filmov
tv
Mastering Nested Functions in JavaScript: Creating a Counter
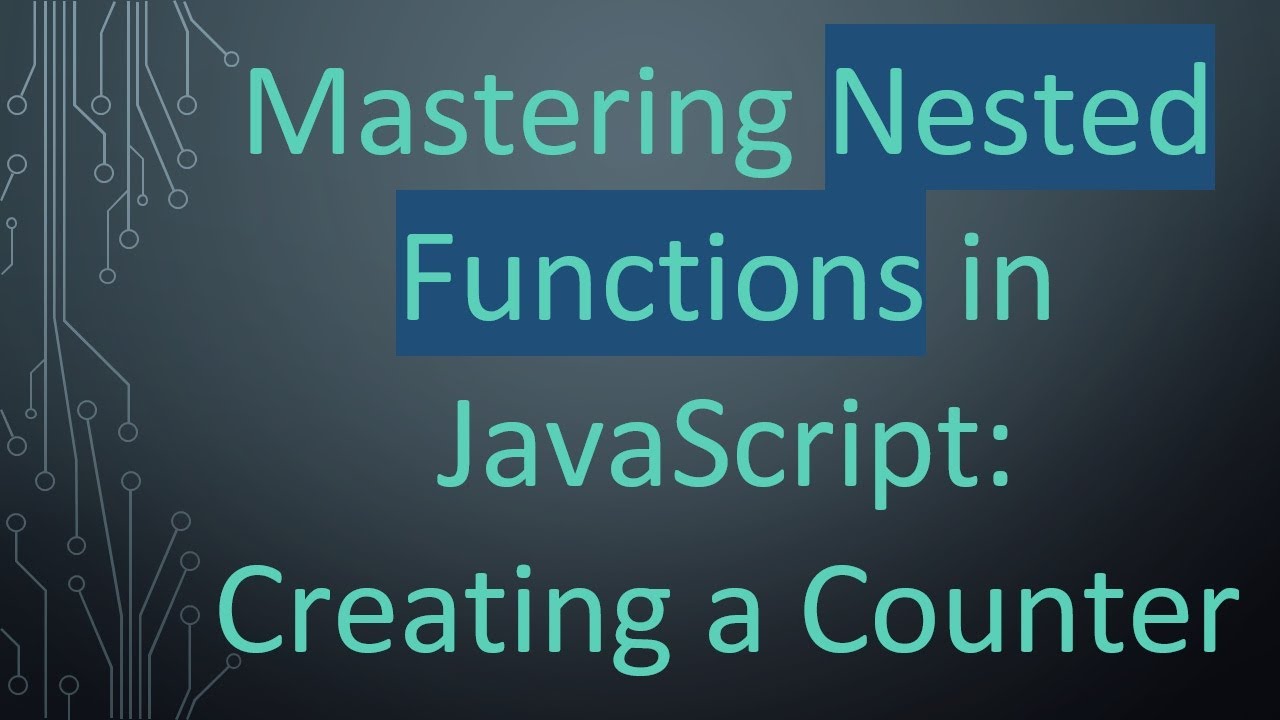
Показать описание
Learn how to effectively use `nested functions` in JavaScript to create a customizable counter with unique functionalities.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Calling a nested function JavaScript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Nested Functions in JavaScript: Creating a Counter
As a budding JavaScript developer, you may often encounter the challenge of using nested functions effectively. One particular use case involves creating a counter with various functionalities. In this guide, we'll break down how to build such a counter and how to call the nested functions outside of its defining context. Let's get started!
The Problem: Creating a Functional Counter
Imagine you need a JavaScript counter that provides you with three essential functionalities:
counter() should return the next number incrementally starting from zero.
Here’s a simple implementation to illustrate this concept:
[[See Video to Reveal this Text or Code Snippet]]
Now the question arises, how do we call these nested functions from outside their context? The answer lies in returning the counter function from our makeCounter function. Let’s dive deeper into this solution.
The Solution: Returning the Counter Function
To gain access to the nested functions (i.e., decrease and set), follow these steps:
Step 1: Modify the makeCounter function
To expose the counter, you need to return it from makeCounter. Here’s how it should look:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Instantiate the Counter
Next, you’ll create a new instance of the counter using the makeCounter function:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Use the Counter Functions
Now that we have an instance of the counter, we can call its functionalities easily:
[[See Video to Reveal this Text or Code Snippet]]
Important Notes on Counter Behavior
When using count++, be aware that it will return the current value of count before incrementing it. If you want to increment and then return the updated value, consider using ++count instead.
Understanding the implications of nested functions can greatly enhance your JavaScript programming capabilities, especially when dealing with closures and encapsulation.
Conclusion
By returning the counter function from makeCounter, you can effectively manage a customizable counter with independent functionalities. This method demonstrates the power of nested functions and closures in JavaScript. As you continue to explore JavaScript, keep playing with nested functions to unlock more potential in your coding projects.
Get creative and happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Calling a nested function JavaScript
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Nested Functions in JavaScript: Creating a Counter
As a budding JavaScript developer, you may often encounter the challenge of using nested functions effectively. One particular use case involves creating a counter with various functionalities. In this guide, we'll break down how to build such a counter and how to call the nested functions outside of its defining context. Let's get started!
The Problem: Creating a Functional Counter
Imagine you need a JavaScript counter that provides you with three essential functionalities:
counter() should return the next number incrementally starting from zero.
Here’s a simple implementation to illustrate this concept:
[[See Video to Reveal this Text or Code Snippet]]
Now the question arises, how do we call these nested functions from outside their context? The answer lies in returning the counter function from our makeCounter function. Let’s dive deeper into this solution.
The Solution: Returning the Counter Function
To gain access to the nested functions (i.e., decrease and set), follow these steps:
Step 1: Modify the makeCounter function
To expose the counter, you need to return it from makeCounter. Here’s how it should look:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Instantiate the Counter
Next, you’ll create a new instance of the counter using the makeCounter function:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Use the Counter Functions
Now that we have an instance of the counter, we can call its functionalities easily:
[[See Video to Reveal this Text or Code Snippet]]
Important Notes on Counter Behavior
When using count++, be aware that it will return the current value of count before incrementing it. If you want to increment and then return the updated value, consider using ++count instead.
Understanding the implications of nested functions can greatly enhance your JavaScript programming capabilities, especially when dealing with closures and encapsulation.
Conclusion
By returning the counter function from makeCounter, you can effectively manage a customizable counter with independent functionalities. This method demonstrates the power of nested functions and closures in JavaScript. As you continue to explore JavaScript, keep playing with nested functions to unlock more potential in your coding projects.
Get creative and happy coding!