filmov
tv
Sorting a Sentence (LeetCode 1859) | Solution with examples | Detail explanation | Study Algorithms
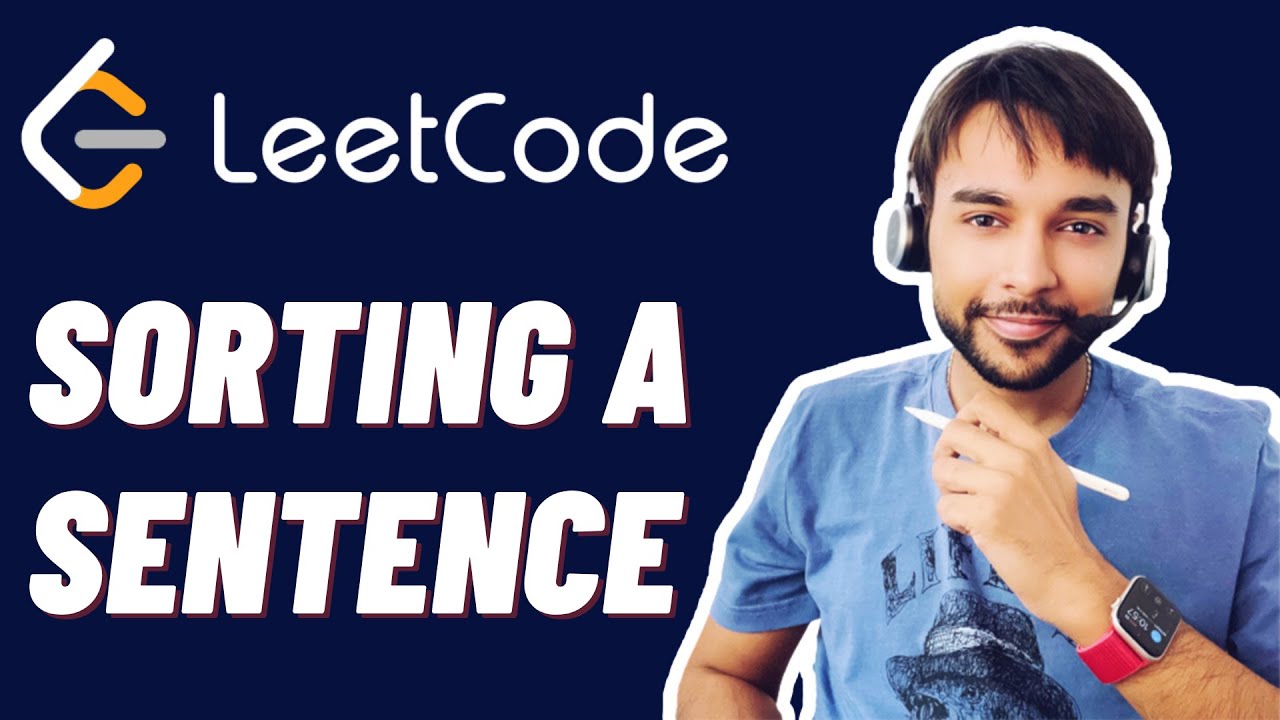
Показать описание
Whenever you are given a sentence string, the best way to iterate over each word is to take advantage of the space (“ “) character. This will help you to separate out different words. We take the help of this idea to solve this easy problem on LeetCode. Watch the video to understand how a HashSet can help you optimize your solution with time. All of this with examples and a dry-run of code in JAVA
Chapters:
00:00 - Intro
00:54 - Problem statement and description
03:00 - How to approach the problem?
04:36 - Solving for efficiency
07:04 - Dry-run of Code
09:33 - Final Thoughts
📚 Links to topics I talk about in the video:
📖 Reference Books:
🎥 My Recording Gear:
💻 Get Social 💻
#hashset #leetcode #algorithms
Chapters:
00:00 - Intro
00:54 - Problem statement and description
03:00 - How to approach the problem?
04:36 - Solving for efficiency
07:04 - Dry-run of Code
09:33 - Final Thoughts
📚 Links to topics I talk about in the video:
📖 Reference Books:
🎥 My Recording Gear:
💻 Get Social 💻
#hashset #leetcode #algorithms
Комментарии