filmov
tv
Solving the Blazor Server Prerendering Challenge: Loading Data and UI Updates Made Easy
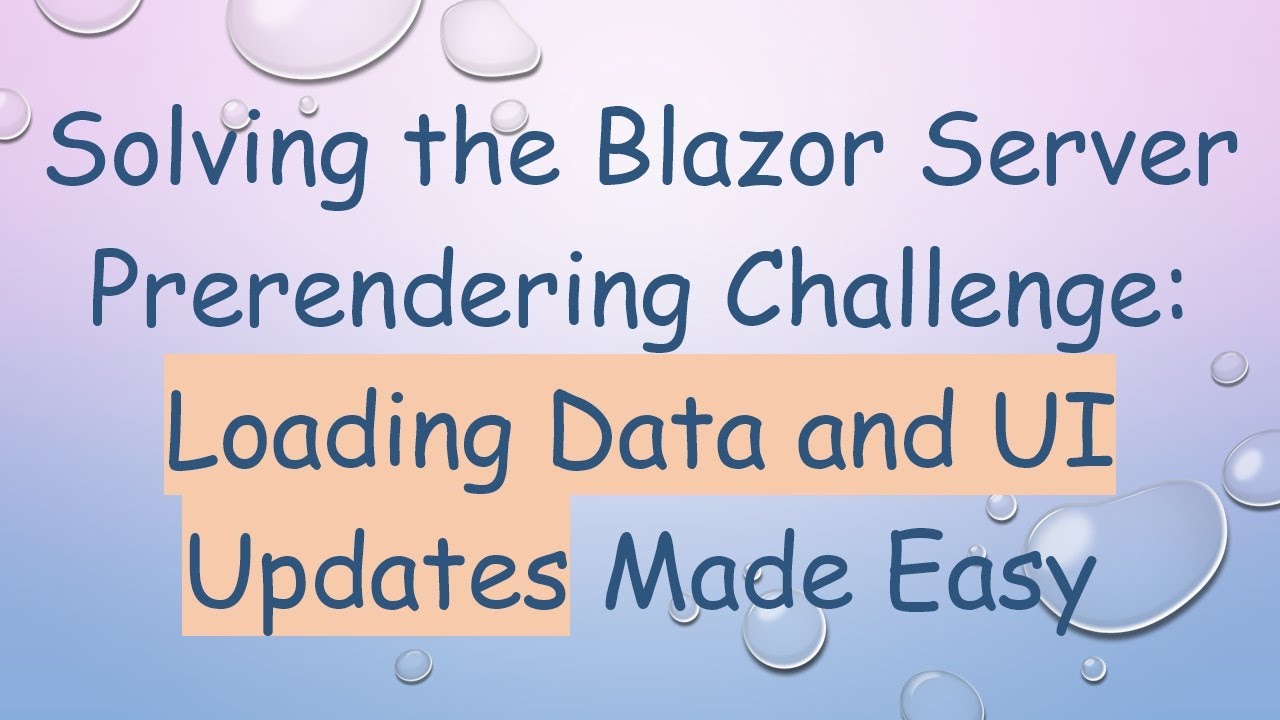
Показать описание
Discover how to load data from an API in Blazor Server while showing a loading animation and ensuring the UI updates correctly, without multiple API calls.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Blazor Server Prerendered: Where to load data and update the UI?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the Blazor Server Prerendering Challenge: Loading Data and UI Updates Made Easy
When developing applications with Blazor Server, one common challenge that developers face is efficiently loading data while managing user interactions, such as displaying loading animations. Today, we’ll discuss a specific problem related to data loading and user interface updates with Blazor Server prerendering, and how to effectively solve it.
The Challenge
You might have noticed that when you load data in the OnInitializedAsync() method, the loading animation appears correctly, but the API call is executed twice. Conversely, if you attempt to execute the API call within OnAfterRenderAsync(), it runs only once, but the UI does not update as expected.
The Code Example
Using OnInitializedAsync()
[[See Video to Reveal this Text or Code Snippet]]
Using OnAfterRenderAsync()
[[See Video to Reveal this Text or Code Snippet]]
The question then arises: How can you load the data only once, display the loading animation during the process, and ensure the UI updates appropriately?
The Solution
Fortunately, the solution is straightforward! The key is utilizing StateHasChanged() in the correct sequence to trigger the UI updates at each stage of data loading.
Updated OnAfterRenderAsync() Implementation
Here’s how you can adjust your code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code Changes
Flag Management: Before the API call, you set isLoading to true to indicate that loading is in progress.
Initial UI Update: The first call to StateHasChanged() updates the UI, showing the loading animation. This is crucial for providing feedback to the user.
Making the API Call: Wait for the API call to complete and populate your data.
Ending the Load: Once the data is retrieved, set isLoading to false to signal that loading has finished.
Final UI Update: The second call to StateHasChanged() updates the UI again to remove the loading animation and display the retrieved data.
Conclusion
By implementing these straightforward adjustments, you can successfully load data with Blazor Server while maintaining a responsive user interface that reflects loading states. This approach not only enhances user experience but also ensures that your application performs optimally by avoiding unnecessary API calls.
Now, you're equipped to tackle data loading and UI updates effectively in your Blazor Server applications! Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Blazor Server Prerendered: Where to load data and update the UI?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Solving the Blazor Server Prerendering Challenge: Loading Data and UI Updates Made Easy
When developing applications with Blazor Server, one common challenge that developers face is efficiently loading data while managing user interactions, such as displaying loading animations. Today, we’ll discuss a specific problem related to data loading and user interface updates with Blazor Server prerendering, and how to effectively solve it.
The Challenge
You might have noticed that when you load data in the OnInitializedAsync() method, the loading animation appears correctly, but the API call is executed twice. Conversely, if you attempt to execute the API call within OnAfterRenderAsync(), it runs only once, but the UI does not update as expected.
The Code Example
Using OnInitializedAsync()
[[See Video to Reveal this Text or Code Snippet]]
Using OnAfterRenderAsync()
[[See Video to Reveal this Text or Code Snippet]]
The question then arises: How can you load the data only once, display the loading animation during the process, and ensure the UI updates appropriately?
The Solution
Fortunately, the solution is straightforward! The key is utilizing StateHasChanged() in the correct sequence to trigger the UI updates at each stage of data loading.
Updated OnAfterRenderAsync() Implementation
Here’s how you can adjust your code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Code Changes
Flag Management: Before the API call, you set isLoading to true to indicate that loading is in progress.
Initial UI Update: The first call to StateHasChanged() updates the UI, showing the loading animation. This is crucial for providing feedback to the user.
Making the API Call: Wait for the API call to complete and populate your data.
Ending the Load: Once the data is retrieved, set isLoading to false to signal that loading has finished.
Final UI Update: The second call to StateHasChanged() updates the UI again to remove the loading animation and display the retrieved data.
Conclusion
By implementing these straightforward adjustments, you can successfully load data with Blazor Server while maintaining a responsive user interface that reflects loading states. This approach not only enhances user experience but also ensures that your application performs optimally by avoiding unnecessary API calls.
Now, you're equipped to tackle data loading and UI updates effectively in your Blazor Server applications! Happy coding!