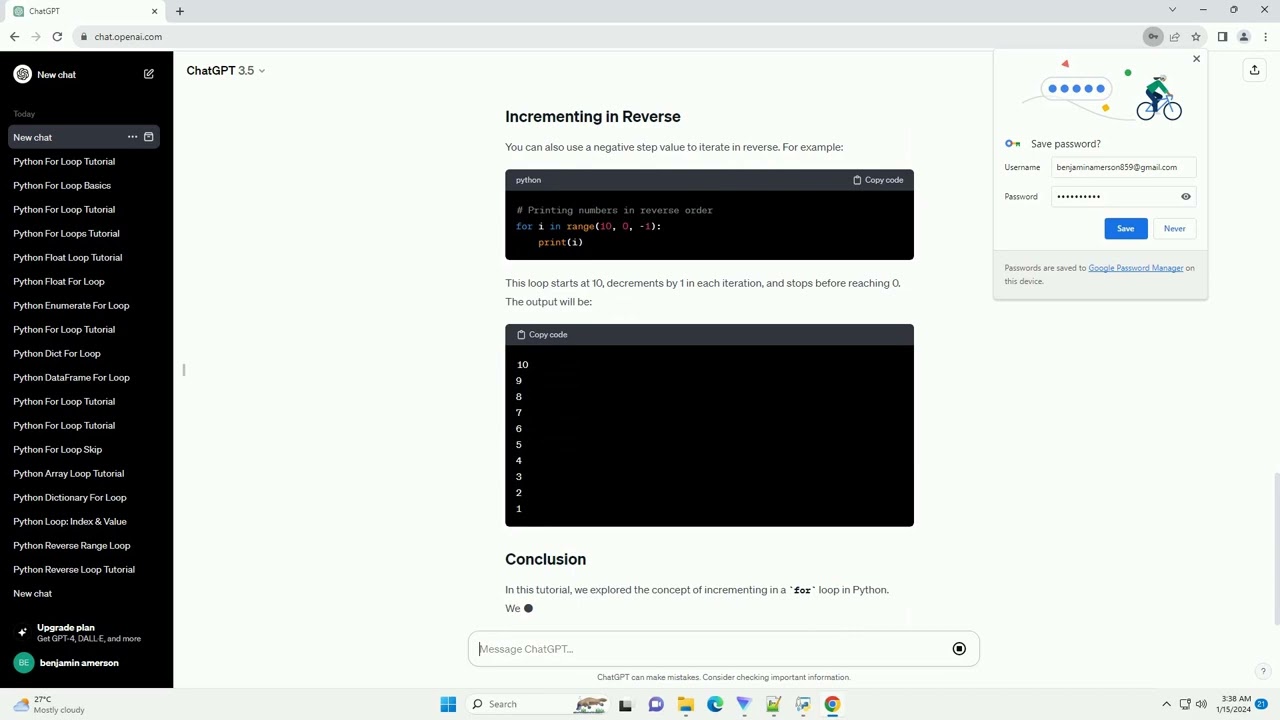
Показать описание
The for loop is a powerful construct in Python used for iterating over a sequence (such as a list, tuple, string, or range). While the basic for loop syntax is straightforward, understanding how to use an increment or step value can enhance its functionality. In this tutorial, we will explore the concept of incrementing in a for loop in Python with detailed explanations and practical examples.
The basic syntax of a for loop in Python is as follows:
Here, variable takes on the values in the sequence one by one, and the indented code block under the for statement is executed for each iteration.
The range() function is commonly used to generate a sequence of numbers, and it can be employed to control the loop's iteration. The range() function has the following general syntax:
To increment the loop variable by a specific value, you can use the range() function with the appropriate start, stop, and step values. Here's an example:
In this example, the loop will start at 0, end before 10, and increment by 2 in each iteration. The output will be:
Let's say you want to print the first 10 even numbers using a for loop with increment. You can achieve this with the following code:
This loop starts at 0, ends before 20, and increments by 2 in each iteration. The output will be:
You can also use a negative step value to iterate in reverse. For example:
This loop starts at 10, decrements by 1 in each iteration, and stops before reaching 0. The output will be:
In this tutorial, we explored the concept of incrementing in a for loop in Python. We covered the basic for loop syntax, the use of the range() function to control iteration, and provided practical examples for better understanding. By mastering incrementing in for loops, you can efficiently iterate over sequences with custom step values, making your code more flexible and concise.
ChatGPT
The basic syntax of a for loop in Python is as follows:
Here, variable takes on the values in the sequence one by one, and the indented code block under the for statement is executed for each iteration.
The range() function is commonly used to generate a sequence of numbers, and it can be employed to control the loop's iteration. The range() function has the following general syntax:
To increment the loop variable by a specific value, you can use the range() function with the appropriate start, stop, and step values. Here's an example:
In this example, the loop will start at 0, end before 10, and increment by 2 in each iteration. The output will be:
Let's say you want to print the first 10 even numbers using a for loop with increment. You can achieve this with the following code:
This loop starts at 0, ends before 20, and increments by 2 in each iteration. The output will be:
You can also use a negative step value to iterate in reverse. For example:
This loop starts at 10, decrements by 1 in each iteration, and stops before reaching 0. The output will be:
In this tutorial, we explored the concept of incrementing in a for loop in Python. We covered the basic for loop syntax, the use of the range() function to control iteration, and provided practical examples for better understanding. By mastering incrementing in for loops, you can efficiently iterate over sequences with custom step values, making your code more flexible and concise.
ChatGPT