filmov
tv
Mastering Python Regex to Match URLs Without File Extensions
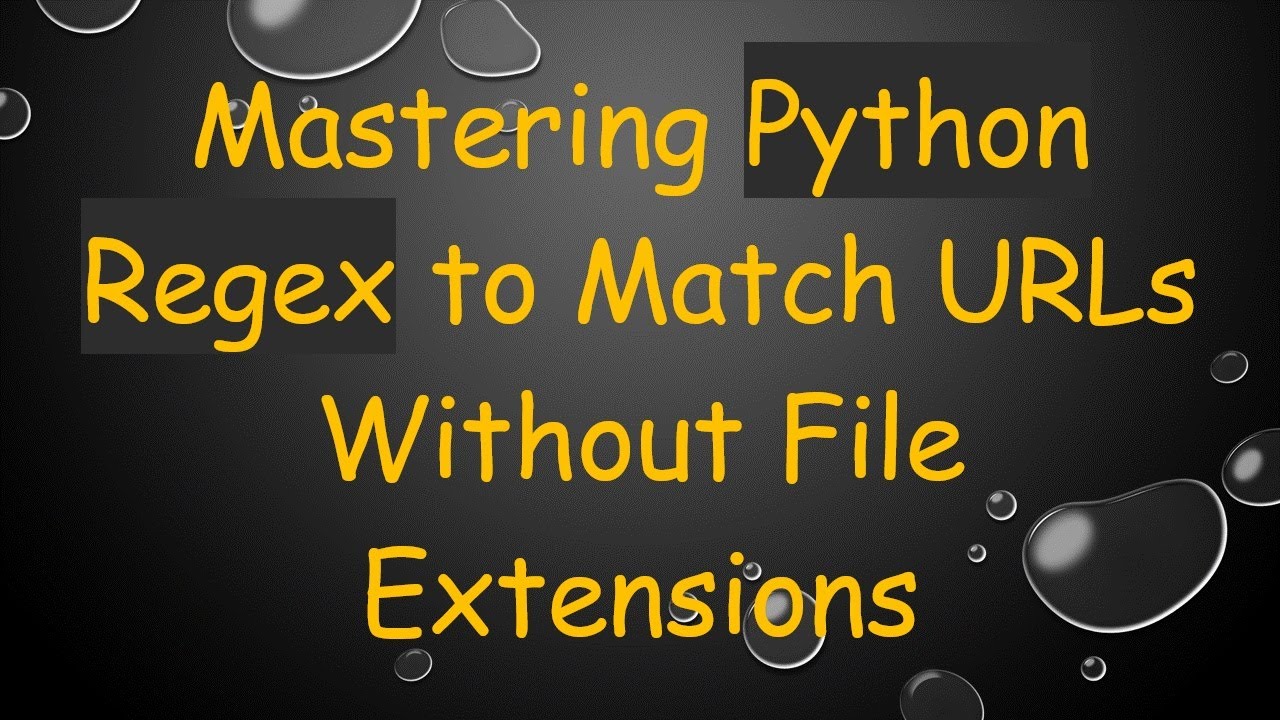
Показать описание
Learn how to create a Python regex that matches URLs without file extensions. This guide breaks down the solution into clear steps with practical examples.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python regex match url without file extension
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Python Regex to Match URLs Without File Extensions
Regular expressions (regex) are powerful tools in Python for searching and manipulating strings. One common use case is matching URLs, specifically those that do not end with file extensions. In this guide, we'll explore how to create a regex pattern that achieves this goal effectively.
The Problem: Matching URLs Without File Extensions
Imagine you need to filter out URLs that end with file extensions while still matching simple relative URLs. For instance, given these examples:
Understanding the Current Approach
The user initially tried the following regex pattern:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Refining the Regex Pattern
To effectively filter out unwanted file extensions while matching valid URLs, we need to modify the existing regex pattern. Here’s an improved version:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Regex Components
(/[a-zA-Z]+)*: This matches the leading slash followed by one or more alphabetical characters, allowing for multiple segments in the path.
/([a-zA-Z]+): This captures the last segment of the URL, ensuring it consists only of alphabetical characters.
(?!.[a-zA-Z]+): This is a negative lookahead assertion that checks if the URL does not end with a period followed by alphabetic characters, effectively preventing matches that would indicate a file extension.
(?:.)?: This part optionally matches a period but does not capture it. Adding it ensures that the regex can match URLs that end with a dot (like /foo.).
$: This anchors the pattern to the end of the string, ensuring that nothing follows the matched pattern.
Example Usage
Here’s how you would use the refined regex in Python:
[[See Video to Reveal this Text or Code Snippet]]
What to Expect
When you run the code above:
It will validate and return a match for /foo and /foo..
Conclusion
By utilizing the improved regex pattern, you can effectively match URLs without file extensions in Python. Regular expressions may seem daunting at first, but with practice and understanding, they can become an invaluable asset in your programming toolkit.
If you have further questions about regex or specific use cases, feel free to reach out in the comments below!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python regex match url without file extension
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering Python Regex to Match URLs Without File Extensions
Regular expressions (regex) are powerful tools in Python for searching and manipulating strings. One common use case is matching URLs, specifically those that do not end with file extensions. In this guide, we'll explore how to create a regex pattern that achieves this goal effectively.
The Problem: Matching URLs Without File Extensions
Imagine you need to filter out URLs that end with file extensions while still matching simple relative URLs. For instance, given these examples:
Understanding the Current Approach
The user initially tried the following regex pattern:
[[See Video to Reveal this Text or Code Snippet]]
The Solution: Refining the Regex Pattern
To effectively filter out unwanted file extensions while matching valid URLs, we need to modify the existing regex pattern. Here’s an improved version:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Regex Components
(/[a-zA-Z]+)*: This matches the leading slash followed by one or more alphabetical characters, allowing for multiple segments in the path.
/([a-zA-Z]+): This captures the last segment of the URL, ensuring it consists only of alphabetical characters.
(?!.[a-zA-Z]+): This is a negative lookahead assertion that checks if the URL does not end with a period followed by alphabetic characters, effectively preventing matches that would indicate a file extension.
(?:.)?: This part optionally matches a period but does not capture it. Adding it ensures that the regex can match URLs that end with a dot (like /foo.).
$: This anchors the pattern to the end of the string, ensuring that nothing follows the matched pattern.
Example Usage
Here’s how you would use the refined regex in Python:
[[See Video to Reveal this Text or Code Snippet]]
What to Expect
When you run the code above:
It will validate and return a match for /foo and /foo..
Conclusion
By utilizing the improved regex pattern, you can effectively match URLs without file extensions in Python. Regular expressions may seem daunting at first, but with practice and understanding, they can become an invaluable asset in your programming toolkit.
If you have further questions about regex or specific use cases, feel free to reach out in the comments below!