filmov
tv
Insert() vs Extend() vs Append() functions in python list methods. | #pythontips #pythontutorial
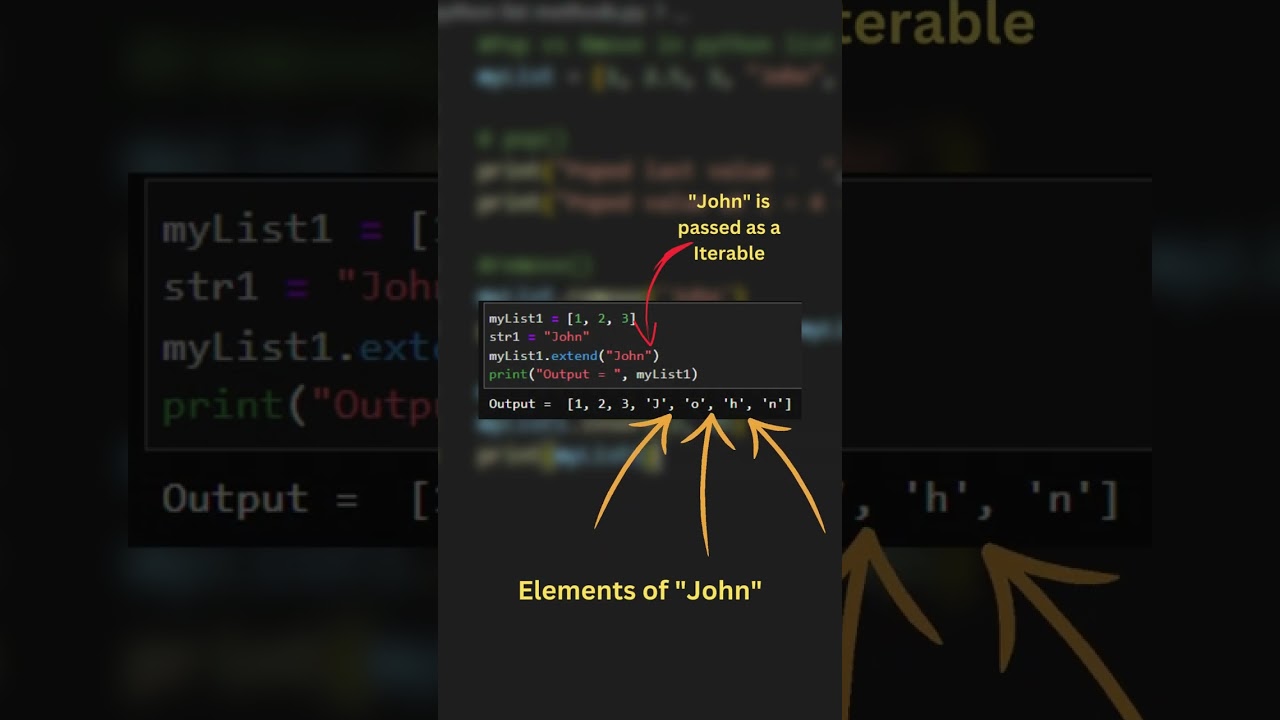
Показать описание
Difference between insert, extend and append functions in python list methods.
In Python, lists are a very commonly used data structure. There are several built-in methods to manipulate lists, including insert(), extend(), and append(). Although these methods may seem similar, they have different functionalities.
Here's a brief explanation of each method:
- `append(item)` adds a single item to the end of the list. The argument passed to append() is added as a single element, and it doesn't matter whether it is a list, tuple, or any other sequence. The item is appended to the end of the list as a single element.
- `extend(iterable)` adds all the items from an iterable (such as a list, tuple, string, or set) to the end of the list. The argument passed to extend() must be an iterable, and each element of the iterable is added to the list individually.
- `insert(index, item)` inserts an item at a specified index. The first argument specifies the index where the item should be inserted, and the second argument is the item to be inserted. All the items after the specified index are shifted one position to the right to accommodate the new item.
Here's an example to demonstrate the difference between the methods:
# Example list
my_list = [1, 2, 3]
# Append an item to the list
# Result: [1, 2, 3, 4]
# Extend the list with another list
# Result: [1, 2, 3, 4, 5, 6]
# Insert an item at a specified index
# Result: [1, 2, 'hello', 3, 4, 5, 6]
Note that append() and extend() modify the original list, while insert() can modify the original list or create a new list if used on a copy of the original list.
#python #python3 #pythonbeginner #pythonprogramming #pythontutorial #pythonforbeginners #pythonstatus #pythontutorialforbeginner #pythontips #tipsandtricks #tips #tricks #insert #append #extend #pythonlist #listmethods
In Python, lists are a very commonly used data structure. There are several built-in methods to manipulate lists, including insert(), extend(), and append(). Although these methods may seem similar, they have different functionalities.
Here's a brief explanation of each method:
- `append(item)` adds a single item to the end of the list. The argument passed to append() is added as a single element, and it doesn't matter whether it is a list, tuple, or any other sequence. The item is appended to the end of the list as a single element.
- `extend(iterable)` adds all the items from an iterable (such as a list, tuple, string, or set) to the end of the list. The argument passed to extend() must be an iterable, and each element of the iterable is added to the list individually.
- `insert(index, item)` inserts an item at a specified index. The first argument specifies the index where the item should be inserted, and the second argument is the item to be inserted. All the items after the specified index are shifted one position to the right to accommodate the new item.
Here's an example to demonstrate the difference between the methods:
# Example list
my_list = [1, 2, 3]
# Append an item to the list
# Result: [1, 2, 3, 4]
# Extend the list with another list
# Result: [1, 2, 3, 4, 5, 6]
# Insert an item at a specified index
# Result: [1, 2, 'hello', 3, 4, 5, 6]
Note that append() and extend() modify the original list, while insert() can modify the original list or create a new list if used on a copy of the original list.
#python #python3 #pythonbeginner #pythonprogramming #pythontutorial #pythonforbeginners #pythonstatus #pythontutorialforbeginner #pythontips #tipsandtricks #tips #tricks #insert #append #extend #pythonlist #listmethods