filmov
tv
Convert ASCII to Hex in Dart: A Guide for Flutter Developers
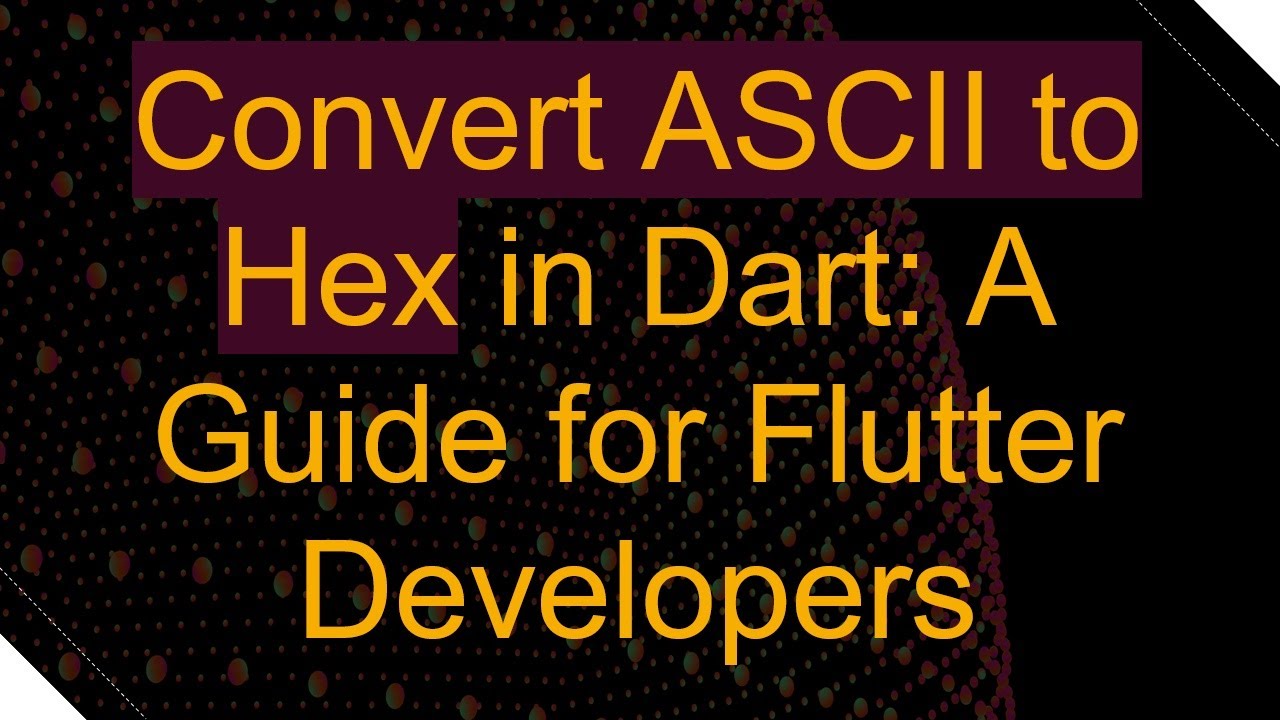
Показать описание
Summary: Learn how to convert ASCII to hex in Dart, especially for Flutter developers, by following this step-by-step guide for a critical task in string manipulation.
---
Convert ASCII to Hex in Dart: A Guide for Flutter Developers
In many programming projects, especially those that deal with communication protocols or low-level data processing, converting ASCII to hex can be a crucial task. If you're coming from a Java background and are now working with Dart in Flutter, this guide is for you. Here, we'll explore how to perform this conversion efficiently in Dart.
Understanding ASCII and Hex
Before diving into code, let’s briefly understand what we mean by ASCII and hex.
ASCII stands for American Standard Code for Information Interchange. It represents text in computers and other devices that use text.
Hex (or hexadecimal) is a base-16 number system used in mathematics and computing for a more human-friendly representation of binary-coded values.
Step-by-Step Guide to Convert ASCII to Hex in Dart
Define the Input String
First, you need an ASCII string that you want to convert into its hex representation.
[[See Video to Reveal this Text or Code Snippet]]
Convert ASCII to Hex
To accomplish the conversion, we will utilize Dart’s codeUnits property on the String class which gives us a list of UTF-16 code units for the string. Each of these units can then be converted to its hex representation.
[[See Video to Reveal this Text or Code Snippet]]
The toRadixString(16) converts each code unit to its hexadecimal string representation. The padLeft(2, '0') ensures each hex byte is two characters long, padding with zeros if necessary.
Test the Function
Finally, let's test our function with the example input string.
[[See Video to Reveal this Text or Code Snippet]]
When you run this code in your Flutter or Dart environment, you should see the corresponding hex representation of the input string.
Conclusion
The ability to convert ASCII to hex is a simple yet essential skill for developers working with text and data formats. By following the steps outlined in this guide, you should now be able to perform this conversion in Dart easily. This is particularly useful for Flutter developers who may need to handle various data encoding tasks.
Keep coding and happy developing!
---
Convert ASCII to Hex in Dart: A Guide for Flutter Developers
In many programming projects, especially those that deal with communication protocols or low-level data processing, converting ASCII to hex can be a crucial task. If you're coming from a Java background and are now working with Dart in Flutter, this guide is for you. Here, we'll explore how to perform this conversion efficiently in Dart.
Understanding ASCII and Hex
Before diving into code, let’s briefly understand what we mean by ASCII and hex.
ASCII stands for American Standard Code for Information Interchange. It represents text in computers and other devices that use text.
Hex (or hexadecimal) is a base-16 number system used in mathematics and computing for a more human-friendly representation of binary-coded values.
Step-by-Step Guide to Convert ASCII to Hex in Dart
Define the Input String
First, you need an ASCII string that you want to convert into its hex representation.
[[See Video to Reveal this Text or Code Snippet]]
Convert ASCII to Hex
To accomplish the conversion, we will utilize Dart’s codeUnits property on the String class which gives us a list of UTF-16 code units for the string. Each of these units can then be converted to its hex representation.
[[See Video to Reveal this Text or Code Snippet]]
The toRadixString(16) converts each code unit to its hexadecimal string representation. The padLeft(2, '0') ensures each hex byte is two characters long, padding with zeros if necessary.
Test the Function
Finally, let's test our function with the example input string.
[[See Video to Reveal this Text or Code Snippet]]
When you run this code in your Flutter or Dart environment, you should see the corresponding hex representation of the input string.
Conclusion
The ability to convert ASCII to hex is a simple yet essential skill for developers working with text and data formats. By following the steps outlined in this guide, you should now be able to perform this conversion in Dart easily. This is particularly useful for Flutter developers who may need to handle various data encoding tasks.
Keep coding and happy developing!